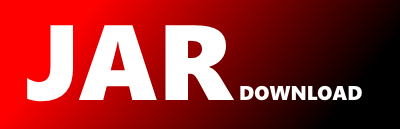
grpc.io.deephaven.proto.backplane.grpc.ApplicationServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-proto-backplane-grpc Show documentation
Show all versions of deephaven-proto-backplane-grpc Show documentation
The Deephaven proto-backplane-grpc
package io.deephaven.proto.backplane.grpc;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* Allows clients to list fields that are accessible to them.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.63.1)",
comments = "Source: deephaven/proto/application.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class ApplicationServiceGrpc {
private ApplicationServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "io.deephaven.proto.backplane.grpc.ApplicationService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getListFieldsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListFields",
requestType = io.deephaven.proto.backplane.grpc.ListFieldsRequest.class,
responseType = io.deephaven.proto.backplane.grpc.FieldsChangeUpdate.class,
methodType = io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
public static io.grpc.MethodDescriptor getListFieldsMethod() {
io.grpc.MethodDescriptor getListFieldsMethod;
if ((getListFieldsMethod = ApplicationServiceGrpc.getListFieldsMethod) == null) {
synchronized (ApplicationServiceGrpc.class) {
if ((getListFieldsMethod = ApplicationServiceGrpc.getListFieldsMethod) == null) {
ApplicationServiceGrpc.getListFieldsMethod = getListFieldsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.SERVER_STREAMING)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListFields"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.deephaven.proto.backplane.grpc.ListFieldsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.deephaven.proto.backplane.grpc.FieldsChangeUpdate.getDefaultInstance()))
.setSchemaDescriptor(new ApplicationServiceMethodDescriptorSupplier("ListFields"))
.build();
}
}
}
return getListFieldsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static ApplicationServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ApplicationServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ApplicationServiceStub(channel, callOptions);
}
};
return ApplicationServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static ApplicationServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ApplicationServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ApplicationServiceBlockingStub(channel, callOptions);
}
};
return ApplicationServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static ApplicationServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public ApplicationServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ApplicationServiceFutureStub(channel, callOptions);
}
};
return ApplicationServiceFutureStub.newStub(factory, channel);
}
/**
*
* Allows clients to list fields that are accessible to them.
*
*/
public interface AsyncService {
/**
*
* Request the list of the fields exposed via the worker.
* - The first received message contains all fields that are currently available
* on the worker. None of these fields will be RemovedFields.
* - Subsequent messages modify the existing state. Fields are identified by
* their ticket and may be replaced or removed.
*
*/
default void listFields(io.deephaven.proto.backplane.grpc.ListFieldsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListFieldsMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service ApplicationService.
*
* Allows clients to list fields that are accessible to them.
*
*/
public static abstract class ApplicationServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return ApplicationServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service ApplicationService.
*
* Allows clients to list fields that are accessible to them.
*
*/
public static final class ApplicationServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private ApplicationServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ApplicationServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ApplicationServiceStub(channel, callOptions);
}
/**
*
* Request the list of the fields exposed via the worker.
* - The first received message contains all fields that are currently available
* on the worker. None of these fields will be RemovedFields.
* - Subsequent messages modify the existing state. Fields are identified by
* their ticket and may be replaced or removed.
*
*/
public void listFields(io.deephaven.proto.backplane.grpc.ListFieldsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncServerStreamingCall(
getChannel().newCall(getListFieldsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service ApplicationService.
*
* Allows clients to list fields that are accessible to them.
*
*/
public static final class ApplicationServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private ApplicationServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ApplicationServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ApplicationServiceBlockingStub(channel, callOptions);
}
/**
*
* Request the list of the fields exposed via the worker.
* - The first received message contains all fields that are currently available
* on the worker. None of these fields will be RemovedFields.
* - Subsequent messages modify the existing state. Fields are identified by
* their ticket and may be replaced or removed.
*
*/
public java.util.Iterator listFields(
io.deephaven.proto.backplane.grpc.ListFieldsRequest request) {
return io.grpc.stub.ClientCalls.blockingServerStreamingCall(
getChannel(), getListFieldsMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service ApplicationService.
*
* Allows clients to list fields that are accessible to them.
*
*/
public static final class ApplicationServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private ApplicationServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected ApplicationServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new ApplicationServiceFutureStub(channel, callOptions);
}
}
private static final int METHODID_LIST_FIELDS = 0;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_LIST_FIELDS:
serviceImpl.listFields((io.deephaven.proto.backplane.grpc.ListFieldsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getListFieldsMethod(),
io.grpc.stub.ServerCalls.asyncServerStreamingCall(
new MethodHandlers<
io.deephaven.proto.backplane.grpc.ListFieldsRequest,
io.deephaven.proto.backplane.grpc.FieldsChangeUpdate>(
service, METHODID_LIST_FIELDS)))
.build();
}
private static abstract class ApplicationServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
ApplicationServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.deephaven.proto.backplane.grpc.Application.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("ApplicationService");
}
}
private static final class ApplicationServiceFileDescriptorSupplier
extends ApplicationServiceBaseDescriptorSupplier {
ApplicationServiceFileDescriptorSupplier() {}
}
private static final class ApplicationServiceMethodDescriptorSupplier
extends ApplicationServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
ApplicationServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (ApplicationServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new ApplicationServiceFileDescriptorSupplier())
.addMethod(getListFieldsMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy