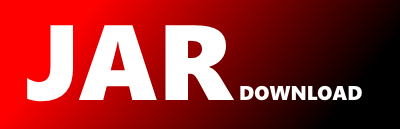
io.datakernel.config.ConfigConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datakernel-boot Show documentation
Show all versions of datakernel-boot Show documentation
An intelligent way of booting complex applications and services according to their dependencies
/*
* Copyright (C) 2015 SoftIndex LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.datakernel.config;
import io.datakernel.annotation.Nullable;
import java.util.function.Function;
import java.util.function.Predicate;
import static io.datakernel.util.Preconditions.checkArgument;
public interface ConfigConverter {
T get(Config config, @Nullable T defaultValue);
T get(Config config);
/**
* Applies given converter function to the converted value
*
* @param to converter from T to V
* @param from converter from V to T
* @param return type
* @return converter that knows how to get V value from T value saved in config
*/
default ConfigConverter transform(Function to, Function from) {
ConfigConverter thisConverter = this;
return new ConfigConverter() {
@Override
@Nullable
public V get(Config config, @Nullable V defaultValue) {
T value = thisConverter.get(config, defaultValue == null ? null : from.apply(defaultValue));
return value != null ? to.apply(value) : null;
}
@Override
@Nullable
public V get(Config config) {
return to.apply(thisConverter.get(config));
}
};
}
default ConfigConverter withConstraint(Predicate predicate) {
ConfigConverter thisConverter = this;
return new ConfigConverter() {
@Override
public T get(Config config, T defaultValue) {
T value = thisConverter.get(config, defaultValue);
checkArgument(predicate.test(value));
return value;
}
@Override
public T get(Config config) {
T t = thisConverter.get(config);
checkArgument(predicate.test(t));
return t;
}
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy