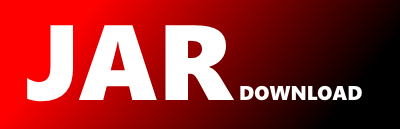
io.datarouter.tasktracker.service.LongRunningTaskService Maven / Gradle / Ivy
The newest version!
/*
* Copyright © 2009 HotPads ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.datarouter.tasktracker.service;
import java.time.Instant;
import java.util.Comparator;
import java.util.HashMap;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.SortedSet;
import java.util.TreeSet;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import io.datarouter.instrumentation.task.TaskTracker;
import io.datarouter.tasktracker.scheduler.LongRunningTaskStatus;
import io.datarouter.tasktracker.storage.LongRunningTask;
import io.datarouter.tasktracker.storage.LongRunningTaskDao;
import io.datarouter.tasktracker.storage.LongRunningTaskKey;
import io.datarouter.types.MilliTime;
import io.datarouter.util.tuple.Range;
import jakarta.inject.Inject;
import jakarta.inject.Singleton;
@Singleton
public class LongRunningTaskService{
private static final Logger logger = LoggerFactory.getLogger(LongRunningTaskService.class);
@Inject
private LongRunningTaskDao dao;
public Optional getLastRun(TaskTracker tracker){
String name = tracker.getName();
var firstPrefix = new LongRunningTaskKey(name, null, null);
var lastPrefix = new LongRunningTaskKey(
name,
MilliTime.of(tracker.getScheduledTime()),
tracker.getServerName());
Range range = new Range<>(firstPrefix, true, lastPrefix, false);
return dao.scan(range)
.reduce((firstRun, secondRun) -> secondRun);
}
public Optional findLastSuccess(String name){
var key = new LongRunningTaskKey(name, null, null);
return dao.scanWithPrefix(key)
.include(task -> task.getJobExecutionStatus() == LongRunningTaskStatus.SUCCESS)
.map(LongRunningTask::getFinish)
.exclude(Objects::isNull)
.map(MilliTime::toInstant)
.findMax(Instant::compareTo);
}
public boolean isRunning(String name){
var key = new LongRunningTaskKey(name, null, null);
return dao.scanWithPrefix(key)
.findLast()
.map(LongRunningTask::isRunning)
.orElse(false);
}
public Optional findLastNonRunningStatusTask(String name){
var key = new LongRunningTaskKey(name, null, null);
return dao.scanWithPrefix(key)
.exclude(task -> task.getJobExecutionStatus() == LongRunningTaskStatus.RUNNING)
.exclude(task -> {
if(task.getFinish() == null){
logger.warn("LongRunningTask={} with status={} has null finishedTime.", task, task
.getJobExecutionStatus());
return true;
}
return false;
})
.sort(Comparator.comparing(LongRunningTask::getFinish))
.findLast();
}
public LongRunningTaskSummaryDto getSummary(){
Map lastCompletions = new HashMap<>();
Map currentlyRunningTasks = new HashMap<>();
Map> runningOnServers = new HashMap<>();
for(LongRunningTask task : dao.scan().iterable()){
String name = task.getKey().getName();
if(task.isRunning()){
// currentlyRunningTasks
LongRunningTask current = currentlyRunningTasks.get(name);
if(current == null || task.getStart().isAfter(current.getStart())){
currentlyRunningTasks.put(name, task);
}
// runningOnServers
runningOnServers.putIfAbsent(name, new TreeSet<>());
runningOnServers.get(name).add(task.getKey().getServerName());
}
// lastCompletions
if(task.isSuccess()){
LongRunningTask current = lastCompletions.get(name);
if(current == null || task.getFinish().isAfter(current.getFinish())){
lastCompletions.put(name, task);
}
}
}
return new LongRunningTaskSummaryDto(lastCompletions, currentlyRunningTasks, runningOnServers);
}
public static class LongRunningTaskSummaryDto{
public final Map lastCompletions;
public final Map currentlyRunningTasks;
public final Map> runningOnServers;
public LongRunningTaskSummaryDto(Map lastCompletions,
Map currentlyRunningTasks, Map> runningOnServers){
this.lastCompletions = lastCompletions;
this.currentlyRunningTasks = currentlyRunningTasks;
this.runningOnServers = runningOnServers;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy