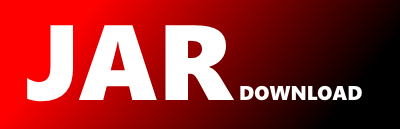
io.datakernel.util.guice.InitializerModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datakernel-boot Show documentation
Show all versions of datakernel-boot Show documentation
An intelligent way of booting complex applications and services according to their dependencies
package io.datakernel.util.guice;
import com.google.inject.*;
import com.google.inject.matcher.AbstractMatcher;
import com.google.inject.spi.ProvisionListener;
import io.datakernel.util.Initializable;
import io.datakernel.util.Initializer;
import io.datakernel.util.SimpleType;
import java.lang.annotation.Annotation;
import java.lang.reflect.Type;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
/**
* This module adds some Guice magic to your applications.
*
* It listens for any elements that extend {@link Initializable} interface.
* For each of those, it searches for the appropriate {@link Initializer} element.
* If initializer is found, it applies it to the initializable element.
*
*/
public final class InitializerModule extends AbstractModule {
private InitializerModule() {
}
public static InitializerModule create() {
return new InitializerModule();
}
@Override
protected void configure() {
Provider injectorProvider = getProvider(Injector.class);
Map, Initializer> initializerCache = new ConcurrentHashMap<>();
bindListener(new AbstractMatcher>() {
@Override
public boolean matches(Binding> binding) {
return Initializable.class.isAssignableFrom(binding.getKey().getTypeLiteral().getRawType());
}
}, new ProvisionListener() {
@SuppressWarnings("unchecked")
@Override
public void onProvision(ProvisionInvocation provision) {
T object = provision.provision();
if (object == null) {
return;
}
initializerCache
.computeIfAbsent(provision.getBinding().getKey(), key -> {
Type type = key.getTypeLiteral().getType();
Type initializerType = SimpleType.of(OptionalInitializer.class, SimpleType.ofType(type)).getType();
Annotation annotation = key.getAnnotation();
Binding> binding = annotation != null ?
injectorProvider.get().getBinding(Key.get(initializerType, annotation)) :
injectorProvider.get().getBinding(Key.get(initializerType));
return ((OptionalInitializer) binding.getProvider().get());
})
.accept((Initializable) object);
}
});
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy