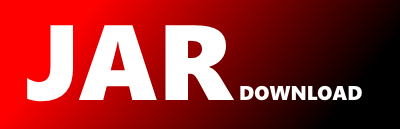
io.deephaven.engine.table.impl.TableKeyStateRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.table.impl;
import io.deephaven.engine.table.impl.locations.ImmutableTableKey;
import io.deephaven.hash.KeyedObjectHashMap;
import io.deephaven.hash.KeyedObjectKey;
import io.deephaven.engine.table.impl.locations.TableKey;
import org.jetbrains.annotations.NotNull;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* A registry for states keyed by {@link TableKey}.
*/
public class TableKeyStateRegistry {
private final KeyedObjectHashMap> registeredTableMaps =
new KeyedObjectHashMap<>(StateKey.getInstance());
/**
* Get (or create if none exists) a value for the supplied {@link TableKey}.
*
* @param tableKey The table key
* @return The associated value
*/
public VALUE_TYPE computeIfAbsent(@NotNull final TableKey tableKey,
@NotNull final Function valueFactory) {
return registeredTableMaps.putIfAbsent(tableKey, State::new, valueFactory).value;
}
public void forEach(@NotNull final Consumer consumer) {
registeredTableMaps.values().forEach(s -> consumer.accept(s.value));
}
public void clear() {
registeredTableMaps.clear();
}
private static class State {
private final ImmutableTableKey key;
private final VALUE_TYPE value;
private State(@NotNull final TableKey key, @NotNull final Function valueFactory) {
this.key = key.makeImmutable();
value = valueFactory.apply(key);
}
}
private static class StateKey extends KeyedObjectKey.Basic> {
@SuppressWarnings("rawtypes")
private static final StateKey INSTANCE = new StateKey();
private static KeyedObjectKey> getInstance() {
// noinspection unchecked
return (KeyedObjectKey>) INSTANCE;
}
private StateKey() {}
@Override
public TableKey getKey(@NotNull final State state) {
return state.key;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy