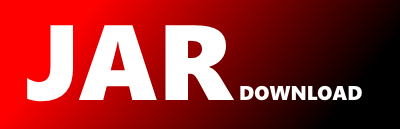
io.dataspray.singletable.SingleTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of single-table Show documentation
Show all versions of single-table Show documentation
DynamoDB best practices encourages a single-table design that allows multiple record types to reside within the
same table. The goal of this library is to improve the experience of Java developers and make it safer to define
non-conflicting schema of each record, serializing and deserializing automatically and working with secondary
indexes.
The newest version!
// SPDX-FileCopyrightText: 2019-2022 Matus Faro
// SPDX-License-Identifier: Apache-2.0
package io.dataspray.singletable;
import com.dampcake.gson.immutable.ImmutableAdapterFactory;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.base.Strings;
import com.google.gson.FieldNamingPolicy;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import lombok.Builder;
import software.amazon.awscdk.services.dynamodb.Table;
import software.amazon.awssdk.services.dynamodb.DynamoDbClient;
import software.amazon.awssdk.services.dynamodb.model.QueryRequest;
import software.constructs.Construct;
import javax.annotation.Nullable;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import java.util.function.Consumer;
public class SingleTable implements DynamoMapper {
public static final String TTL_IN_EPOCH_SEC_ATTR_NAME = "ttlInEpochSec";
@VisibleForTesting
DynamoMapperImpl mapper;
@VisibleForTesting
DynamoUtil util;
@Builder
private SingleTable(
@Nullable String tableName,
@Deprecated @Nullable String tablePrefix,
@Nullable Gson overrideGson,
@Nullable List> overrideTypeConverters,
@Nullable List> overrideCollectionTypeConverters) {
if (Strings.isNullOrEmpty(tablePrefix) == Strings.isNullOrEmpty(tableName)) {
throw new RuntimeException("Must specify either tableName or tablePrefix");
}
Gson gson = overrideGson != null ? overrideGson : new GsonBuilder()
.setFieldNamingPolicy(FieldNamingPolicy.IDENTITY)
.disableHtmlEscaping()
.registerTypeAdapterFactory(ImmutableAdapterFactory.forGuava())
.create();
this.mapper = new DynamoMapperImpl(tableName, tablePrefix, gson, overrideTypeConverters, overrideCollectionTypeConverters);
this.util = new DynamoUtil();
}
@Override
public String getTableName() {
return mapper.getTableName();
}
@Override
public Table createCdkTable(Construct scope, String stackId, int lsiCount, int gsiCount) {
return mapper.createCdkTable(scope, stackId, lsiCount, gsiCount);
}
@Override
public void createTableIfNotExists(DynamoDbClient dynamo, int lsiCount, int gsiCount) {
mapper.createTableIfNotExists(dynamo, lsiCount, gsiCount);
}
@Override
public TableSchema parseTableSchema(Class objClazz) {
return mapper.parseTableSchema(objClazz);
}
@Override
public IndexSchema parseLocalSecondaryIndexSchema(long indexNumber, Class objClazz) {
return mapper.parseLocalSecondaryIndexSchema(indexNumber, objClazz);
}
@Override
public IndexSchema parseGlobalSecondaryIndexSchema(long indexNumber, Class objClazz) {
return mapper.parseGlobalSecondaryIndexSchema(indexNumber, objClazz);
}
@Override
public ShardedTableSchema parseShardedTableSchema(Class objClazz) {
return mapper.parseShardedTableSchema(objClazz);
}
@Override
public ShardedIndexSchema parseShardedLocalSecondaryIndexSchema(long indexNumber, Class objClazz) {
return mapper.parseShardedLocalSecondaryIndexSchema(indexNumber, objClazz);
}
@Override
public ShardedIndexSchema parseShardedGlobalSecondaryIndexSchema(long indexNumber, Class objClazz) {
return mapper.parseShardedGlobalSecondaryIndexSchema(indexNumber, objClazz);
}
/**
* @deprecated use {@link ShardedTableSchema#querySharded} or {@link ShardedIndexSchema#querySharded}
*/
@Deprecated
public ShardPageResult fetchShardNextPage(DynamoDbClient client, Schema schema, Optional cursorOpt, int maxPageSize) {
return util.fetchShardNextPage(client, schema, cursorOpt, maxPageSize);
}
/**
* @deprecated use {@link ShardedTableSchema#querySharded} or {@link ShardedIndexSchema#querySharded}
*/
@Deprecated
public ShardPageResult fetchShardNextPage(DynamoDbClient client, Schema schema, Optional cursorOpt, int maxPageSize, Map keyConditions) {
return util.fetchShardNextPage(client, schema, cursorOpt, maxPageSize, keyConditions);
}
/**
* @deprecated use {@link ShardedTableSchema#querySharded} or {@link ShardedIndexSchema#querySharded}
*/
@Deprecated
public ShardPageResult fetchShardNextPage(DynamoDbClient client, Schema schema, Optional cursorOpt, int maxPageSize, Map keyConditions, Consumer queryRequestConsumer) {
return util.fetchShardNextPage(client, schema, cursorOpt, maxPageSize, keyConditions, queryRequestConsumer);
}
/**
* @deprecated use {@link ShardedTableSchema#querySharded} or {@link ShardedIndexSchema#querySharded}
*/
@Deprecated
public int deterministicPartition(String input, int partitionCount) {
return DynamoUtil.deterministicPartition(input, partitionCount);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy