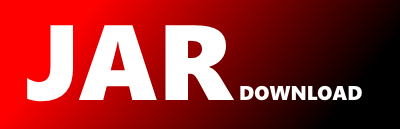
io.deephaven.tuple.generated.LongLongTuple Maven / Gradle / Ivy
Show all versions of deephaven-engine-tuple Show documentation
package io.deephaven.tuple.generated;
import gnu.trove.map.TIntObjectMap;
import io.deephaven.tuple.CanonicalizableTuple;
import io.deephaven.tuple.serialization.SerializationUtils;
import io.deephaven.tuple.serialization.StreamingExternalizable;
import io.deephaven.util.compare.LongComparisons;
import org.jetbrains.annotations.NotNull;
import java.io.Externalizable;
import java.io.IOException;
import java.io.ObjectInput;
import java.io.ObjectOutput;
import java.util.function.UnaryOperator;
/**
* 2-Tuple (double) key class composed of long and long elements.
*
Generated by io.deephaven.replicators.TupleCodeGenerator.
*/
public class LongLongTuple implements Comparable, Externalizable, StreamingExternalizable, CanonicalizableTuple {
private static final long serialVersionUID = 1L;
private long element1;
private long element2;
private transient int cachedHashCode;
public LongLongTuple(
final long element1,
final long element2
) {
initialize(
element1,
element2
);
}
/** Public no-arg constructor for {@link Externalizable} support only. Application code should not use this! **/
public LongLongTuple() {
}
private void initialize(
final long element1,
final long element2
) {
this.element1 = element1;
this.element2 = element2;
cachedHashCode = (31 +
Long.hashCode(element1)) * 31 +
Long.hashCode(element2);
}
public final long getFirstElement() {
return element1;
}
public final long getSecondElement() {
return element2;
}
@Override
public final int hashCode() {
return cachedHashCode;
}
@Override
public final boolean equals(final Object other) {
if (this == other) {
return true;
}
if (other == null || getClass() != other.getClass()) {
return false;
}
final LongLongTuple typedOther = (LongLongTuple) other;
// @formatter:off
return element1 == typedOther.element1 &&
element2 == typedOther.element2;
// @formatter:on
}
@Override
public final int compareTo(@NotNull final LongLongTuple other) {
if (this == other) {
return 0;
}
int comparison;
// @formatter:off
return 0 != (comparison = LongComparisons.compare(element1, other.element1)) ? comparison :
LongComparisons.compare(element2, other.element2);
// @formatter:on
}
@Override
public void writeExternal(@NotNull final ObjectOutput out) throws IOException {
out.writeLong(element1);
out.writeLong(element2);
}
@Override
public void readExternal(@NotNull final ObjectInput in) throws IOException, ClassNotFoundException {
initialize(
in.readLong(),
in.readLong()
);
}
@Override
public void writeExternalStreaming(@NotNull final ObjectOutput out, @NotNull final TIntObjectMap cachedWriters) throws IOException {
out.writeLong(element1);
out.writeLong(element2);
}
@Override
public void readExternalStreaming(@NotNull final ObjectInput in, @NotNull final TIntObjectMap cachedReaders) throws Exception {
initialize(
in.readLong(),
in.readLong()
);
}
@Override
public String toString() {
return "LongLongTuple{" +
element1 + ", " +
element2 + '}';
}
@Override
public LongLongTuple canonicalize(@NotNull final UnaryOperator