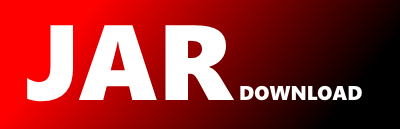
io.deephaven.engine.util.PythonScope Maven / Gradle / Ivy
Show all versions of deephaven-engine-table Show documentation
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.util;
import org.jpy.PyDictWrapper;
import org.jpy.PyObject;
import java.util.AbstractMap.SimpleImmutableEntry;
import java.util.Collection;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Optional;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* A collection of methods around retrieving objects from the given Python scope.
*
* The scope is likely coming from some sort of Python dictionary. The scope might be local, global, or other.
*
* @param the implementation's raw Python object type
*/
public interface PythonScope {
/**
* Retrieves a value from the given scope.
*
* No conversion is done.
*
* @param name the name of the python variable
* @return the value, or empty
*/
Optional getValueRaw(String name);
/**
* Retrieves all keys from the give scope.
*
* No conversion is done.
*
* Technically, the keys can be tuples...
*
* @return the keys
*/
Stream getKeysRaw();
/**
* Retrieves all keys and values from the given scope.
*
* No conversion is done.
*
* @return the keys and values
*/
Stream> getEntriesRaw();
/**
* The helper method to turn a raw key into a string key.
*
* Note: this assumes that all the keys are strings, which is not always true. Indices can also be tuples. TODO:
* revise interface as appropriate if this becomes an issue.
*
* @param key the raw key
* @return the string key
* @throws IllegalArgumentException if the key is not a string
*/
String convertStringKey(PyObj key);
/**
* The helper method to turn a raw value into an implementation specific object.
*
* This method should NOT convert PyObj of None type to null - we need to preserve the None object so it works with
* other Optional return values.
*
* @param value the raw value
* @return the converted object value
*/
Object convertValue(PyObj value);
/**
* Finds out if a variable is in scope
*
* @param name the name of the python variable
* @return true iff the scope contains the variable
*/
default boolean containsKey(String name) {
return getValueRaw(name).isPresent();
}
/**
* Equivalent to {@link #getValueRaw(String)}.map({@link #convertValue(Object)})
*
* @param name the name of the python variable
* @return the converted object value, or empty
*/
default Optional