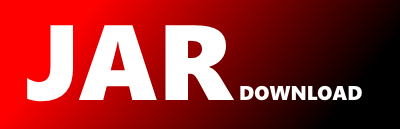
io.deephaven.engine.table.impl.remote.InitialSnapshotTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.table.impl.remote;
import io.deephaven.base.verify.Assert;
import io.deephaven.engine.rowset.WritableRowSet;
import io.deephaven.engine.rowset.RowSet;
import io.deephaven.engine.rowset.RowSetFactory;
import io.deephaven.engine.table.*;
import io.deephaven.engine.table.impl.QueryTable;
import io.deephaven.engine.table.impl.sources.ArrayBackedColumnSource;
import io.deephaven.engine.table.impl.sources.WritableRedirectedColumnSource;
import io.deephaven.engine.table.WritableColumnSource;
import io.deephaven.engine.table.impl.util.*;
import java.time.Instant;
import java.time.ZonedDateTime;
import java.util.BitSet;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
public class InitialSnapshotTable extends QueryTable {
protected final Setter>[] setters;
protected int capacity;
protected WritableRowSet freeset = RowSetFactory.empty();
protected final WritableRowSet populatedRows;
protected final WritableRowSet[] populatedCells;
protected WritableColumnSource>[] writableSources;
protected WritableRowRedirection rowRedirection;
private final BitSet subscribedColumns;
protected InitialSnapshotTable(Map> result,
WritableColumnSource>[] writableSources,
WritableRowRedirection rowRedirection, BitSet subscribedColumns) {
super(RowSetFactory.empty().toTracking(), result);
this.subscribedColumns = subscribedColumns;
this.writableSources = writableSources;
this.setters = new Setter[writableSources.length];
this.populatedCells = new WritableRowSet[writableSources.length];
for (int ii = 0; ii < writableSources.length; ++ii) {
setters[ii] = getSetter(writableSources[ii]);
this.populatedCells[ii] = RowSetFactory.fromKeys();
}
this.rowRedirection = rowRedirection;
this.populatedRows = RowSetFactory.fromKeys();
}
public BitSet getSubscribedColumns() {
return subscribedColumns;
}
public boolean isSubscribedColumn(int column) {
return subscribedColumns == null || subscribedColumns.get(column);
}
@SuppressWarnings("rawtypes")
protected Setter> getSetter(final WritableColumnSource source) {
if (source.getType() == byte.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else if (source.getType() == char.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else if (source.getType() == double.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else if (source.getType() == float.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else if (source.getType() == int.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else if (source.getType() == long.class
|| source.getType() == Instant.class
|| source.getType() == ZonedDateTime.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else if (source.getType() == short.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else if (source.getType() == Boolean.class) {
return (Setter) (array, arrayIndex, destIndex) -> source.set(destIndex, array[arrayIndex]);
} else {
return (Setter
© 2015 - 2024 Weber Informatics LLC | Privacy Policy