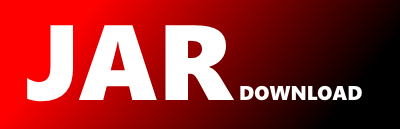
commonTest.io.data2viz.geojson.FeatureParsingTests.kt Maven / Gradle / Ivy
package io.data2viz.geojson
import kotlin.test.*
class FeatureParsingTests {
@Test
fun feature() {
//language=JSON
val json = """
{
"type": "Feature",
"geometry": {
"type": "LineString",
"coordinates": [
[102.0, 0.0],
[105.0, 1.0]
]
}
}
""".trimIndent()
val feature = json.toGeoJsonObject() as Feature
assertEquals(2, (feature.geometry as? LineString)?.coordinates?.size)
assertNull(feature.id)
}
@Test
fun featureWithStringId() {
//language=JSON
val json = """
{
"type": "Feature",
"id": "1234",
"geometry": {
"type": "LineString",
"coordinates": [
[0.0, 0.0],
[1.0, 1.0]
]
}
}
""".trimIndent()
val feature = json.toGeoJsonObject() as Feature
assertEquals("1234", feature.id)
assertTrue { feature.geometry is LineString }
}
@Test
fun featureWithIntId() {
//language=JSON
val json = """
{
"type": "Feature",
"id": 1234,
"geometry": {
"type": "LineString",
"coordinates": [
[0.0, 0.0],
[1.0, 1.0]
]
}
}
""".trimIndent()
val feature = json.toGeoJsonObject() as Feature
assertEquals(1234, feature.id)
assertTrue { feature.geometry is LineString }
}
@Test
fun featureWithProperties() {
//language=JSON
val json = """
{
"type": "Feature",
"geometry": { "type": "LineString", "coordinates": [ [102.0, 0.0], [105.0, 1.0] ] },
"properties": { "name" : "Archamps", "code" : 74016}
}
""".trimIndent()
val feature = json.toGeoJsonObject() as Feature
assertNotNull(feature.properties)
}
@Test
fun featuresWithProperties() {
//language=JSON
val json = """
{"type":"FeatureCollection","features":[
{
"type": "Feature",
"geometry": { "type": "LineString", "coordinates": [ [102.0, 0.0], [105.0, 1.0] ] },
"properties": { "name" : "Archamps", "code" : 74016}
},
{
"type": "Feature",
"geometry": { "type": "LineString", "coordinates": [ [102.0, 0.0], [105.0, 1.0] ] },
"properties": { "name" : "Collonges", "code" : 74017}
}
]}
""".trimIndent()
data class Commune(val name: String, val code: Int)
val features: List> = json.toFeaturesAndProperties {
Commune(stringProperty("name"), intProperty("code"))
}
assertEquals(2, features.size)
val archamps = features[0]
assertEquals("Archamps", archamps.second.name)
}
@Test
fun loadWorld110m() {
val json = """
{"type":"FeatureCollection", "features": [
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[-57.147436,5.97315],[-55.949318,5.772878],[-55.03325,6.025291],[-53.958045,5.756548],[-54.478633,4.896756],[-54.399542,4.212611],[-54.006931,3.620038],[-54.524754,2.311849],[-55.973322,2.510364],[-56.539386,1.899523],[-58.044694,4.060864],[-57.914289,4.812626],[-57.307246,5.073567],[-57.147436,5.97315]]]},"properties":{"name":"Suriname"},"id":"SUR"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[18.853144,49.49623],[19.825023,49.217125],[21.607808,49.470107],[22.558138,49.085738],[22.085608,48.422264],[20.801294,48.623854],[20.239054,48.327567],[17.857133,47.758429],[16.979667,48.123497],[16.960288,48.596982],[18.853144,49.49623]]]},"properties":{"name":"Slovakia"},"id":"SVK"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[13.806475,46.509306],[14.632472,46.431817],[16.202298,46.852386],[16.564808,46.503751],[15.768733,46.238108],[15.327675,45.452316],[13.71506,45.500324],[13.93763,45.591016],[13.806475,46.509306]]]},"properties":{"name":"Slovenia"},"id":"SVN"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[11.027369,58.856149],[12.300366,60.117933],[12.631147,61.293572],[11.992064,61.800362],[11.930569,63.128318],[12.579935,64.066219],[13.571916,64.049114],[13.55569,64.787028],[15.108411,66.193867],[16.768879,68.013937],[17.729182,68.010552],[17.993868,68.567391],[19.87856,68.407194],[20.645593,69.106247],[23.539473,67.936009],[23.56588,66.396051],[23.903379,66.006927],[22.183173,65.723741],[21.213517,65.026005],[21.369631,64.413588],[17.847779,62.7494],[17.119555,61.341166],[18.787722,60.081914],[17.869225,58.953766],[16.829185,58.719827],[16.44771,57.041118],[15.879786,56.104302],[14.666681,56.200885],[14.100721,55.407781],[12.942911,55.361737],[12.625101,56.30708],[11.787942,57.441817],[11.027369,58.856149]]]},"properties":{"name":"Sweden"},"id":"SWE"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[32.071665,-26.73382],[31.282773,-27.285879],[30.685962,-26.743845],[31.04408,-25.731452],[31.837778,-25.843332],[32.071665,-26.73382]]]},"properties":{"name":"Swaziland"},"id":"SWZ"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[38.792341,33.378686],[36.834062,32.312938],[35.719918,32.709192],[35.821101,33.277426],[36.61175,34.201789],[35.998403,34.644914],[36.149763,35.821535],[37.066761,36.623036],[38.167727,36.90121],[39.52258,36.716054],[40.673259,37.091276],[42.349591,37.229873],[41.289707,36.358815],[41.383965,35.628317],[41.006159,34.419372],[38.792341,33.378686]]]},"properties":{"name":"Syria"},"id":"SYR"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[14.495787,12.859396],[13.954477,13.353449],[13.540394,14.367134],[13.97217,15.68437],[15.247731,16.627306],[15.300441,17.92795],[15.903247,20.387619],[15.096888,21.308519],[14.8513,22.86295],[15.86085,23.40972],[23.83766,19.58047],[23.88689,15.61084],[23.02459,15.68072],[22.56795,14.94429],[22.03759,12.95546],[22.864165,11.142395],[21.723822,10.567056],[21.000868,9.475985],[20.059685,9.012706],[19.094008,9.074847],[17.96493,7.890914],[16.705988,7.508328],[15.27946,7.421925],[14.979996,8.796104],[13.954218,9.549495],[14.171466,10.021378],[15.467873,9.982337],[14.923565,10.891325],[14.89336,12.21905],[14.495787,12.859396]]]},"properties":{"name":"Chad"},"id":"TCD"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[1.865241,6.142158],[1.060122,5.928837],[0.490957,7.411744],[0.36758,10.191213],[0.023803,11.018682],[0.899563,10.997339],[0.772336,10.470808],[1.664478,9.12859],[1.618951,6.832038],[1.865241,6.142158]]]},"properties":{"name":"Togo"},"id":"TGO"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[102.584932,12.186595],[101.687158,12.64574],[100.83181,12.627085],[100.978467,13.412722],[100.097797,13.406856],[100.018733,12.307001],[99.153772,9.963061],[99.222399,9.239255],[99.873832,9.207862],[100.459274,7.429573],[102.141187,6.221636],[101.814282,5.810808],[100.085757,6.464489],[99.519642,7.343454],[98.503786,8.382305],[98.553551,9.93296],[99.587286,11.892763],[99.196354,12.804748],[99.097755,13.827503],[98.430819,14.622028],[98.903348,16.177824],[97.375896,18.445438],[97.797783,18.62708],[98.253724,19.708203],[98.959676,19.752981],[100.115988,20.41785],[100.606294,19.508344],[101.282015,19.462585],[101.059548,17.512497],[102.113592,18.109102],[102.998706,17.961695],[103.956477,18.240954],[104.716947,17.428859],[104.779321,16.441865],[105.589039,15.570316],[105.218777,14.273212],[104.281418,14.416743],[102.988422,14.225721],[102.348099,13.394247],[102.584932,12.186595]]]},"properties":{"name":"Thailand"},"id":"THA"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[71.014198,40.244366],[70.648019,39.935754],[69.55961,40.103211],[69.464887,39.526683],[70.549162,39.604198],[71.784694,39.279463],[73.675379,39.431237],[73.928852,38.505815],[74.864816,38.378846],[74.980002,37.41999],[73.260056,37.495257],[71.844638,36.738171],[71.448693,37.065645],[71.541918,37.905774],[70.806821,38.486282],[70.116578,37.588223],[69.518785,37.608997],[68.135562,37.023115],[67.83,37.144994],[68.392033,38.157025],[68.176025,38.901553],[69.329495,40.727824],[70.666622,40.960213],[71.014198,40.244366]]]},"properties":{"name":"Tajikistan"},"id":"TJK"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[61.210817,35.650072],[61.123071,36.491597],[60.377638,36.527383],[59.234762,37.412988],[57.330434,38.029229],[55.511578,37.964117],[54.800304,37.392421],[53.921598,37.198918],[53.880929,38.952093],[53.101028,39.290574],[52.693973,40.033629],[52.915251,40.876523],[53.858139,40.631034],[54.736845,40.951015],[53.721713,42.123191],[52.50246,41.783316],[54.079418,42.324109],[54.755345,42.043971],[55.455251,41.259859],[55.968191,41.308642],[58.629011,42.751551],[59.976422,42.223082],[60.083341,41.425146],[61.882714,41.084857],[62.37426,40.053886],[64.170223,38.892407],[66.54615,37.974685],[66.518607,37.362784],[64.746105,37.111818],[64.546479,36.312073],[63.193538,35.857166],[62.230651,35.270664],[61.210817,35.650072]]]},"properties":{"name":"Turkmenistan"},"id":"TKM"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[124.968682,-8.89279],[126.644704,-8.398247],[126.967992,-8.668256],[125.08852,-9.393173],[124.968682,-8.89279]]]},"properties":{"name":"East Timor"},"id":"TLS"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[-61.68,10.76],[-60.895,10.855],[-60.935,10.11],[-61.77,10],[-61.68,10.76]]]},"properties":{"name":"Trinidad and Tobago"},"id":"TTO"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[9.48214,30.307556],[9.055603,32.102692],[7.612642,33.344115],[7.524482,34.097376],[8.140981,34.655146],[8.376368,35.479876],[8.420964,36.946427],[9.509994,37.349994],[10.210002,37.230002],[10.939519,35.698984],[10.807847,34.833507],[10.149593,34.330773],[11.488787,33.136996],[11.432253,32.368903],[9.950225,31.37607],[9.970017,30.539325],[9.48214,30.307556]]]},"properties":{"name":"Tunisia"},"id":"TUN"},
{"type":"Feature","geometry":{"type":"MultiPolygon","coordinates":[[[[41.554084,41.535656],[42.619549,41.583173],[43.582746,41.092143],[43.656436,40.253564],[44.79399,39.713003],[44.109225,39.428136],[44.77267,37.17045],[42.779126,37.385264],[42.349591,37.229873],[40.673259,37.091276],[39.52258,36.716054],[38.167727,36.90121],[37.066761,36.623036],[36.149763,35.821535],[35.550936,36.565443],[34.714553,36.795532],[34.026895,36.21996],[32.509158,36.107564],[31.699595,36.644275],[30.621625,36.677865],[29.699976,36.144357],[28.732903,36.676831],[27.641187,36.658822],[27.048768,37.653361],[26.318218,38.208133],[26.8047,38.98576],[26.170785,39.463612],[27.28002,40.420014],[28.819978,40.460011],[29.240004,41.219991],[31.145934,41.087622],[32.347979,41.736264],[33.513283,42.01896],[35.167704,42.040225],[36.913127,41.335358],[38.347665,40.948586],[40.373433,41.013673],[41.554084,41.535656]]],[[[26.056942,40.824123],[26.117042,41.826905],[27.135739,42.141485],[27.99672,42.007359],[28.806438,41.054962],[27.619017,40.999823],[26.358009,40.151994],[26.056942,40.824123]]]]},"properties":{"name":"Turkey"},"id":"TUR"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[121.777818,24.394274],[120.74708,21.970571],[120.106189,23.556263],[120.69468,24.538451],[121.495044,25.295459],[121.777818,24.394274]]]},"properties":{"name":"Taiwan"},"id":"TWN"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[31.78597,52.10168],[33.7527,52.335075],[35.022183,51.207572],[35.356116,50.577197],[36.626168,50.225591],[37.39346,50.383953],[38.010631,49.915662],[40.06904,49.60105],[39.67465,48.78382],[39.738278,47.898937],[38.77057,47.82562],[38.223538,47.10219],[36.759855,46.6987],[35.823685,46.645964],[34.962342,46.273197],[35.239999,44.939996],[33.882511,44.361479],[33.546924,45.034771],[32.630804,45.519186],[33.298567,46.080598],[30.748749,46.5831],[29.603289,45.293308],[28.233554,45.488283],[28.933717,46.25883],[29.759972,46.349988],[29.122698,47.849095],[27.522537,48.467119],[26.619337,48.220726],[24.866317,47.737526],[23.142236,48.096341],[22.710531,47.882194],[22.085608,48.422264],[22.558138,49.085738],[22.51845,49.476774],[24.029986,50.705407],[23.527071,51.578454],[24.553106,51.888461],[26.337959,51.832289],[29.254938,51.368234],[30.555117,51.319503],[30.927549,52.042353],[31.78597,52.10168]]]},"properties":{"name":"Ukraine"},"id":"UKR"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[-57.625133,-30.216295],[-56.976026,-30.109686],[-53.787952,-32.047243],[-53.209589,-32.727666],[-53.373662,-33.768378],[-53.806426,-34.396815],[-54.935866,-34.952647],[-56.215297,-34.859836],[-57.139685,-34.430456],[-57.817861,-34.462547],[-58.427074,-33.909454],[-58.14244,-32.044504],[-57.625133,-30.216295]]]},"properties":{"name":"Uruguay"},"id":"URY"},
{"type":"Feature","geometry":{"type":"MultiPolygon","coordinates":[[[[-155.54211,19.08348],[-156.07347,19.70294],[-155.78505,20.2487],[-154.83147,19.45328],[-155.54211,19.08348]]],[[[-67.13741,45.13753],[-68.03252,44.3252],[-70.11617,43.68405],[-70.81489,42.8653],[-70.64,41.475],[-71.86,41.32],[-71.945,40.93],[-73.982,40.628],[-74.17838,39.70926],[-75.72205,37.93705],[-75.9718,36.89726],[-75.72749,35.55074],[-76.36318,34.80854],[-77.397635,34.51201],[-78.55435,33.86133],[-79.20357,33.15839],[-80.301325,32.509355],[-81.33629,31.44049],[-81.49042,30.72999],[-80.056539,26.88],[-80.38103,25.20616],[-81.17213,25.20126],[-81.71,25.87],[-82.85526,27.88624],[-82.65,28.55],[-83.70959,29.93656],[-85.10882,29.63615],[-86.4,30.4],[-89.18049,30.31598],[-89.40823,29.15961],[-90.880225,29.148535],[-91.626785,29.677],[-93.22637,29.78375],[-94.69,29.48],[-95.60026,28.73863],[-96.59404,28.30748],[-97.37,27.38],[-97.140008,25.869997],[-99.02,26.37],[-99.52,27.54],[-100.9576,29.38071],[-101.6624,29.7793],[-102.48,29.76],[-103.11,28.97],[-104.45697,29.57196],[-105.03737,30.64402],[-106.50759,31.75452],[-108.24,31.754854],[-108.24194,31.34222],[-111.02361,31.33472],[-114.815,32.52528],[-117.12776,32.53534],[-117.295938,33.046225],[-118.519895,34.027782],[-120.36778,34.44711],[-120.74433,35.15686],[-121.71457,36.16153],[-122.54747,37.55176],[-123.7272,38.95166],[-123.86517,39.76699],[-124.39807,40.3132],[-124.2137,41.99964],[-124.53284,42.76599],[-124.14214,43.70838],[-123.89893,45.52341],[-124.079635,46.86475],[-124.566101,48.379715],[-123.12,48.04],[-122.84,49],[-95.15907,49],[-94.32914,48.67074],[-92.61,48.45],[-91.64,48.14],[-90.83,48.27],[-89.6,48.01],[-88.378114,48.302918],[-84.87608,46.900083],[-83.592851,45.816894],[-82.550925,45.347517],[-82.137642,43.571088],[-83.12,42.08],[-82.439278,41.675105],[-81.277747,42.209026],[-80.247448,42.3662],[-78.939362,42.863611],[-78.72028,43.625089],[-76.820034,43.628784],[-76.5,44.018459],[-74.867,45.00048],[-71.50506,45.0082],[-70.66,45.46],[-69.99997,46.69307],[-69.237216,47.447781],[-67.79046,47.06636],[-67.79134,45.70281],[-67.13741,45.13753]]],[[[-153.006314,57.115842],[-154.00509,56.734677],[-154.670993,57.461196],[-153.228729,57.968968],[-153.006314,57.115842]]],[[[-140.986,69.712],[-140.99778,60.30639],[-139.039,60.000007],[-137.4525,58.905],[-135.47583,59.78778],[-134.945,59.27056],[-133.35556,58.41028],[-131.70781,56.55212],[-130.00778,55.91583],[-129.98,55.285],[-130.53611,54.80278],[-131.967211,55.497776],[-132.250011,56.369996],[-133.539181,57.178887],[-134.078063,58.123068],[-136.628062,58.212209],[-137.800006,58.499995],[-139.867787,59.537762],[-142.574444,60.084447],[-143.958881,59.99918],[-147.114374,60.884656],[-148.224306,60.672989],[-148.018066,59.978329],[-149.727858,59.705658],[-151.716393,59.155821],[-151.895839,60.727198],[-152.57833,60.061657],[-154.019172,59.350279],[-153.287511,58.864728],[-154.232492,58.146374],[-156.308335,57.422774],[-156.556097,56.979985],[-158.117217,56.463608],[-158.433321,55.994154],[-159.603327,55.566686],[-160.28972,55.643581],[-163.069447,54.689737],[-162.870001,55.348043],[-161.804175,55.894986],[-160.563605,56.008055],[-160.07056,56.418055],[-157.72277,57.570001],[-157.550274,58.328326],[-158.517218,58.787781],[-159.058606,58.424186],[-160.355271,59.071123],[-161.968894,58.671665],[-161.874171,59.633621],[-162.518059,59.989724],[-163.818341,59.798056],[-165.346388,60.507496],[-165.350832,61.073895],[-166.121379,61.500019],[-164.562508,63.146378],[-163.067224,63.059459],[-162.260555,63.541936],[-160.772507,63.766108],[-161.391926,64.777235],[-162.757786,64.338605],[-163.546394,64.55916],[-164.96083,64.446945],[-166.425288,64.686672],[-168.11056,65.669997],[-164.47471,66.57666],[-163.788602,66.077207],[-161.677774,66.11612],[-162.489715,66.735565],[-163.719717,67.116395],[-165.390287,68.042772],[-166.764441,68.358877],[-166.204707,68.883031],[-164.430811,68.915535],[-163.168614,69.371115],[-162.930566,69.858062],[-161.908897,70.33333],[-159.039176,70.891642],[-158.119723,70.824721],[-156.580825,71.357764],[-153.900006,70.889989],[-152.210006,70.829992],[-150.739992,70.430017],[-149.720003,70.53001],[-147.613362,70.214035],[-144.920011,69.989992],[-143.589446,70.152514],[-140.986,69.712]]]]},"properties":{"name":"USA"},"id":"USA"},
{"type":"Feature","geometry":{"type":"Polygon","coordinates":[[[31.191409,-22.25151],[29.432188,-22.091313],[28.02137,-21.485975],[27.724747,-20.499059],[27.296505,-20.39152],[26.164791,-19.293086],[25.264226,-17.73654],[27.044427,-17.938026],[27.598243,-17.290831],[29.516834,-15.644678],[30.274256,-15.507787],[32.847639,-16.713398],[32.772708,-19.715592],[32.244988,-21.116489],[31.191409,-22.25151]]]},"properties":{"name":"Zimbabwe"},"id":"ZWE"}
]}
""".trimIndent()
val features: List> = json.toFeaturesAndProperties { stringProperty("name") }
val country = features.first { it.first.id == "ZWE" }
assertEquals("Zimbabwe", country.second)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy