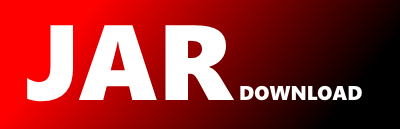
io.deephaven.engine.table.impl.SortingOrder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.table.impl;
import org.jetbrains.annotations.NotNull;
import java.util.Comparator;
/**
* Enum value for ascending vs. descending sorts.
*/
public enum SortingOrder {
Ascending(1, getAscendingComparable()), Descending(-1, getDescendingComparator());
public final int direction;
private final Comparator comparator;
SortingOrder(int direction, Comparator comparator) {
this.direction = direction;
this.comparator = comparator;
}
public int getDirection() {
return direction;
}
public Comparator getComparator() {
return comparator;
}
public boolean isAscending() {
return direction == 1;
}
public boolean isDescending() {
return direction == -1;
}
@NotNull
private static Comparator getAscendingComparable() {
return (o1, o2) -> {
if (o1 == o2) {
return 0;
} else if (o1 == null) {
return -1;
} else if (o2 == null) {
return 1;
}
// noinspection unchecked
return o1.compareTo(o2);
};
}
@NotNull
private static Comparator getDescendingComparator() {
return (o1, o2) -> {
if (o1 == o2) {
return 0;
} else if (o1 == null) {
return 1;
} else if (o2 == null) {
return -1;
}
// noinspection unchecked
return -o1.compareTo(o2);
};
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy