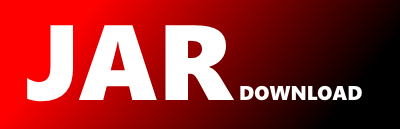
io.deephaven.engine.table.impl.sources.regioned.RegionedTableComponentFactoryImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.table.impl.sources.regioned;
import io.deephaven.engine.table.ColumnDefinition;
import io.deephaven.engine.table.impl.locations.TableDataException;
import io.deephaven.engine.table.impl.ColumnSourceManager;
import io.deephaven.engine.table.impl.ColumnToCodecMappings;
import io.deephaven.util.type.TypeUtils;
import org.jetbrains.annotations.NotNull;
import java.time.Instant;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.function.Supplier;
/**
* Factory that assembles modular components for regioned source tables.
*/
public class RegionedTableComponentFactoryImpl implements RegionedTableComponentFactory {
private static final Map, Supplier>> SIMPLE_DATA_TYPE_TO_REGIONED_COLUMN_SOURCE_SUPPLIER;
static {
Map, Supplier>> typeToSupplier = new HashMap<>();
typeToSupplier.put(Byte.class, RegionedColumnSourceByte.AsValues::new);
typeToSupplier.put(Character.class, RegionedColumnSourceChar.AsValues::new);
typeToSupplier.put(Double.class, RegionedColumnSourceDouble.AsValues::new);
typeToSupplier.put(Float.class, RegionedColumnSourceFloat.AsValues::new);
typeToSupplier.put(Integer.class, RegionedColumnSourceInt.AsValues::new);
typeToSupplier.put(Long.class, RegionedColumnSourceLong.AsValues::new);
typeToSupplier.put(Short.class, RegionedColumnSourceShort.AsValues::new);
typeToSupplier.put(Boolean.class, RegionedColumnSourceBoolean::new);
typeToSupplier.put(Instant.class, RegionedColumnSourceInstant::new);
SIMPLE_DATA_TYPE_TO_REGIONED_COLUMN_SOURCE_SUPPLIER = Collections.unmodifiableMap(typeToSupplier);
}
public static final RegionedTableComponentFactory INSTANCE = new RegionedTableComponentFactoryImpl();
private RegionedTableComponentFactoryImpl() {}
@Override
public ColumnSourceManager createColumnSourceManager(
final boolean isRefreshing,
@NotNull final ColumnToCodecMappings codecMappings,
@NotNull final List> columnDefinitions) {
return new RegionedColumnSourceManager(isRefreshing, this, codecMappings, columnDefinitions);
}
/**
* Create a new {@link RegionedColumnSource} appropriate to implement the supplied {@link ColumnDefinition}.
*
* @param columnDefinition The column definition
* @param The data type of the column
* @return A new RegionedColumnSource.
*/
@SuppressWarnings("unchecked")
@Override
public RegionedColumnSource createRegionedColumnSource(
@NotNull final ColumnDefinition columnDefinition,
@NotNull final ColumnToCodecMappings codecMappings) {
Class dataType = (Class) TypeUtils.getBoxedType(columnDefinition.getDataType());
if (columnDefinition.isPartitioning()) {
return PartitioningSourceFactory.makePartitioningSource(dataType);
}
final Supplier> simpleImplementationSupplier =
SIMPLE_DATA_TYPE_TO_REGIONED_COLUMN_SOURCE_SUPPLIER.get(dataType);
if (simpleImplementationSupplier != null) {
return (RegionedColumnSource) simpleImplementationSupplier.get();
}
try {
if (CharSequence.class.isAssignableFrom(dataType)) {
return new RegionedColumnSourceWithDictionary<>(dataType, null);
} else {
return new RegionedColumnSourceObject.AsValues<>(dataType, columnDefinition.getComponentType());
}
} catch (IllegalArgumentException except) {
throw new TableDataException(
"Can't create column for " + dataType + " in column definition " + columnDefinition, except);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy