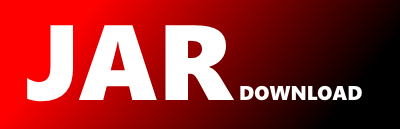
io.dataspray.stream.control.client.ControlApi Maven / Gradle / Ivy
The newest version!
/*
* DataSpray API
* DataSpray API documentation for controlling and development.
*
* The version of the OpenAPI document: 1.0.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.dataspray.stream.control.client;
import io.dataspray.stream.control.client.ApiCallback;
import io.dataspray.stream.control.client.ApiClient;
import io.dataspray.stream.control.client.ApiException;
import io.dataspray.stream.control.client.ApiResponse;
import io.dataspray.stream.control.client.Configuration;
import io.dataspray.stream.control.client.Pair;
import io.dataspray.stream.control.client.ProgressRequestBody;
import io.dataspray.stream.control.client.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.dataspray.stream.control.client.model.DeployRequest;
import io.dataspray.stream.control.client.model.TaskStatus;
import io.dataspray.stream.control.client.model.TaskStatuses;
import io.dataspray.stream.control.client.model.TaskVersion;
import io.dataspray.stream.control.client.model.TaskVersions;
import io.dataspray.stream.control.client.model.Topics;
import io.dataspray.stream.control.client.model.UploadCodeRequest;
import io.dataspray.stream.control.client.model.UploadCodeResponse;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class ControlApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public ControlApi() {
this(Configuration.getDefaultApiClient());
}
public ControlApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
/**
* Build call for activateVersion
* @param organizationName (required)
* @param taskId (required)
* @param version (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call activateVersionCall(String organizationName, String taskId, String version, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/task/{taskId}/activate"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "taskId" + "}", localVarApiClient.escapeString(taskId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (version != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("version", version));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call activateVersionValidateBeforeCall(String organizationName, String taskId, String version, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling activateVersion(Async)");
}
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException("Missing the required parameter 'taskId' when calling activateVersion(Async)");
}
// verify the required parameter 'version' is set
if (version == null) {
throw new ApiException("Missing the required parameter 'version' when calling activateVersion(Async)");
}
return activateVersionCall(organizationName, taskId, version, _callback);
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @param version (required)
* @return TaskStatus
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskStatus activateVersion(String organizationName, String taskId, String version) throws ApiException {
ApiResponse localVarResp = activateVersionWithHttpInfo(organizationName, taskId, version);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @param version (required)
* @return ApiResponse<TaskStatus>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse activateVersionWithHttpInfo(String organizationName, String taskId, String version) throws ApiException {
okhttp3.Call localVarCall = activateVersionValidateBeforeCall(organizationName, taskId, version, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param taskId (required)
* @param version (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call activateVersionAsync(String organizationName, String taskId, String version, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = activateVersionValidateBeforeCall(organizationName, taskId, version, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for delete
* @param organizationName (required)
* @param taskId (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call deleteCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/task/{taskId}/delete"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "taskId" + "}", localVarApiClient.escapeString(taskId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "DELETE", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call deleteValidateBeforeCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling delete(Async)");
}
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException("Missing the required parameter 'taskId' when calling delete(Async)");
}
return deleteCall(organizationName, taskId, _callback);
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return TaskStatus
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskStatus delete(String organizationName, String taskId) throws ApiException {
ApiResponse localVarResp = deleteWithHttpInfo(organizationName, taskId);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return ApiResponse<TaskStatus>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deleteWithHttpInfo(String organizationName, String taskId) throws ApiException {
okhttp3.Call localVarCall = deleteValidateBeforeCall(organizationName, taskId, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param taskId (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call deleteAsync(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = deleteValidateBeforeCall(organizationName, taskId, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for deployVersion
* @param organizationName (required)
* @param taskId (required)
* @param deployRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call deployVersionCall(String organizationName, String taskId, DeployRequest deployRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = deployRequest;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/task/{taskId}/deploy"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "taskId" + "}", localVarApiClient.escapeString(taskId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call deployVersionValidateBeforeCall(String organizationName, String taskId, DeployRequest deployRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling deployVersion(Async)");
}
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException("Missing the required parameter 'taskId' when calling deployVersion(Async)");
}
// verify the required parameter 'deployRequest' is set
if (deployRequest == null) {
throw new ApiException("Missing the required parameter 'deployRequest' when calling deployVersion(Async)");
}
return deployVersionCall(organizationName, taskId, deployRequest, _callback);
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @param deployRequest (required)
* @return TaskVersion
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskVersion deployVersion(String organizationName, String taskId, DeployRequest deployRequest) throws ApiException {
ApiResponse localVarResp = deployVersionWithHttpInfo(organizationName, taskId, deployRequest);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @param deployRequest (required)
* @return ApiResponse<TaskVersion>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse deployVersionWithHttpInfo(String organizationName, String taskId, DeployRequest deployRequest) throws ApiException {
okhttp3.Call localVarCall = deployVersionValidateBeforeCall(organizationName, taskId, deployRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param taskId (required)
* @param deployRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call deployVersionAsync(String organizationName, String taskId, DeployRequest deployRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = deployVersionValidateBeforeCall(organizationName, taskId, deployRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for getTopics
* @param organizationName (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getTopicsCall(String organizationName, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/topics"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getTopicsValidateBeforeCall(String organizationName, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling getTopics(Async)");
}
return getTopicsCall(organizationName, _callback);
}
/**
*
*
* @param organizationName (required)
* @return Topics
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public Topics getTopics(String organizationName) throws ApiException {
ApiResponse localVarResp = getTopicsWithHttpInfo(organizationName);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @return ApiResponse<Topics>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getTopicsWithHttpInfo(String organizationName) throws ApiException {
okhttp3.Call localVarCall = getTopicsValidateBeforeCall(organizationName, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getTopicsAsync(String organizationName, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getTopicsValidateBeforeCall(organizationName, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for getVersions
* @param organizationName (required)
* @param taskId (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call getVersionsCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/task/{taskId}/versions"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "taskId" + "}", localVarApiClient.escapeString(taskId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call getVersionsValidateBeforeCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling getVersions(Async)");
}
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException("Missing the required parameter 'taskId' when calling getVersions(Async)");
}
return getVersionsCall(organizationName, taskId, _callback);
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return TaskVersions
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskVersions getVersions(String organizationName, String taskId) throws ApiException {
ApiResponse localVarResp = getVersionsWithHttpInfo(organizationName, taskId);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return ApiResponse<TaskVersions>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse getVersionsWithHttpInfo(String organizationName, String taskId) throws ApiException {
okhttp3.Call localVarCall = getVersionsValidateBeforeCall(organizationName, taskId, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param taskId (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call getVersionsAsync(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = getVersionsValidateBeforeCall(organizationName, taskId, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for pause
* @param organizationName (required)
* @param taskId (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call pauseCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/task/{taskId}/pause"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "taskId" + "}", localVarApiClient.escapeString(taskId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call pauseValidateBeforeCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling pause(Async)");
}
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException("Missing the required parameter 'taskId' when calling pause(Async)");
}
return pauseCall(organizationName, taskId, _callback);
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return TaskStatus
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskStatus pause(String organizationName, String taskId) throws ApiException {
ApiResponse localVarResp = pauseWithHttpInfo(organizationName, taskId);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return ApiResponse<TaskStatus>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse pauseWithHttpInfo(String organizationName, String taskId) throws ApiException {
okhttp3.Call localVarCall = pauseValidateBeforeCall(organizationName, taskId, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param taskId (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call pauseAsync(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = pauseValidateBeforeCall(organizationName, taskId, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for resume
* @param organizationName (required)
* @param taskId (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call resumeCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/task/{taskId}/resume"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "taskId" + "}", localVarApiClient.escapeString(taskId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "PATCH", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call resumeValidateBeforeCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling resume(Async)");
}
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException("Missing the required parameter 'taskId' when calling resume(Async)");
}
return resumeCall(organizationName, taskId, _callback);
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return TaskStatus
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskStatus resume(String organizationName, String taskId) throws ApiException {
ApiResponse localVarResp = resumeWithHttpInfo(organizationName, taskId);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return ApiResponse<TaskStatus>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse resumeWithHttpInfo(String organizationName, String taskId) throws ApiException {
okhttp3.Call localVarCall = resumeValidateBeforeCall(organizationName, taskId, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param taskId (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call resumeAsync(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = resumeValidateBeforeCall(organizationName, taskId, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for status
* @param organizationName (required)
* @param taskId (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call statusCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/task/{taskId}/status/"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()))
.replace("{" + "taskId" + "}", localVarApiClient.escapeString(taskId.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call statusValidateBeforeCall(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling status(Async)");
}
// verify the required parameter 'taskId' is set
if (taskId == null) {
throw new ApiException("Missing the required parameter 'taskId' when calling status(Async)");
}
return statusCall(organizationName, taskId, _callback);
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return TaskStatus
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskStatus status(String organizationName, String taskId) throws ApiException {
ApiResponse localVarResp = statusWithHttpInfo(organizationName, taskId);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param taskId (required)
* @return ApiResponse<TaskStatus>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse statusWithHttpInfo(String organizationName, String taskId) throws ApiException {
okhttp3.Call localVarCall = statusValidateBeforeCall(organizationName, taskId, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param taskId (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call statusAsync(String organizationName, String taskId, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = statusValidateBeforeCall(organizationName, taskId, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for statusAll
* @param organizationName (required)
* @param cursor (optional)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call statusAllCall(String organizationName, String cursor, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/status"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (cursor != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("cursor", cursor));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "GET", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call statusAllValidateBeforeCall(String organizationName, String cursor, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling statusAll(Async)");
}
return statusAllCall(organizationName, cursor, _callback);
}
/**
*
*
* @param organizationName (required)
* @param cursor (optional)
* @return TaskStatuses
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public TaskStatuses statusAll(String organizationName, String cursor) throws ApiException {
ApiResponse localVarResp = statusAllWithHttpInfo(organizationName, cursor);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param cursor (optional)
* @return ApiResponse<TaskStatuses>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse statusAllWithHttpInfo(String organizationName, String cursor) throws ApiException {
okhttp3.Call localVarCall = statusAllValidateBeforeCall(organizationName, cursor, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param cursor (optional)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call statusAllAsync(String organizationName, String cursor, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = statusAllValidateBeforeCall(organizationName, cursor, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
/**
* Build call for uploadCode
* @param organizationName (required)
* @param uploadCodeRequest (required)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
*/
public okhttp3.Call uploadCodeCall(String organizationName, UploadCodeRequest uploadCodeRequest, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = uploadCodeRequest;
// create path and map variables
String localVarPath = "/v1/organization/{organizationName}/control/code/upload"
.replace("{" + "organizationName" + "}", localVarApiClient.escapeString(organizationName.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/json"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "Authorizer" };
return localVarApiClient.buildCall(basePath, localVarPath, "PUT", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call uploadCodeValidateBeforeCall(String organizationName, UploadCodeRequest uploadCodeRequest, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'organizationName' is set
if (organizationName == null) {
throw new ApiException("Missing the required parameter 'organizationName' when calling uploadCode(Async)");
}
// verify the required parameter 'uploadCodeRequest' is set
if (uploadCodeRequest == null) {
throw new ApiException("Missing the required parameter 'uploadCodeRequest' when calling uploadCode(Async)");
}
return uploadCodeCall(organizationName, uploadCodeRequest, _callback);
}
/**
*
*
* @param organizationName (required)
* @param uploadCodeRequest (required)
* @return UploadCodeResponse
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public UploadCodeResponse uploadCode(String organizationName, UploadCodeRequest uploadCodeRequest) throws ApiException {
ApiResponse localVarResp = uploadCodeWithHttpInfo(organizationName, uploadCodeRequest);
return localVarResp.getData();
}
/**
*
*
* @param organizationName (required)
* @param uploadCodeRequest (required)
* @return ApiResponse<UploadCodeResponse>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse uploadCodeWithHttpInfo(String organizationName, UploadCodeRequest uploadCodeRequest) throws ApiException {
okhttp3.Call localVarCall = uploadCodeValidateBeforeCall(organizationName, uploadCodeRequest, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
*
* @param organizationName (required)
* @param uploadCodeRequest (required)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public okhttp3.Call uploadCodeAsync(String organizationName, UploadCodeRequest uploadCodeRequest, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = uploadCodeValidateBeforeCall(organizationName, uploadCodeRequest, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy