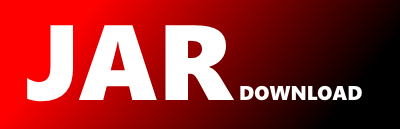
io.deephaven.tuple.ArrayTuple Maven / Gradle / Ivy
Show all versions of deephaven-engine-tuple Show documentation
//
// Copyright (c) 2016-2024 Deephaven Data Labs and Patent Pending
//
package io.deephaven.tuple;
import io.deephaven.tuple.serialization.SerializationUtils;
import io.deephaven.tuple.serialization.StreamingExternalizable;
import gnu.trove.map.TIntObjectMap;
import io.deephaven.util.compare.ObjectComparisons;
import io.deephaven.util.type.ArrayTypeUtils;
import org.jetbrains.annotations.NotNull;
import java.io.*;
import java.util.Arrays;
import java.util.function.UnaryOperator;
import java.util.stream.Stream;
/**
*
* N-Tuple key class backed by an array of elements.
*/
public class ArrayTuple
implements Comparable, Externalizable, StreamingExternalizable, CanonicalizableTuple {
private static final long serialVersionUID = 1L;
private Object[] elements;
private transient int cachedHashCode;
/**
* Construct a tuple backed by the supplied array of elements. The elements array should not be changed after this
* call.
*
* @param elements The array to wrap
*/
public ArrayTuple(final Object... elements) {
initialize(elements == null ? ArrayTypeUtils.EMPTY_OBJECT_ARRAY : elements);
}
/**
* This is required for {@link Externalizable} support, but should not be used otherwise.
*/
public ArrayTuple() {}
private void initialize(@NotNull final Object[] elements) {
this.elements = elements;
cachedHashCode = Arrays.hashCode(elements);
}
@SuppressWarnings("unused")
public final T getElement(final int elementIndex) {
// noinspection unchecked
return (T) elements[elementIndex];
}
/**
* Return a new array of the elements of this tuple, safe for any use.
*
* @return A new array of the elements of this tuple
*/
public final Object[] getElements() {
final Object[] exportedElements = new Object[elements.length];
System.arraycopy(elements, 0, exportedElements, 0, elements.length);
return exportedElements;
}
/**
* Get the elements of this tuple as a {@link Stream}.
*
* @return A new {@link Stream} of the elements of this tuple
*/
public Stream