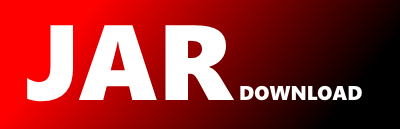
io.deephaven.engine.table.impl.naturaljoin.StaticHashedNaturalJoinStateManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.table.impl.naturaljoin;
import io.deephaven.engine.rowset.RowSet;
import io.deephaven.engine.table.ColumnSource;
import io.deephaven.engine.table.Table;
import io.deephaven.engine.table.impl.JoinControl;
import io.deephaven.engine.table.impl.QueryTable;
import io.deephaven.engine.table.impl.StaticNaturalJoinStateManager;
import io.deephaven.engine.table.impl.sources.*;
import io.deephaven.engine.table.impl.util.ContiguousWritableRowRedirection;
import io.deephaven.engine.table.impl.util.LongColumnSourceWritableRowRedirection;
import io.deephaven.engine.table.impl.util.WritableRowRedirection;
import io.deephaven.engine.table.impl.util.WritableRowRedirectionLockFree;
import java.util.function.LongUnaryOperator;
public abstract class StaticHashedNaturalJoinStateManager extends StaticNaturalJoinStateManager {
protected StaticHashedNaturalJoinStateManager(ColumnSource>[] keySourcesForErrorMessages) {
super(keySourcesForErrorMessages);
}
public abstract void buildFromLeftSide(final Table leftTable, ColumnSource>[] leftSources, final IntegerArraySource leftHashSlots);
public abstract void buildFromRightSide(final Table rightTable, ColumnSource> [] rightSources);
public abstract void decorateLeftSide(RowSet leftRowSet, ColumnSource> [] leftSources, final LongArraySource leftRedirections);
public abstract void decorateWithRightSide(Table rightTable, ColumnSource> [] rightSources);
public abstract WritableRowRedirection buildRowRedirectionFromHashSlot(QueryTable leftTable, boolean exactMatch, IntegerArraySource leftHashSlots, JoinControl.RedirectionType redirectionType);
public abstract WritableRowRedirection buildRowRedirectionFromRedirections(QueryTable leftTable, boolean exactMatch, LongArraySource leftRedirections, JoinControl.RedirectionType redirectionType);
public abstract WritableRowRedirection buildGroupedRowRedirection(QueryTable leftTable, boolean exactMatch, long groupingSize, IntegerArraySource leftHashSlots, ArrayBackedColumnSource leftIndices, JoinControl.RedirectionType redirectionType);
protected WritableRowRedirection buildGroupedRowRedirection(QueryTable leftTable, boolean exactMatch, long groupingSize, LongUnaryOperator groupPositionToRightSide, ArrayBackedColumnSource leftIndices, JoinControl.RedirectionType redirectionType) {
switch (redirectionType) {
case Contiguous: {
if (!leftTable.isFlat()) {
throw new IllegalStateException("Left table is not flat for contiguous row redirection build!");
}
// we can use an array, which is perfect for a small enough flat table
final long[] innerIndex = new long[leftTable.intSize("contiguous redirection build")];
for (int ii = 0; ii < groupingSize; ++ii) {
final long rightSide = groupPositionToRightSide.applyAsLong(ii);
checkExactMatch(exactMatch, ii, rightSide);
final RowSet leftRowSetForKey = leftIndices.get(ii);
leftRowSetForKey.forAllRowKeys((long ll) -> innerIndex[(int) ll] = rightSide);
}
return new ContiguousWritableRowRedirection(innerIndex);
}
case Sparse: {
final LongSparseArraySource sparseRedirections = new LongSparseArraySource();
for (int ii = 0; ii < groupingSize; ++ii) {
final long rightSide = groupPositionToRightSide.applyAsLong(ii);
checkExactMatch(exactMatch, ii, rightSide);
if (rightSide != NO_RIGHT_ENTRY_VALUE) {
final RowSet leftRowSetForKey = leftIndices.get(ii);
leftRowSetForKey.forAllRowKeys((long ll) -> sparseRedirections.set(ll, rightSide));
}
}
return new LongColumnSourceWritableRowRedirection(sparseRedirections);
}
case Hash: {
final WritableRowRedirection rowRedirection = WritableRowRedirectionLockFree.FACTORY.createRowRedirection(leftTable.intSize());
for (int ii = 0; ii < groupingSize; ++ii) {
final long rightSide = groupPositionToRightSide.applyAsLong(ii);
checkExactMatch(exactMatch, ii, rightSide);
if (rightSide != NO_RIGHT_ENTRY_VALUE) {
final RowSet leftRowSetForKey = leftIndices.get(ii);
leftRowSetForKey.forAllRowKeys((long ll) -> rowRedirection.put(ll, rightSide));
}
}
return rowRedirection;
}
}
throw new IllegalStateException("Bad redirectionType: " + redirectionType);
}
public void errorOnDuplicates(IntegerArraySource leftHashSlots, long size, LongUnaryOperator groupPositionToRightSide, LongUnaryOperator firstLeftKey) {
for (int ii = 0; ii < size; ++ii) {
final long rightSide = groupPositionToRightSide.applyAsLong(ii);
if (rightSide == DUPLICATE_RIGHT_VALUE) {
throw new IllegalStateException("Natural Join found duplicate right key for " + extractKeyStringFromSourceTable(firstLeftKey.applyAsLong(ii)));
}
}
}
public void errorOnDuplicatesGrouped(IntegerArraySource leftHashSlots, long size, ObjectArraySource rowSetSource) {
throw new UnsupportedOperationException();
}
public void errorOnDuplicatesSingle(IntegerArraySource leftHashSlots, long size, RowSet rowSet) {
throw new UnsupportedOperationException();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy