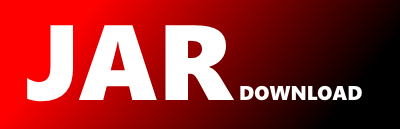
io.data2viz.voronoi.Cell.kt Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2018-2019. data2viz sàrl.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
*/
package io.data2viz.voronoi
import kotlin.math.atan2
internal var wCells: Array? = null
class Cell(val site: Site) {
/**
* List of index of edges of the current Cell.
*/
val halfedges = mutableListOf()
companion object {
fun createCell(site: Site) {
wCells!![site.index] = Cell(site)
}
}
fun halfedgeAngle(edge: Edge): Double {
var va = edge.left.pos
var vb = edge.right?.pos
if (site.pos === vb) {
vb = va
va = site.pos
}
if (vb != null) {
return atan2(vb.y - va.y, vb.x - va.x)
}
if (site.pos === va) {
va = edge.end!!
vb = edge.start
} else {
va = edge.start!!
vb = edge.end
}
return atan2(va.x - vb!!.x, vb.y - va.y)
}
fun halfedgeStart(edge: Edge) = if (edge.left !== site) edge.end else edge.start
fun halfedgeEnd(edge: Edge) = if (edge.left === site) edge.end else edge.start
fun cellHalfedgeStart(edge:Edge) = if (edge.left !== site) edge.end else edge.start
fun cellHalfedgeEnd (edge:Edge) = if (edge.left === site) edge.end else edge.start
}
/**
* Sort all cells halfedges, necessary for polygons.
*/
fun sortCellHalfedges() {
var edgeCount:Int
var halfedges: MutableList
var cell: Cell
for (cellIndex in 0..wCells!!.lastIndex) {
cell = wCells!![cellIndex]!!
halfedges = cell.halfedges
edgeCount = halfedges.size
val indexes: Array = Array(edgeCount) { it }
val angles: Array = Array(edgeCount) { cell.halfedgeAngle(wEdges[halfedges[it]]!!)}
indexes.sortWith(object : Comparator { override fun compare(a: Int, b: Int) = angles[b].compareTo(angles[a]) })
val temp: Array = Array(edgeCount) {halfedges[indexes[it]]}
(0..edgeCount-1).forEach { halfedges[it] = temp[it] }
}
}
|
© 2015 - 2025 Weber Informatics LLC | Privacy Policy