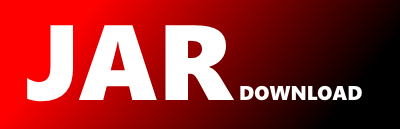
io.dataspray.stream.control.client.AuthNzApi Maven / Gradle / Ivy
/*
* DataSpray API
* DataSpray API documentation for controlling and development.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.dataspray.stream.control.client;
import io.dataspray.stream.control.client.ApiCallback;
import io.dataspray.stream.control.client.ApiClient;
import io.dataspray.stream.control.client.ApiException;
import io.dataspray.stream.control.client.ApiResponse;
import io.dataspray.stream.control.client.Configuration;
import io.dataspray.stream.control.client.Pair;
import io.dataspray.stream.control.client.ProgressRequestBody;
import io.dataspray.stream.control.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.dataspray.stream.control.client.model.ApiKeyCreate;
import io.dataspray.stream.control.client.model.ApiKeyWithSecret;
import io.dataspray.stream.control.client.model.ApiKeys;
import io.dataspray.stream.control.client.model.SignInChallengePasswordChangeRequest;
import io.dataspray.stream.control.client.model.SignInChallengeTotpCodeRequest;
import io.dataspray.stream.control.client.model.SignInRefreshTokenRequest;
import io.dataspray.stream.control.client.model.SignInRequest;
import io.dataspray.stream.control.client.model.SignInResponse;
import io.dataspray.stream.control.client.model.SignOutRequest;
import io.dataspray.stream.control.client.model.SignUpConfirmCodeRequest;
import io.dataspray.stream.control.client.model.SignUpRequest;
import io.dataspray.stream.control.client.model.SignUpResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class AuthNzApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public AuthNzApi() {
this(Configuration.getDefaultApiClient());
}
public AuthNzApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
/**
* Build call for createApiKey
* @param organizationName (required)
* @param apiKeyCreate (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call createApiKeyCall(String organizationName, ApiKeyCreate apiKeyCreate, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = apiKeyCreate;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/auth/apikey"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call createApiKeyValidateBeforeCall(String organizationName, ApiKeyCreate apiKeyCreate, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling createApiKey(Async)");
}
// verify the required parameter 'apiKeyCreate' is set
if (apiKeyCreate == null) {
throw new ApiException("Missing the required parameter 'apiKeyCreate' when calling createApiKey(Async)");
}
return createApiKeyCall(organizationName, apiKeyCreate, _callback);
}
/**
*
*
* @param organizationName (required)
* @param apiKeyCreate (required)
* @return ApiKeyWithSecret
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiKeyWithSecret createApiKey(String organizationName, ApiKeyCreate apiKeyCreate) throws ApiException {
ApiResponse localVarResp = createApiKeyWithHttpInfo(organizationName, apiKeyCreate);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param apiKeyCreate (required)
* @return ApiResponse<ApiKeyWithSecret>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse createApiKeyWithHttpInfo(String organizationName, ApiKeyCreate apiKeyCreate) throws ApiException {
okhttp3.Call localVarCall = createApiKeyValidateBeforeCall(organizationName, apiKeyCreate, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param apiKeyCreate (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call createApiKeyAsync(String organizationName, ApiKeyCreate apiKeyCreate, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = createApiKeyValidateBeforeCall(organizationName, apiKeyCreate, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for listApiKeys
* @param organizationName (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call listApiKeysCall(String organizationName, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/auth/apikeys"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call listApiKeysValidateBeforeCall(String organizationName, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling listApiKeys(Async)");
}
return listApiKeysCall(organizationName, _callback);
}
/**
*
*
* @param organizationName (required)
* @return ApiKeys
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiKeys listApiKeys(String organizationName) throws ApiException {
ApiResponse localVarResp = listApiKeysWithHttpInfo(organizationName);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @return ApiResponse<ApiKeys>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse listApiKeysWithHttpInfo(String organizationName) throws ApiException {
okhttp3.Call localVarCall = listApiKeysValidateBeforeCall(organizationName, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call listApiKeysAsync(String organizationName, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = listApiKeysValidateBeforeCall(organizationName, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for revokeApiKey
* @param organizationName (required)
* @param apiKeyId (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call revokeApiKeyCall(String organizationName, String apiKeyId, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/auth/apikey/{apiKeyId}"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "apiKeyId" + "}", localVarApiClient.escapeString(apiKeyId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call revokeApiKeyValidateBeforeCall(String organizationName, String apiKeyId, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling revokeApiKey(Async)");
}
// verify the required parameter 'apiKeyId' is set
if (apiKeyId == null) {
throw new ApiException("Missing the required parameter 'apiKeyId' when calling revokeApiKey(Async)");
}
return revokeApiKeyCall(organizationName, apiKeyId, _callback);
}
/**
*
*
* @param organizationName (required)
* @param apiKeyId (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void revokeApiKey(String organizationName, String apiKeyId) throws ApiException {
revokeApiKeyWithHttpInfo(organizationName, apiKeyId);
}
/**
*
*
* @param organizationName (required)
* @param apiKeyId (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse revokeApiKeyWithHttpInfo(String organizationName, String apiKeyId) throws ApiException {
okhttp3.Call localVarCall = revokeApiKeyValidateBeforeCall(organizationName, apiKeyId, null);
return localVarApiClient.execute(localVarCall);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param apiKeyId (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call revokeApiKeyAsync(String organizationName, String apiKeyId, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = revokeApiKeyValidateBeforeCall(organizationName, apiKeyId, _callback);
localVarApiClient.executeAsync(localVarCall, _callback);
return localVarCall;
}
/**
* Build call for signIn
* @param signInRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call signInCall(SignInRequest signInRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = signInRequest;
// create path and map variables
String localVarPath = "/v1/auth/sign-in";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call signInValidateBeforeCall(SignInRequest signInRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'signInRequest' is set
if (signInRequest == null) {
throw new ApiException("Missing the required parameter 'signInRequest' when calling signIn(Async)");
}
return signInCall(signInRequest, _callback);
}
/**
*
*
* @param signInRequest (required)
* @return SignInResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SignInResponse signIn(SignInRequest signInRequest) throws ApiException {
ApiResponse localVarResp = signInWithHttpInfo(signInRequest);
return localVarResp.getData();
}
/**
*
*
* @param signInRequest (required)
* @return ApiResponse<SignInResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signInWithHttpInfo(SignInRequest signInRequest) throws ApiException {
okhttp3.Call localVarCall = signInValidateBeforeCall(signInRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param signInRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call signInAsync(SignInRequest signInRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = signInValidateBeforeCall(signInRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for signInChallengePasswordChange
* @param signInChallengePasswordChangeRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call signInChallengePasswordChangeCall(SignInChallengePasswordChangeRequest signInChallengePasswordChangeRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = signInChallengePasswordChangeRequest;
// create path and map variables
String localVarPath = "/v1/auth/sign-in/password-change";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call signInChallengePasswordChangeValidateBeforeCall(SignInChallengePasswordChangeRequest signInChallengePasswordChangeRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'signInChallengePasswordChangeRequest' is set
if (signInChallengePasswordChangeRequest == null) {
throw new ApiException("Missing the required parameter 'signInChallengePasswordChangeRequest' when calling signInChallengePasswordChange(Async)");
}
return signInChallengePasswordChangeCall(signInChallengePasswordChangeRequest, _callback);
}
/**
*
*
* @param signInChallengePasswordChangeRequest (required)
* @return SignInResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SignInResponse signInChallengePasswordChange(SignInChallengePasswordChangeRequest signInChallengePasswordChangeRequest) throws ApiException {
ApiResponse localVarResp = signInChallengePasswordChangeWithHttpInfo(signInChallengePasswordChangeRequest);
return localVarResp.getData();
}
/**
*
*
* @param signInChallengePasswordChangeRequest (required)
* @return ApiResponse<SignInResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signInChallengePasswordChangeWithHttpInfo(SignInChallengePasswordChangeRequest signInChallengePasswordChangeRequest) throws ApiException {
okhttp3.Call localVarCall = signInChallengePasswordChangeValidateBeforeCall(signInChallengePasswordChangeRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param signInChallengePasswordChangeRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call signInChallengePasswordChangeAsync(SignInChallengePasswordChangeRequest signInChallengePasswordChangeRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = signInChallengePasswordChangeValidateBeforeCall(signInChallengePasswordChangeRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for signInChallengeTotpCode
* @param signInChallengeTotpCodeRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call signInChallengeTotpCodeCall(SignInChallengeTotpCodeRequest signInChallengeTotpCodeRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = signInChallengeTotpCodeRequest;
// create path and map variables
String localVarPath = "/v1/auth/sign-in/totp";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call signInChallengeTotpCodeValidateBeforeCall(SignInChallengeTotpCodeRequest signInChallengeTotpCodeRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'signInChallengeTotpCodeRequest' is set
if (signInChallengeTotpCodeRequest == null) {
throw new ApiException("Missing the required parameter 'signInChallengeTotpCodeRequest' when calling signInChallengeTotpCode(Async)");
}
return signInChallengeTotpCodeCall(signInChallengeTotpCodeRequest, _callback);
}
/**
*
*
* @param signInChallengeTotpCodeRequest (required)
* @return SignInResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SignInResponse signInChallengeTotpCode(SignInChallengeTotpCodeRequest signInChallengeTotpCodeRequest) throws ApiException {
ApiResponse localVarResp = signInChallengeTotpCodeWithHttpInfo(signInChallengeTotpCodeRequest);
return localVarResp.getData();
}
/**
*
*
* @param signInChallengeTotpCodeRequest (required)
* @return ApiResponse<SignInResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signInChallengeTotpCodeWithHttpInfo(SignInChallengeTotpCodeRequest signInChallengeTotpCodeRequest) throws ApiException {
okhttp3.Call localVarCall = signInChallengeTotpCodeValidateBeforeCall(signInChallengeTotpCodeRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param signInChallengeTotpCodeRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call signInChallengeTotpCodeAsync(SignInChallengeTotpCodeRequest signInChallengeTotpCodeRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = signInChallengeTotpCodeValidateBeforeCall(signInChallengeTotpCodeRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for signInRefreshToken
* @param signInRefreshTokenRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call signInRefreshTokenCall(SignInRefreshTokenRequest signInRefreshTokenRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = signInRefreshTokenRequest;
// create path and map variables
String localVarPath = "/v1/auth/sign-in/refresh";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call signInRefreshTokenValidateBeforeCall(SignInRefreshTokenRequest signInRefreshTokenRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'signInRefreshTokenRequest' is set
if (signInRefreshTokenRequest == null) {
throw new ApiException("Missing the required parameter 'signInRefreshTokenRequest' when calling signInRefreshToken(Async)");
}
return signInRefreshTokenCall(signInRefreshTokenRequest, _callback);
}
/**
*
*
* @param signInRefreshTokenRequest (required)
* @return SignInResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SignInResponse signInRefreshToken(SignInRefreshTokenRequest signInRefreshTokenRequest) throws ApiException {
ApiResponse localVarResp = signInRefreshTokenWithHttpInfo(signInRefreshTokenRequest);
return localVarResp.getData();
}
/**
*
*
* @param signInRefreshTokenRequest (required)
* @return ApiResponse<SignInResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signInRefreshTokenWithHttpInfo(SignInRefreshTokenRequest signInRefreshTokenRequest) throws ApiException {
okhttp3.Call localVarCall = signInRefreshTokenValidateBeforeCall(signInRefreshTokenRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param signInRefreshTokenRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call signInRefreshTokenAsync(SignInRefreshTokenRequest signInRefreshTokenRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = signInRefreshTokenValidateBeforeCall(signInRefreshTokenRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for signOut
* @param signOutRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call signOutCall(SignOutRequest signOutRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = signOutRequest;
// create path and map variables
String localVarPath = "/v1/auth/sign-out";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call signOutValidateBeforeCall(SignOutRequest signOutRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'signOutRequest' is set
if (signOutRequest == null) {
throw new ApiException("Missing the required parameter 'signOutRequest' when calling signOut(Async)");
}
return signOutCall(signOutRequest, _callback);
}
/**
*
*
* @param signOutRequest (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void signOut(SignOutRequest signOutRequest) throws ApiException {
signOutWithHttpInfo(signOutRequest);
}
/**
*
*
* @param signOutRequest (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signOutWithHttpInfo(SignOutRequest signOutRequest) throws ApiException {
okhttp3.Call localVarCall = signOutValidateBeforeCall(signOutRequest, null);
return localVarApiClient.execute(localVarCall);
}
/**
* (asynchronously)
*
* @param signOutRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call signOutAsync(SignOutRequest signOutRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = signOutValidateBeforeCall(signOutRequest, _callback);
localVarApiClient.executeAsync(localVarCall, _callback);
return localVarCall;
}
/**
* Build call for signUp
* @param signUpRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call signUpCall(SignUpRequest signUpRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = signUpRequest;
// create path and map variables
String localVarPath = "/v1/auth/sign-up";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call signUpValidateBeforeCall(SignUpRequest signUpRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'signUpRequest' is set
if (signUpRequest == null) {
throw new ApiException("Missing the required parameter 'signUpRequest' when calling signUp(Async)");
}
return signUpCall(signUpRequest, _callback);
}
/**
*
*
* @param signUpRequest (required)
* @return SignUpResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SignUpResponse signUp(SignUpRequest signUpRequest) throws ApiException {
ApiResponse localVarResp = signUpWithHttpInfo(signUpRequest);
return localVarResp.getData();
}
/**
*
*
* @param signUpRequest (required)
* @return ApiResponse<SignUpResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signUpWithHttpInfo(SignUpRequest signUpRequest) throws ApiException {
okhttp3.Call localVarCall = signUpValidateBeforeCall(signUpRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param signUpRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call signUpAsync(SignUpRequest signUpRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = signUpValidateBeforeCall(signUpRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for signUpConfirmCode
* @param signUpConfirmCodeRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call signUpConfirmCodeCall(SignUpConfirmCodeRequest signUpConfirmCodeRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = signUpConfirmCodeRequest;
// create path and map variables
String localVarPath = "/v1/auth/sign-up/code";
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { };
return localVarApiClient.buildCall(basePath, localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call signUpConfirmCodeValidateBeforeCall(SignUpConfirmCodeRequest signUpConfirmCodeRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'signUpConfirmCodeRequest' is set
if (signUpConfirmCodeRequest == null) {
throw new ApiException("Missing the required parameter 'signUpConfirmCodeRequest' when calling signUpConfirmCode(Async)");
}
return signUpConfirmCodeCall(signUpConfirmCodeRequest, _callback);
}
/**
*
*
* @param signUpConfirmCodeRequest (required)
* @return SignUpResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public SignUpResponse signUpConfirmCode(SignUpConfirmCodeRequest signUpConfirmCodeRequest) throws ApiException {
ApiResponse localVarResp = signUpConfirmCodeWithHttpInfo(signUpConfirmCodeRequest);
return localVarResp.getData();
}
/**
*
*
* @param signUpConfirmCodeRequest (required)
* @return ApiResponse<SignUpResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse signUpConfirmCodeWithHttpInfo(SignUpConfirmCodeRequest signUpConfirmCodeRequest) throws ApiException {
okhttp3.Call localVarCall = signUpConfirmCodeValidateBeforeCall(signUpConfirmCodeRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param signUpConfirmCodeRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call signUpConfirmCodeAsync(SignUpConfirmCodeRequest signUpConfirmCodeRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = signUpConfirmCodeValidateBeforeCall(signUpConfirmCodeRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy