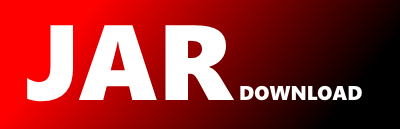
io.deephaven.engine.util.ScriptSession Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.util;
import io.deephaven.engine.context.ExecutionContext;
import io.deephaven.engine.context.QueryScope;
import io.deephaven.engine.liveness.LivenessNode;
import io.deephaven.engine.liveness.ReleasableLivenessManager;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
import java.nio.file.Path;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Set;
/**
* Interface for interactive console script sessions.
*/
public interface ScriptSession extends ReleasableLivenessManager, LivenessNode {
/**
* Retrieve a variable from the script session's bindings.
*
* Please use {@link ScriptSession#getVariable(String, Object)} if you expect the variable may not exist.
*
* @param name the variable to retrieve
* @return the variable
* @throws QueryScope.MissingVariableException if the variable does not exist
*/
@NotNull
Object getVariable(String name) throws QueryScope.MissingVariableException;
/**
* Retrieve a variable from the script session's bindings. If the variable is not present, return defaultValue.
*
* If the variable is present, but is not of type (T), a ClassCastException may result.
*
* @param name the variable to retrieve
* @param defaultValue the value to use when no value is present in the session's scope
* @param the type of the variable
* @return the value of the variable, or defaultValue if not present
*/
T getVariable(String name, T defaultValue);
/**
* A {@link VariableProvider} instance, for services like autocomplete which may want a limited "just the variables"
* view of our session state.
*
* @return a VariableProvider instance backed by the global/binding context of this script session.
*/
VariableProvider getVariableProvider();
/**
* Obtain an {@link ExecutionContext} instance for the current script session. This is the execution context that is
* used when executing scripts.
*/
ExecutionContext getExecutionContext();
class Changes {
public RuntimeException error = null;
// TODO(deephaven-core#1781): Close gaps between proto "CustomType" fields
public Map created = new LinkedHashMap<>();
public Map updated = new LinkedHashMap<>();
public Map removed = new LinkedHashMap<>();
public boolean isEmpty() {
return error == null && created.isEmpty() && updated.isEmpty() && removed.isEmpty();
}
public void throwIfError() {
if (error != null) {
throw error;
}
}
}
interface Listener {
void onScopeChanges(ScriptSession scriptSession, Changes changes);
}
/**
* Observe (and report via {@link Listener#onScopeChanges(ScriptSession, Changes) onScopeChanges}) any changes to
* this ScriptSession's {@link QueryScope} that may have been made externally, rather than during
* {@link #evaluateScript script evaluation}.
*
* @apiNote This method should be regarded as an unstable API
*/
void observeScopeChanges();
/**
* Evaluates the script and manages liveness of objects that are exported to the user. This method should be called
* from the serial executor as it manipulates static state.
*
* @param script the code to execute
* @return the changes made to the exportable objects
*/
default Changes evaluateScript(String script) {
return evaluateScript(script, null);
}
/**
* Evaluates the script and manages liveness of objects that are exported to the user. This method should be called
* from the serial executor as it manipulates static state.
*
* @param script the code to execute
* @param scriptName an optional script name, which may be ignored by the implementation, or used improve error
* messages or for other internal purposes
* @return the changes made to the exportable objects
*/
Changes evaluateScript(String script, @Nullable String scriptName);
/**
* Evaluates the script and manages liveness of objects that are exported to the user. This method should be called
* from the serial executor as it manipulates static state.
*
* @param scriptPath the path to the script to execute
* @return the changes made to the exportable objects
*/
Changes evaluateScript(Path scriptPath);
/**
* Retrieves all of the variables present in the session's scope (e.g., Groovy binding, Python globals()).
*
* @return an unmodifiable map with variable names as the keys, and the Objects as the result
*/
Map getVariables();
/**
* Retrieves all of the variable names present in the session's scope
*
* @return an unmodifiable set of variable names
*/
Set getVariableNames();
/**
* Check if the scope has the given variable name
*
* @param name the variable name
* @return True iff the scope has the given variable name
*/
boolean hasVariableName(String name);
/**
* Inserts a value into the script's scope.
*
* @param name the variable name to set
* @param value the new value of the variable
*/
void setVariable(String name, @Nullable Object value);
/**
* @return a textual description of this script session's language for use in messages.
*/
String scriptType();
/**
* If this script session can throw unserializable exceptions, this method is responsible for turning those
* exceptions into something suitable for sending back to a client.
*
* @param e the exception to (possibly) sanitize
* @return the sanitized exception
*/
default Throwable sanitizeThrowable(Throwable e) {
return e;
}
/**
* Asks the session to remove any wrapping that exists on scoped objects so that clients can fetch them. Defaults to
* returning the object itself.
*
* @param object the scoped object
* @return an obj which can be consumed by a client
*/
default Object unwrapObject(Object object) {
return object;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy