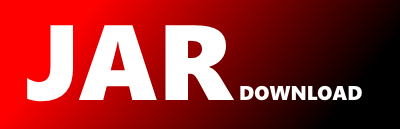
grpc.io.deephaven.proto.backplane.grpc.InputTableServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of deephaven-proto-backplane-grpc Show documentation
Show all versions of deephaven-proto-backplane-grpc Show documentation
The Deephaven proto-backplane-grpc
The newest version!
package io.deephaven.proto.backplane.grpc;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*
* This service offers methods to manipulate the contents of input tables.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.63.1)",
comments = "Source: deephaven_core/proto/inputtable.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class InputTableServiceGrpc {
private InputTableServiceGrpc() {}
public static final java.lang.String SERVICE_NAME = "io.deephaven.proto.backplane.grpc.InputTableService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getAddTableToInputTableMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "AddTableToInputTable",
requestType = io.deephaven.proto.backplane.grpc.AddTableRequest.class,
responseType = io.deephaven.proto.backplane.grpc.AddTableResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getAddTableToInputTableMethod() {
io.grpc.MethodDescriptor getAddTableToInputTableMethod;
if ((getAddTableToInputTableMethod = InputTableServiceGrpc.getAddTableToInputTableMethod) == null) {
synchronized (InputTableServiceGrpc.class) {
if ((getAddTableToInputTableMethod = InputTableServiceGrpc.getAddTableToInputTableMethod) == null) {
InputTableServiceGrpc.getAddTableToInputTableMethod = getAddTableToInputTableMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "AddTableToInputTable"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.deephaven.proto.backplane.grpc.AddTableRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.deephaven.proto.backplane.grpc.AddTableResponse.getDefaultInstance()))
.setSchemaDescriptor(new InputTableServiceMethodDescriptorSupplier("AddTableToInputTable"))
.build();
}
}
}
return getAddTableToInputTableMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteTableFromInputTableMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "DeleteTableFromInputTable",
requestType = io.deephaven.proto.backplane.grpc.DeleteTableRequest.class,
responseType = io.deephaven.proto.backplane.grpc.DeleteTableResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteTableFromInputTableMethod() {
io.grpc.MethodDescriptor getDeleteTableFromInputTableMethod;
if ((getDeleteTableFromInputTableMethod = InputTableServiceGrpc.getDeleteTableFromInputTableMethod) == null) {
synchronized (InputTableServiceGrpc.class) {
if ((getDeleteTableFromInputTableMethod = InputTableServiceGrpc.getDeleteTableFromInputTableMethod) == null) {
InputTableServiceGrpc.getDeleteTableFromInputTableMethod = getDeleteTableFromInputTableMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "DeleteTableFromInputTable"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.deephaven.proto.backplane.grpc.DeleteTableRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
io.deephaven.proto.backplane.grpc.DeleteTableResponse.getDefaultInstance()))
.setSchemaDescriptor(new InputTableServiceMethodDescriptorSupplier("DeleteTableFromInputTable"))
.build();
}
}
}
return getDeleteTableFromInputTableMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static InputTableServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public InputTableServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new InputTableServiceStub(channel, callOptions);
}
};
return InputTableServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static InputTableServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public InputTableServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new InputTableServiceBlockingStub(channel, callOptions);
}
};
return InputTableServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static InputTableServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public InputTableServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new InputTableServiceFutureStub(channel, callOptions);
}
};
return InputTableServiceFutureStub.newStub(factory, channel);
}
/**
*
* This service offers methods to manipulate the contents of input tables.
*
*/
public interface AsyncService {
/**
*
* Adds the provided table to the specified input table. The new data to add must only have
* columns (name, types, and order) which match the given input table's columns.
*
*/
default void addTableToInputTable(io.deephaven.proto.backplane.grpc.AddTableRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getAddTableToInputTableMethod(), responseObserver);
}
/**
*
* Removes the provided table from the specified input tables. The tables indicating which rows
* to remove are expected to only have columns that match the key columns of the input table.
*
*/
default void deleteTableFromInputTable(io.deephaven.proto.backplane.grpc.DeleteTableRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getDeleteTableFromInputTableMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service InputTableService.
*
* This service offers methods to manipulate the contents of input tables.
*
*/
public static abstract class InputTableServiceImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return InputTableServiceGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service InputTableService.
*
* This service offers methods to manipulate the contents of input tables.
*
*/
public static final class InputTableServiceStub
extends io.grpc.stub.AbstractAsyncStub {
private InputTableServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected InputTableServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new InputTableServiceStub(channel, callOptions);
}
/**
*
* Adds the provided table to the specified input table. The new data to add must only have
* columns (name, types, and order) which match the given input table's columns.
*
*/
public void addTableToInputTable(io.deephaven.proto.backplane.grpc.AddTableRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getAddTableToInputTableMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Removes the provided table from the specified input tables. The tables indicating which rows
* to remove are expected to only have columns that match the key columns of the input table.
*
*/
public void deleteTableFromInputTable(io.deephaven.proto.backplane.grpc.DeleteTableRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getDeleteTableFromInputTableMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service InputTableService.
*
* This service offers methods to manipulate the contents of input tables.
*
*/
public static final class InputTableServiceBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private InputTableServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected InputTableServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new InputTableServiceBlockingStub(channel, callOptions);
}
/**
*
* Adds the provided table to the specified input table. The new data to add must only have
* columns (name, types, and order) which match the given input table's columns.
*
*/
public io.deephaven.proto.backplane.grpc.AddTableResponse addTableToInputTable(io.deephaven.proto.backplane.grpc.AddTableRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getAddTableToInputTableMethod(), getCallOptions(), request);
}
/**
*
* Removes the provided table from the specified input tables. The tables indicating which rows
* to remove are expected to only have columns that match the key columns of the input table.
*
*/
public io.deephaven.proto.backplane.grpc.DeleteTableResponse deleteTableFromInputTable(io.deephaven.proto.backplane.grpc.DeleteTableRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getDeleteTableFromInputTableMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service InputTableService.
*
* This service offers methods to manipulate the contents of input tables.
*
*/
public static final class InputTableServiceFutureStub
extends io.grpc.stub.AbstractFutureStub {
private InputTableServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected InputTableServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new InputTableServiceFutureStub(channel, callOptions);
}
/**
*
* Adds the provided table to the specified input table. The new data to add must only have
* columns (name, types, and order) which match the given input table's columns.
*
*/
public com.google.common.util.concurrent.ListenableFuture addTableToInputTable(
io.deephaven.proto.backplane.grpc.AddTableRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getAddTableToInputTableMethod(), getCallOptions()), request);
}
/**
*
* Removes the provided table from the specified input tables. The tables indicating which rows
* to remove are expected to only have columns that match the key columns of the input table.
*
*/
public com.google.common.util.concurrent.ListenableFuture deleteTableFromInputTable(
io.deephaven.proto.backplane.grpc.DeleteTableRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getDeleteTableFromInputTableMethod(), getCallOptions()), request);
}
}
private static final int METHODID_ADD_TABLE_TO_INPUT_TABLE = 0;
private static final int METHODID_DELETE_TABLE_FROM_INPUT_TABLE = 1;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_ADD_TABLE_TO_INPUT_TABLE:
serviceImpl.addTableToInputTable((io.deephaven.proto.backplane.grpc.AddTableRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE_TABLE_FROM_INPUT_TABLE:
serviceImpl.deleteTableFromInputTable((io.deephaven.proto.backplane.grpc.DeleteTableRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getAddTableToInputTableMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.deephaven.proto.backplane.grpc.AddTableRequest,
io.deephaven.proto.backplane.grpc.AddTableResponse>(
service, METHODID_ADD_TABLE_TO_INPUT_TABLE)))
.addMethod(
getDeleteTableFromInputTableMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
io.deephaven.proto.backplane.grpc.DeleteTableRequest,
io.deephaven.proto.backplane.grpc.DeleteTableResponse>(
service, METHODID_DELETE_TABLE_FROM_INPUT_TABLE)))
.build();
}
private static abstract class InputTableServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
InputTableServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return io.deephaven.proto.backplane.grpc.Inputtable.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("InputTableService");
}
}
private static final class InputTableServiceFileDescriptorSupplier
extends InputTableServiceBaseDescriptorSupplier {
InputTableServiceFileDescriptorSupplier() {}
}
private static final class InputTableServiceMethodDescriptorSupplier
extends InputTableServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
InputTableServiceMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (InputTableServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new InputTableServiceFileDescriptorSupplier())
.addMethod(getAddTableToInputTableMethod())
.addMethod(getDeleteTableFromInputTableMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy