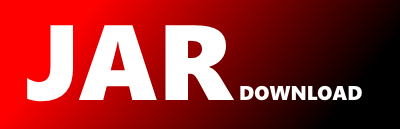
io.dataspray.singletable.Schema Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of single-table Show documentation
Show all versions of single-table Show documentation
DynamoDB best practices encourages a single-table design that allows multiple record types to reside within the
same table. The goal of this library is to improve the experience of Java developers and make it safer to define
non-conflicting schema of each record, serializing and deserializing automatically and working with secondary
indexes.
The newest version!
// SPDX-FileCopyrightText: 2019-2022 Matus Faro
// SPDX-License-Identifier: Apache-2.0
package io.dataspray.singletable;
import com.google.common.collect.ImmutableMap;
import software.amazon.awssdk.services.dynamodb.model.AttributeValue;
import software.amazon.awssdk.services.dynamodb.model.Condition;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
public interface Schema {
String tableName();
Map primaryKey(T obj);
Map primaryKey(Map values);
String partitionKeyName();
Entry partitionKey(T obj);
Entry partitionKey(Map values);
Map attrMapToConditions(Entry attrEntry);
Map attrMapToConditions(Map attrMap);
/**
* Use if shardKeys are set but partitionKeys are empty
*/
Entry shardKey(int shard);
/**
* Use if shardKeys and partitionKeys are both needed
*/
Entry shardKey(int shard, Map values);
String partitionKeyValue(T obj);
String partitionKeyValue(Map values);
String rangeKeyName();
Entry rangeKey(T obj);
Entry rangeKey(Map values);
/**
* Retrieve sort key from incomplete given values.
* Intended to be used by a range query.
*/
Entry rangeKeyPartial(Map values);
/**
* Retrieve sort key value from incomplete given values.
* Intended to be used by a range query.
*/
String rangeValuePartial(Map values);
AttributeValue toAttrValue(Object object);
AttributeValue toAttrValue(String fieldName, Object object);
Object fromAttrValue(String fieldName, AttributeValue attrVal);
ImmutableMap toAttrMap(T obj);
T fromAttrMap(Map attrMap);
/**
* Shard count or -1 if shardKeys are not set
*/
int shardCount();
Optional serializeLastEvaluatedKey(Map lastEvaluatedKey);
Map toExclusiveStartKey(String serializedlastEvaluatedKey);
String serializeShardedLastEvaluatedKey(Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy