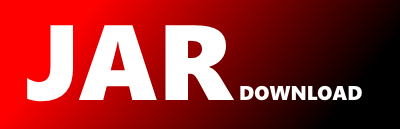
io.deephaven.engine.table.impl.join.stamp.IntReverseStampKernel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
/*
* ---------------------------------------------------------------------------------------------------------------------
* AUTO-GENERATED CLASS - DO NOT EDIT MANUALLY - for any changes edit CharStampKernel and regenerate
* ---------------------------------------------------------------------------------------------------------------------
*/
package io.deephaven.engine.table.impl.join.stamp;
import io.deephaven.chunk.*;
import io.deephaven.engine.rowset.chunkattributes.RowKeys;
import io.deephaven.chunk.attributes.Values;
import io.deephaven.engine.rowset.RowSequence;
public class IntReverseStampKernel implements StampKernel {
static final IntReverseStampKernel INSTANCE = new IntReverseStampKernel();
private IntReverseStampKernel() {} // static use only
@Override
public void computeRedirections(Chunk leftStamps, Chunk rightStamps, LongChunk rightKeyIndices, WritableLongChunk leftRedirections) {
computeRedirections(leftStamps.asIntChunk(), rightStamps.asIntChunk(), rightKeyIndices, leftRedirections);
}
static private void computeRedirections(IntChunk leftStamps, IntChunk rightStamps, LongChunk rightKeyIndices, WritableLongChunk leftRedirections) {
final int leftSize = leftStamps.size();
final int rightSize = rightStamps.size();
if (rightSize == 0) {
leftRedirections.fillWithValue(0, leftSize, RowSequence.NULL_ROW_KEY);
leftRedirections.setSize(leftSize);
return;
}
int rightLowIdx = 0;
int rightLowValue = rightStamps.get(0);
final int maxRightIdx = rightSize - 1;
for (int li = 0; li < leftSize; ) {
final int leftValue = leftStamps.get(li);
if (lt(leftValue, rightLowValue)) {
leftRedirections.set(li++, RowSequence.NULL_ROW_KEY);
continue;
}
else if (eq(leftValue, rightLowValue)) {
leftRedirections.set(li++, rightKeyIndices.get(rightLowIdx));
continue;
}
int rightHighIdx = rightSize;
while (rightLowIdx < rightHighIdx) {
final int rightMidIdx = ((rightHighIdx - rightLowIdx) / 2) + rightLowIdx;
final int rightMidValue = rightStamps.get(rightMidIdx);
if (leq(rightMidValue, leftValue)) {
rightLowIdx = rightMidIdx;
rightLowValue = rightMidValue;
if (rightLowIdx == rightHighIdx - 1 || eq(rightLowValue, leftValue)) {
break;
}
} else {
rightHighIdx = rightMidIdx;
}
}
final long redirectionKey = rightKeyIndices.get(rightLowIdx);
if (rightLowIdx == maxRightIdx) {
leftRedirections.fillWithValue(li, leftSize - li, redirectionKey);
return;
} else {
leftRedirections.set(li++, redirectionKey);
final int nextRightValue = rightStamps.get(rightLowIdx + 1);
while (li < leftSize && lt(leftStamps.get(li), nextRightValue)) {
leftRedirections.set(li++, redirectionKey);
}
}
}
}
// region comparison functions
// note that this is a descending kernel, thus the comparisons here are backwards (e.g., the lt function is in terms of the sort direction, so is implemented by gt)
private static int doComparison(int lhs, int rhs) {
return -1 * Integer.compare(lhs, rhs);
}
// endregion comparison functions
private static boolean lt(int lhs, int rhs) {
return doComparison(lhs, rhs) < 0;
}
private static boolean leq(int lhs, int rhs) {
return doComparison(lhs, rhs) <= 0;
}
private static boolean eq(int lhs, int rhs) {
// region equality function
return lhs == rhs;
// endregion equality function
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy