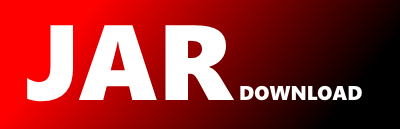
io.deephaven.engine.table.impl.sources.regioned.RegionedColumnSourceByte Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
/*
* ---------------------------------------------------------------------------------------------------------------------
* AUTO-GENERATED CLASS - DO NOT EDIT MANUALLY - for any changes edit RegionedColumnSourceChar and regenerate
* ---------------------------------------------------------------------------------------------------------------------
*/
package io.deephaven.engine.table.impl.sources.regioned;
import io.deephaven.engine.table.ColumnSource;
import io.deephaven.engine.rowset.RowSequence;
import io.deephaven.engine.table.ColumnDefinition;
import io.deephaven.engine.table.impl.locations.ColumnLocation;
import io.deephaven.engine.table.impl.locations.TableDataException;
import io.deephaven.engine.table.impl.locations.TableLocationKey;
import io.deephaven.engine.table.impl.ColumnSourceGetDefaults;
import io.deephaven.chunk.attributes.Values;
import org.jetbrains.annotations.NotNull;
import static io.deephaven.util.type.TypeUtils.unbox;
/**
* Regioned column source implementation for columns of bytes.
*/
abstract class RegionedColumnSourceByte
extends RegionedColumnSourceArray>
implements ColumnSourceGetDefaults.ForByte /* MIXIN_INTERFACES */ {
RegionedColumnSourceByte(@NotNull final ColumnRegionByte nullRegion,
@NotNull final MakeDeferred> makeDeferred) {
super(nullRegion, byte.class, makeDeferred);
}
@Override
public byte getByte(final long rowKey) {
return (rowKey == RowSequence.NULL_ROW_KEY ? getNullRegion() : lookupRegion(rowKey)).getByte(rowKey);
}
interface MakeRegionDefault extends MakeRegion> {
@Override
default ColumnRegionByte makeRegion(@NotNull final ColumnDefinition> columnDefinition,
@NotNull final ColumnLocation columnLocation,
final int regionIndex) {
if (columnLocation.exists()) {
return columnLocation.makeColumnRegionByte(columnDefinition);
}
return null;
}
}
// region reinterpretation
@Override
public boolean allowsReinterpret(@NotNull Class alternateDataType) {
return alternateDataType == boolean.class || alternateDataType == Boolean.class || super.allowsReinterpret(alternateDataType);
}
@Override
protected ColumnSource doReinterpret(@NotNull Class alternateDataType) {
//noinspection unchecked
return (ColumnSource) new RegionedColumnSourceBoolean((RegionedColumnSourceByte)this);
}
// endregion reinterpretation
static final class AsValues extends RegionedColumnSourceByte implements MakeRegionDefault {
AsValues() {
super(ColumnRegionByte.createNull(PARAMETERS.regionMask), DeferredColumnRegionByte::new);
}
}
static final class Partitioning extends RegionedColumnSourceByte {
Partitioning() {
super(ColumnRegionByte.createNull(PARAMETERS.regionMask),
(pm, rs) -> rs.get() // No need to interpose a deferred region in this case
);
}
@Override
public ColumnRegionByte makeRegion(@NotNull final ColumnDefinition> columnDefinition,
@NotNull final ColumnLocation columnLocation,
final int regionIndex) {
final TableLocationKey locationKey = columnLocation.getTableLocation().getKey();
final Object partitioningColumnValue = locationKey.getPartitionValue(columnDefinition.getName());
if (partitioningColumnValue != null && !Byte.class.isAssignableFrom(partitioningColumnValue.getClass())) {
throw new TableDataException("Unexpected partitioning column value type for " + columnDefinition.getName()
+ ": " + partitioningColumnValue + " is not a Byte at location " + locationKey);
}
return new ColumnRegionByte.Constant<>(regionMask(), unbox((Byte) partitioningColumnValue));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy