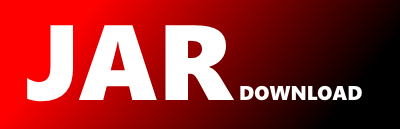
io.datarouter.gcp.spanner.SpannerClientNodeFactory Maven / Gradle / Ivy
/*
* Copyright © 2009 HotPads ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.datarouter.gcp.spanner;
import java.util.List;
import java.util.function.UnaryOperator;
import javax.inject.Inject;
import javax.inject.Singleton;
import io.datarouter.gcp.spanner.field.SpannerFieldCodecRegistry;
import io.datarouter.gcp.spanner.node.SpannerNode;
import io.datarouter.gcp.spanner.node.entity.SpannerSubEntityNode;
import io.datarouter.model.databean.Databean;
import io.datarouter.model.entity.Entity;
import io.datarouter.model.key.entity.EntityKey;
import io.datarouter.model.key.primary.EntityPrimaryKey;
import io.datarouter.model.serialize.fielder.DatabeanFielder;
import io.datarouter.opencensus.adapter.physical.PhysicalIndexedSortedMapStorageOpencensusAdapter;
import io.datarouter.opencensus.adapter.physical.PhysicalSubEntitySortedMapStorageOpencensusAdapter;
import io.datarouter.storage.client.imp.BaseClientNodeFactory;
import io.datarouter.storage.client.imp.WrappedNodeFactory;
import io.datarouter.storage.client.imp.WrappedSubEntityNodeFactory;
import io.datarouter.storage.node.NodeParams;
import io.datarouter.storage.node.adapter.availability.PhysicalIndexedSortedMapStorageAvailabilityAdapterFactory;
import io.datarouter.storage.node.adapter.availability.PhysicalSubEntitySortedMapStorageAvailabilityAdapterFactory;
import io.datarouter.storage.node.adapter.callsite.physical.PhysicalIndexedSortedMapStorageCallsiteAdapter;
import io.datarouter.storage.node.adapter.callsite.physical.PhysicalSubEntitySortedMapStorageCallsiteAdapter;
import io.datarouter.storage.node.adapter.counter.physical.PhysicalIndexedSortedMapStorageCounterAdapter;
import io.datarouter.storage.node.adapter.counter.physical.PhysicalSubEntitySortedMapStorageCounterAdapter;
import io.datarouter.storage.node.adapter.sanitization.physical.PhysicalIndexedSortedMapStorageSanitizationAdapter;
import io.datarouter.storage.node.adapter.sanitization.physical.PhysicalSubEntitySortedMapStorageSanitizationAdapter;
import io.datarouter.storage.node.adapter.trace.physical.PhysicalIndexedSortedMapStorageTraceAdapter;
import io.datarouter.storage.node.adapter.trace.physical.PhysicalSubEntitySortedMapStorageTraceAdapter;
import io.datarouter.storage.node.entity.EntityNodeParams;
import io.datarouter.storage.node.entity.PhysicalSubEntitySortedMapStorageNode;
import io.datarouter.storage.node.op.combo.IndexedSortedMapStorage.PhysicalIndexedSortedMapStorageNode;
import io.datarouter.storage.node.type.index.ManagedNodesHolder;
@Singleton
public class SpannerClientNodeFactory extends BaseClientNodeFactory{
@Inject
private SpannerClientType spannerClientType;
@Inject
private ManagedNodesHolder managedNodesHolder;
@Inject
private SpannerClientManager spannerClientManager;
@Inject
private SpannerFieldCodecRegistry spannerFieldCodecRegistry;
@Inject
private PhysicalIndexedSortedMapStorageAvailabilityAdapterFactory
physicalIndexedSortedMapStorageAvailabilityAdapterFactory;
@Inject
private PhysicalSubEntitySortedMapStorageAvailabilityAdapterFactory
physicalSubEntitySortedMapStorageAvailabilityAdapterFactory;
public class SpannerWrappedNodeFactory<
EK extends EntityKey,
E extends Entity,
PK extends EntityPrimaryKey,
D extends Databean,
F extends DatabeanFielder>
extends WrappedNodeFactory>{
@Override
protected PhysicalIndexedSortedMapStorageNode createNode(
EntityNodeParams entityNodeParams,
NodeParams nodeParams){
return new SpannerNode<>(
nodeParams,
spannerClientType,
managedNodesHolder,
spannerClientManager,
spannerFieldCodecRegistry);
}
@Override
protected List>> getAdapters(){
return List.of(
PhysicalIndexedSortedMapStorageSanitizationAdapter::new,
PhysicalIndexedSortedMapStorageCounterAdapter::new,
PhysicalIndexedSortedMapStorageOpencensusAdapter::new,
PhysicalIndexedSortedMapStorageTraceAdapter::new,
physicalIndexedSortedMapStorageAvailabilityAdapterFactory::create,
PhysicalIndexedSortedMapStorageCallsiteAdapter::new);
}
}
public class SpannerWrappedSubEntityNodeFactory<
EK extends EntityKey,
E extends Entity,
PK extends EntityPrimaryKey,
D extends Databean,
F extends DatabeanFielder>
extends WrappedSubEntityNodeFactory>{
@Override
protected PhysicalSubEntitySortedMapStorageNode createSubEntityNode(
EntityNodeParams entityNodeParams,
NodeParams nodeParams){
return new SpannerSubEntityNode<>(nodeParams,
spannerClientType,
entityNodeParams,
managedNodesHolder,
spannerClientManager,
spannerFieldCodecRegistry);
}
@Override
protected List>> getAdapters(){
return List.of(
PhysicalSubEntitySortedMapStorageSanitizationAdapter::new,
PhysicalSubEntitySortedMapStorageCounterAdapter::new,
PhysicalSubEntitySortedMapStorageOpencensusAdapter::new,
PhysicalSubEntitySortedMapStorageTraceAdapter::new,
physicalSubEntitySortedMapStorageAvailabilityAdapterFactory::create,
PhysicalSubEntitySortedMapStorageCallsiteAdapter::new);
}
}
@Override
protected <
EK extends EntityKey,
E extends Entity,
PK extends EntityPrimaryKey,
D extends Databean,
F extends DatabeanFielder>
WrappedNodeFactory makeWrappedNodeFactory(){
return new SpannerWrappedNodeFactory<>();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy