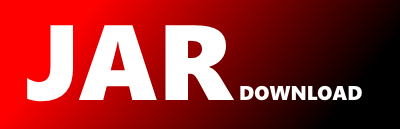
io.datakernel.worker.WorkerPools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of datakernel-boot Show documentation
Show all versions of datakernel-boot Show documentation
An intelligent way of booting complex applications and services according to their dependencies
/*
* Copyright (C) 2015 SoftIndex LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.datakernel.worker;
import com.google.inject.Key;
import com.google.inject.Provider;
import com.google.inject.TypeLiteral;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.CopyOnWriteArrayList;
import java.util.function.Function;
import java.util.stream.Collectors;
import static io.datakernel.util.Preconditions.checkArgument;
import static java.util.stream.Collectors.toList;
@SuppressWarnings("unchecked")
public final class WorkerPools {
private final List workerPools = new CopyOnWriteArrayList<>();
private final ThreadLocal threadLocalWorkerPool = ThreadLocal.withInitial(() ->
workerPools.stream()
// if local worker id exists than this thread is associated with a worker from this pool
.filter(pool -> pool.getLocalWorkerId() != null)
.findAny()
.orElseThrow(() -> new IllegalStateException("No WorkerPool is associated with current thread")));
private final Map, ThreadLocal>> threadLocalCache = new ConcurrentHashMap<>();
void addWorkerPool(WorkerPool workerPool) {
checkArgument(!workerPools.contains(workerPool), "WorkerPool has already been added");
workerPools.add(workerPool);
}
public List getWorkerPools() {
return Collections.unmodifiableList(workerPools);
}
public Map> getWorkerPoolObjects(Key key) {
return workerPools.stream().collect(Collectors.toMap(Function.identity(), workerPool -> workerPool.getInstances(key)));
}
public List getAllObjects(Key key) {
return (List) workerPools.stream().map(workerPool -> workerPool.pool.get(key)).filter(Objects::nonNull).flatMap(List::stream).collect(toList());
}
public WorkerPool getCurrentWorkerPool() {
return threadLocalWorkerPool.get();
}
@SuppressWarnings("unchecked")
public T getCurrentInstance(Key key) {
return getCurrentWorkerPool().getCurrentInstance(key);
}
// region getCurrentInstance overloads
public T getCurrentInstance(Class type) {
return getCurrentInstance(Key.get(type));
}
public T getCurrentInstance(TypeLiteral type) {
return getCurrentInstance(Key.get(type));
}
// endregion
public Provider getCurrentInstanceProvider(Key key) {
checkArgument(isValidBinding(key), "Cannot get provider for key: %s", key);
ThreadLocal threadLocal = (ThreadLocal) threadLocalCache.computeIfAbsent(key, $ ->
ThreadLocal.withInitial(() -> getCurrentInstance(key)));
return threadLocal::get;
}
// region getCurrentInstanceProvider overloads
public Provider getCurrentInstanceProvider(Class type) {
return getCurrentInstanceProvider(Key.get(type));
}
public Provider getCurrentInstanceProvider(TypeLiteral type) {
return getCurrentInstanceProvider(Key.get(type));
}
// endregion
@SuppressWarnings("unchecked")
public List getInstances(Key key) {
return getCurrentWorkerPool().getInstances(key);
}
// region getInstances overloads
public List getInstances(Class type) {
return getInstances(Key.get(type));
}
public List getInstances(TypeLiteral type) {
return getInstances(Key.get(type));
}
// endregion
private boolean isValidBinding(Key> key) {
return workerPools.stream()
.anyMatch(workerPool -> workerPool.isValidBinding(key));
}
public int size(){
return workerPools.size();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy