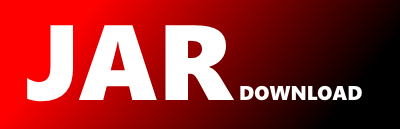
io.datakernel.http.decoder.Decoders Maven / Gradle / Ivy
package io.datakernel.http.decoder;
import io.datakernel.functional.Either;
import io.datakernel.http.HttpRequest;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.util.function.BiFunction;
import java.util.function.Function;
/**
* This class contains some common primitive {@link Decoder HttpDecoders}, that
* can be combined to form complex ones.
*/
@SuppressWarnings("RedundantCast")
public final class Decoders {
public static final String REQUIRED_GET_PARAM = "Required GET param: %1";
public static final String REQUIRED_POST_PARAM = "Required POST param: %1";
public static final String REQUIRED_PATH_PARAM = "Required path param";
public static final String REQUIRED_COOKIE = "Required cookie: %1";
private static Decoder ofParamEx(String paramName,
@NotNull Mapper fn,
@NotNull BiFunction paramSupplier,
String message) {
return new AbstractDecoder(paramName) {
@Override
public Either decode(@NotNull HttpRequest request) {
String str = paramSupplier.apply(request, paramName);
return str != null ?
fn.map(str)
.mapRight(DecodeErrors::of) :
Either.right(DecodeErrors.of(message, paramName));
}
};
}
private static Decoder ofParamEx(String paramName,
@NotNull Mapper fn,
@NotNull BiFunction paramSupplier,
@Nullable T defaultValue) {
return new AbstractDecoder(paramName) {
@Override
public Either decode(@NotNull HttpRequest request) {
String str = paramSupplier.apply(request, paramName);
return str != null ?
fn.map(str)
.mapRight(DecodeErrors::of) :
Either.left(defaultValue);
}
};
}
public static Decoder ofGet(String paramName) {
return ofGetEx(paramName, (Mapper)Either::left);
}
public static Decoder ofGet(String paramName, String defaultValue) {
return ofGetEx(paramName, (Mapper) Either::left, defaultValue);
}
public static Decoder ofGet(String paramName, Function fn, String message) {
return ofGetEx(paramName, Mapper.of(fn, message));
}
public static Decoder ofGet(String paramName, Function fn, T defaultValue) {
return ofGetEx(paramName, Mapper.of(fn), defaultValue);
}
public static Decoder ofGetEx(@NotNull String paramName,
@NotNull Mapper fn) {
return ofParamEx(paramName, fn, HttpRequest::getQueryParameter, REQUIRED_GET_PARAM);
}
public static Decoder ofGetEx(@NotNull String paramName,
@NotNull Mapper fn,
@Nullable T defaultValue) {
return ofParamEx(paramName, fn, HttpRequest::getQueryParameter, defaultValue);
}
public static Decoder ofPost(String paramName) {
return ofPostEx(paramName, (Mapper) Either::left);
}
public static Decoder ofPost(String paramName, String defaultValue) {
return ofPostEx(paramName, (Mapper) Either::left, defaultValue);
}
public static Decoder ofPost(String paramName, Function fn, String message) {
return ofPostEx(paramName, Mapper.of(fn, message));
}
public static Decoder ofPost(String paramName, Function fn, T defaultValue) {
return ofPostEx(paramName, Mapper.of(fn), defaultValue);
}
public static Decoder ofPostEx(@NotNull String paramName,
@NotNull Mapper fn) {
return ofParamEx(paramName, fn, HttpRequest::getPostParameter, REQUIRED_POST_PARAM);
}
public static Decoder ofPostEx(@NotNull String paramName,
@NotNull Mapper fn,
@Nullable T defaultValue) {
return ofParamEx(paramName, fn, HttpRequest::getPostParameter, defaultValue);
}
public static Decoder ofPath(String paramName) {
return ofPathEx(paramName, (Mapper) Either::left);
}
public static Decoder ofPath(String paramName, String defaultValue) {
return ofPathEx(paramName, (Mapper) Either::left, defaultValue);
}
public static Decoder ofPath(String paramName, Function fn, String message) {
return ofPathEx(paramName, Mapper.of(fn, message));
}
public static Decoder ofPath(String paramName, Function fn, T defaultValue) {
return ofPathEx(paramName, Mapper.of(fn), defaultValue);
}
public static Decoder ofPathEx(@NotNull String paramName,
@NotNull Mapper fn) {
return ofParamEx(paramName, fn, HttpRequest::getPathParameter, REQUIRED_PATH_PARAM);
}
public static Decoder ofPathEx(@NotNull String paramName,
@NotNull Mapper fn,
@Nullable T defaultValue) {
return ofParamEx(paramName, fn, HttpRequest::getPathParameter, defaultValue);
}
public static Decoder ofCookie(String paramName) {
return ofCookieEx(paramName, (Mapper) Either::left);
}
public static Decoder ofCookie(String paramName, String defaultValue) {
return ofCookieEx(paramName, (Mapper) Either::left, defaultValue);
}
public static Decoder ofCookie(String paramName, Function fn, String message) {
return ofCookieEx(paramName, Mapper.of(fn, message));
}
public static Decoder ofCookie(String paramName, Function fn, T defaultValue) {
return ofCookieEx(paramName, Mapper.of(fn), defaultValue);
}
public static Decoder ofCookieEx(@NotNull String paramName,
@NotNull Mapper fn) {
return ofParamEx(paramName, fn, HttpRequest::getCookie, REQUIRED_COOKIE);
}
public static Decoder ofCookieEx(@NotNull String paramName,
@NotNull Mapper fn,
@Nullable T defaultValue) {
return ofParamEx(paramName, fn, HttpRequest::getCookie, defaultValue);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy