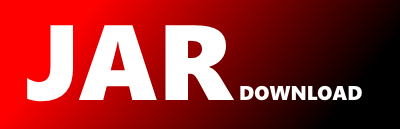
io.datakernel.http.decoder.Decoder Maven / Gradle / Ivy
package io.datakernel.http.decoder;
import io.datakernel.functional.Either;
import io.datakernel.http.HttpRequest;
import io.datakernel.util.*;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.List;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* A high-level API that allows declarative definition of HTTP parsers
* that can convert incoming requests to concrete objects.
* This allows complex parsers to be algebraically built from simple ones.
*/
public interface Decoder {
/**
* Either return the parsed type or format
*/
Either decode(@NotNull HttpRequest request);
@Nullable
default T decodeOrNull(@NotNull HttpRequest request) {
return decode(request).getLeftOrNull();
}
default T decodeOrThrow(@NotNull HttpRequest request) throws DecodeException {
Either either = decode(request);
if (either.isLeft()) return either.getLeft();
throw new DecodeException(either.getRight());
}
/**
* An id that is going to be used in the error-tree if at some point the whole parser fails
*/
String getId();
default Decoder withId(String id) {
return new Decoder() {
@Override
public Either decode(@NotNull HttpRequest request) {
return Decoder.this.decode(request);
}
@Override
public String getId() {
return id;
}
};
}
default Decoder map(Function fn) {
return mapEx(Mapper.of(fn));
}
default Decoder map(Function fn, String message) {
return mapEx(Mapper.of(fn, message));
}
/**
* Enhanced functional 'map' operation.
* If mapped returns an errors, then the returned decoder fails with that error.
*/
default Decoder mapEx(Mapper fn) {
return new AbstractDecoder(getId()) {
@Override
public Either decode(@NotNull HttpRequest request) {
return Decoder.this.decode(request)
.flatMapLeft(value ->
fn.map(value)
.mapRight(DecodeErrors::of));
}
};
}
default Decoder validate(Predicate predicate, String error) {
return validate(Validator.of(predicate, error));
}
/**
* Enhanced functional 'filter' operation.
* If validator returns non-empty list of errors,
* then the returned decoder fails with these errors.
*/
default Decoder validate(Validator validator) {
return new AbstractDecoder(getId()) {
@Override
public Either decode(@NotNull HttpRequest request) {
Either decodedValue = Decoder.this.decode(request);
if (decodedValue.isRight()) return decodedValue;
List errors = validator.validate(decodedValue.getLeft());
if (errors.isEmpty()) return decodedValue;
return Either.right(DecodeErrors.of(errors));
}
};
}
@NotNull
static Decoder create(Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy