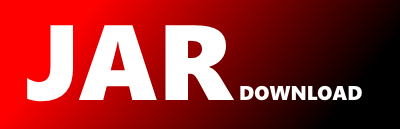
io.deephaven.engine.table.impl.sources.regioned.decoder.SimpleStringDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-engine-table Show documentation
Show all versions of deephaven-engine-table Show documentation
Engine Table: Implementation and closely-coupled utilities
/**
* Copyright (c) 2016-2022 Deephaven Data Labs and Patent Pending
*/
package io.deephaven.engine.table.impl.sources.regioned.decoder;
import io.deephaven.base.string.cache.ByteArrayCharSequenceAdapterImpl;
import io.deephaven.base.string.cache.StringCache;
import io.deephaven.engine.util.string.StringUtils;
import io.deephaven.util.codec.ObjectDecoder;
import org.jetbrains.annotations.NotNull;
public class SimpleStringDecoder implements ObjectDecoder {
private static final ThreadLocal DECODER_ADAPTER =
ThreadLocal.withInitial(ByteArrayCharSequenceAdapterImpl::new);
private final StringCache cache;
public SimpleStringDecoder(Class dataType) {
this.cache = StringUtils.getStringCache(dataType);
}
public SimpleStringDecoder(StringCache cache) {
this.cache = cache;
}
@Override
public final int expectedObjectWidth() {
return VARIABLE_WIDTH_SENTINEL;
}
@Override
public final STRING_LIKE_TYPE decode(@NotNull final byte[] data, final int offset, final int length) {
if (length == 0) {
return null;
}
if (length == 1 && data[offset] == 0) {
return cache.getEmptyString();
}
// NB: Because the StringCache implementations in use convert bytes to chars 1:1 (with a 0xFF mask), we're
// effectively using an ISO-8859-1 decoder.
// We could probably move towards StringCaches with configurable Charsets for encoding/decoding directly
// to/from ByteBuffers, but that's a step for later.
final ByteArrayCharSequenceAdapterImpl adapter = DECODER_ADAPTER.get();
final STRING_LIKE_TYPE result = cache.getCachedString(adapter.set(data, offset, length));
adapter.clear();
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy