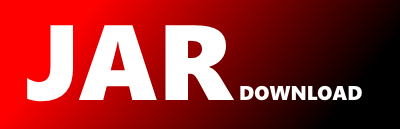
io.deephaven.engine.table.impl.by.BaseAddOnlyFirstOrLastChunkedOperator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.table.impl.by;
import io.deephaven.engine.table.Table;
import io.deephaven.engine.table.impl.MatchPair;
import io.deephaven.engine.table.ColumnSource;
import io.deephaven.engine.table.impl.sources.LongArraySource;
import io.deephaven.engine.table.impl.sources.RedirectedColumnSource;
import io.deephaven.chunk.*;
import io.deephaven.chunk.attributes.ChunkLengths;
import io.deephaven.chunk.attributes.ChunkPositions;
import io.deephaven.engine.rowset.chunkattributes.RowKeys;
import io.deephaven.chunk.attributes.Values;
import io.deephaven.engine.table.impl.util.LongColumnSourceWritableRowRedirection;
import java.util.LinkedHashMap;
import java.util.Map;
abstract class BaseAddOnlyFirstOrLastChunkedOperator
extends NoopStateChangeRecorder // We can never empty or reincarnate states since we're add-only
implements IterativeChunkedAggregationOperator {
final boolean isFirst;
final LongArraySource redirections;
private final LongColumnSourceWritableRowRedirection rowRedirection;
private final Map> resultColumns;
BaseAddOnlyFirstOrLastChunkedOperator(boolean isFirst, MatchPair[] resultPairs, Table originalTable,
String exposeRedirectionAs) {
this.isFirst = isFirst;
this.redirections = new LongArraySource();
this.rowRedirection = new LongColumnSourceWritableRowRedirection(redirections);
this.resultColumns = new LinkedHashMap<>(resultPairs.length);
for (final MatchPair mp : resultPairs) {
resultColumns.put(mp.leftColumn(), RedirectedColumnSource.maybeRedirect(
rowRedirection, originalTable.getColumnSource(mp.rightColumn())));
}
if (exposeRedirectionAs != null) {
resultColumns.put(exposeRedirectionAs, redirections);
}
}
@Override
public void removeChunk(BucketedContext bucketedContext, Chunk extends Values> values,
LongChunk extends RowKeys> inputRowKeys, IntChunk destinations,
IntChunk startPositions, IntChunk length,
WritableBooleanChunk stateModified) {
throw new UnsupportedOperationException();
}
@Override
public void modifyChunk(BucketedContext bucketedContext, Chunk extends Values> previousValues,
Chunk extends Values> newValues, LongChunk extends RowKeys> postShiftRowKeys,
IntChunk destinations, IntChunk startPositions, IntChunk length,
WritableBooleanChunk stateModified) {
// we have no inputs, so should never get here
throw new IllegalStateException();
}
@Override
public void shiftChunk(BucketedContext bucketedContext, Chunk extends Values> previousValues,
Chunk extends Values> newValues, LongChunk extends RowKeys> preShiftRowKeys,
LongChunk extends RowKeys> postShiftRowKeys, IntChunk destinations,
IntChunk startPositions, IntChunk length,
WritableBooleanChunk stateModified) {
throw new UnsupportedOperationException();
}
@Override
public void modifyRowKeys(BucketedContext context, LongChunk extends RowKeys> inputRowKeys,
IntChunk destinations, IntChunk startPositions,
IntChunk length,
WritableBooleanChunk stateModified) {
throw new UnsupportedOperationException();
}
@Override
public boolean removeChunk(SingletonContext singletonContext, int chunkSize, Chunk extends Values> values,
LongChunk extends RowKeys> inputRowKeys, long destination) {
throw new UnsupportedOperationException();
}
@Override
public boolean modifyChunk(SingletonContext singletonContext, int chunkSize, Chunk extends Values> previousValues,
Chunk extends Values> newValues, LongChunk extends RowKeys> postShiftRowKeys, long destination) {
// we have no inputs, so should never get here
throw new IllegalStateException();
}
@Override
public boolean shiftChunk(SingletonContext singletonContext, Chunk extends Values> previousValues,
Chunk extends Values> newValues, LongChunk extends RowKeys> preShiftRowKeys,
LongChunk extends RowKeys> postShiftRowKeys, long destination) {
throw new UnsupportedOperationException();
}
@Override
public boolean modifyRowKeys(SingletonContext context, LongChunk extends RowKeys> rowKeys, long destination) {
throw new UnsupportedOperationException();
}
@Override
public void ensureCapacity(long tableSize) {
redirections.ensureCapacity(tableSize);
}
@Override
public Map> getResultColumns() {
return resultColumns;
}
@Override
public void startTrackingPrevValues() {
rowRedirection.startTrackingPrevValues();
}
@Override
public boolean requiresRowKeys() {
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy