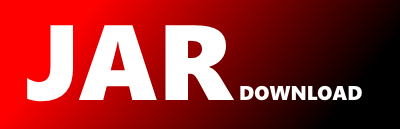
io.deepsense.neptune.apiclient.api.DefaultApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-api-client Show documentation
Show all versions of neptune-api-client Show documentation
Enables integration with Neptune in your Java code
/**
* Neptune API
* Neptune API
*
* OpenAPI spec version: 1.4_c9e4693-SNAPSHOT
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.deepsense.neptune.apiclient.api;
import io.deepsense.neptune.apiclient.ApiCallback;
import io.deepsense.neptune.apiclient.ApiClient;
import io.deepsense.neptune.apiclient.ApiException;
import io.deepsense.neptune.apiclient.ApiResponse;
import io.deepsense.neptune.apiclient.Configuration;
import io.deepsense.neptune.apiclient.Pair;
import io.deepsense.neptune.apiclient.ProgressRequestBody;
import io.deepsense.neptune.apiclient.ProgressResponseBody;
import com.google.gson.reflect.TypeToken;
import java.io.IOException;
import io.deepsense.neptune.apiclient.model.ActionInvocationParams;
import io.deepsense.neptune.apiclient.model.Error;
import io.deepsense.neptune.apiclient.model.ActionInvocationConfirmation;
import io.deepsense.neptune.apiclient.model.CompletedActionParams;
import io.deepsense.neptune.apiclient.model.Chart;
import io.deepsense.neptune.apiclient.model.ConfigInfo;
import io.deepsense.neptune.apiclient.model.FilterGroup;
import io.deepsense.neptune.apiclient.model.FilterGroupParams;
import io.deepsense.neptune.apiclient.model.TensorflowGraph;
import io.deepsense.neptune.apiclient.model.BatchJobUpdateResult;
import io.deepsense.neptune.apiclient.model.JobSimpleView;
import io.deepsense.neptune.apiclient.model.Job;
import io.deepsense.neptune.apiclient.model.ActionEvent;
import io.deepsense.neptune.apiclient.model.ActionInvocation;
import io.deepsense.neptune.apiclient.model.ChannelValues;
import io.deepsense.neptune.apiclient.model.BatchChannelValueError;
import io.deepsense.neptune.apiclient.model.CompletedJobParams;
import io.deepsense.neptune.apiclient.model.ExecutingJobParams;
import io.deepsense.neptune.apiclient.model.EditJobParams;
import io.deepsense.neptune.apiclient.model.QueuedJobParams;
import io.deepsense.neptune.apiclient.model.Quota;
import io.deepsense.neptune.apiclient.model.Status;
import io.deepsense.neptune.apiclient.model.User;
import io.deepsense.neptune.apiclient.model.Version;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class DefaultApi {
private ApiClient apiClient;
public DefaultApi() {
this(Configuration.getDefaultApiClient());
}
public DefaultApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/* Build call for actionsActionIdInvokePost */
private com.squareup.okhttp.Call actionsActionIdInvokePostCall(String jobId, String actionId, ActionInvocationParams invocationParams, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = invocationParams;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling actionsActionIdInvokePost(Async)");
}
// verify the required parameter 'actionId' is set
if (actionId == null) {
throw new ApiException("Missing the required parameter 'actionId' when calling actionsActionIdInvokePost(Async)");
}
// verify the required parameter 'invocationParams' is set
if (invocationParams == null) {
throw new ApiException("Missing the required parameter 'invocationParams' when calling actionsActionIdInvokePost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/actions/{actionId}/invoke".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()))
.replaceAll("\\{" + "actionId" + "\\}", apiClient.escapeString(actionId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param jobId (required)
* @param actionId (required)
* @param invocationParams (required)
* @return ActionInvocationConfirmation
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ActionInvocationConfirmation actionsActionIdInvokePost(String jobId, String actionId, ActionInvocationParams invocationParams) throws ApiException {
ApiResponse resp = actionsActionIdInvokePostWithHttpInfo(jobId, actionId, invocationParams);
return resp.getData();
}
/**
*
*
* @param jobId (required)
* @param actionId (required)
* @param invocationParams (required)
* @return ApiResponse<ActionInvocationConfirmation>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse actionsActionIdInvokePostWithHttpInfo(String jobId, String actionId, ActionInvocationParams invocationParams) throws ApiException {
com.squareup.okhttp.Call call = actionsActionIdInvokePostCall(jobId, actionId, invocationParams, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param jobId (required)
* @param actionId (required)
* @param invocationParams (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call actionsActionIdInvokePostAsync(String jobId, String actionId, ActionInvocationParams invocationParams, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = actionsActionIdInvokePostCall(jobId, actionId, invocationParams, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for actionsActionInvocationIdMarkCompletedPost */
private com.squareup.okhttp.Call actionsActionInvocationIdMarkCompletedPostCall(String jobId, String actionId, String actionInvocationId, CompletedActionParams completedActionParams, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = completedActionParams;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling actionsActionInvocationIdMarkCompletedPost(Async)");
}
// verify the required parameter 'actionId' is set
if (actionId == null) {
throw new ApiException("Missing the required parameter 'actionId' when calling actionsActionInvocationIdMarkCompletedPost(Async)");
}
// verify the required parameter 'actionInvocationId' is set
if (actionInvocationId == null) {
throw new ApiException("Missing the required parameter 'actionInvocationId' when calling actionsActionInvocationIdMarkCompletedPost(Async)");
}
// verify the required parameter 'completedActionParams' is set
if (completedActionParams == null) {
throw new ApiException("Missing the required parameter 'completedActionParams' when calling actionsActionInvocationIdMarkCompletedPost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/actions/{actionId}/invocations/{actionInvocationId}/markCompleted".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()))
.replaceAll("\\{" + "actionId" + "\\}", apiClient.escapeString(actionId.toString()))
.replaceAll("\\{" + "actionInvocationId" + "\\}", apiClient.escapeString(actionInvocationId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param jobId (required)
* @param actionId (required)
* @param actionInvocationId (required)
* @param completedActionParams (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void actionsActionInvocationIdMarkCompletedPost(String jobId, String actionId, String actionInvocationId, CompletedActionParams completedActionParams) throws ApiException {
actionsActionInvocationIdMarkCompletedPostWithHttpInfo(jobId, actionId, actionInvocationId, completedActionParams);
}
/**
*
*
* @param jobId (required)
* @param actionId (required)
* @param actionInvocationId (required)
* @param completedActionParams (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse actionsActionInvocationIdMarkCompletedPostWithHttpInfo(String jobId, String actionId, String actionInvocationId, CompletedActionParams completedActionParams) throws ApiException {
com.squareup.okhttp.Call call = actionsActionInvocationIdMarkCompletedPostCall(jobId, actionId, actionInvocationId, completedActionParams, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param jobId (required)
* @param actionId (required)
* @param actionInvocationId (required)
* @param completedActionParams (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call actionsActionInvocationIdMarkCompletedPostAsync(String jobId, String actionId, String actionInvocationId, CompletedActionParams completedActionParams, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = actionsActionInvocationIdMarkCompletedPostCall(jobId, actionId, actionInvocationId, completedActionParams, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for chartsChartIdDelete */
private com.squareup.okhttp.Call chartsChartIdDeleteCall(String jobId, String chartId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling chartsChartIdDelete(Async)");
}
// verify the required parameter 'chartId' is set
if (chartId == null) {
throw new ApiException("Missing the required parameter 'chartId' when calling chartsChartIdDelete(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/charts/{chartId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()))
.replaceAll("\\{" + "chartId" + "\\}", apiClient.escapeString(chartId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Delete the chart
* @param jobId (required)
* @param chartId (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void chartsChartIdDelete(String jobId, String chartId) throws ApiException {
chartsChartIdDeleteWithHttpInfo(jobId, chartId);
}
/**
*
* Delete the chart
* @param jobId (required)
* @param chartId (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse chartsChartIdDeleteWithHttpInfo(String jobId, String chartId) throws ApiException {
com.squareup.okhttp.Call call = chartsChartIdDeleteCall(jobId, chartId, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
* Delete the chart
* @param jobId (required)
* @param chartId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call chartsChartIdDeleteAsync(String jobId, String chartId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = chartsChartIdDeleteCall(jobId, chartId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for chartsChartIdGet */
private com.squareup.okhttp.Call chartsChartIdGetCall(String jobId, String chartId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling chartsChartIdGet(Async)");
}
// verify the required parameter 'chartId' is set
if (chartId == null) {
throw new ApiException("Missing the required parameter 'chartId' when calling chartsChartIdGet(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/charts/{chartId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()))
.replaceAll("\\{" + "chartId" + "\\}", apiClient.escapeString(chartId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Returns the chart with selected id
* @param jobId (required)
* @param chartId (required)
* @return Chart
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public Chart chartsChartIdGet(String jobId, String chartId) throws ApiException {
ApiResponse resp = chartsChartIdGetWithHttpInfo(jobId, chartId);
return resp.getData();
}
/**
*
* Returns the chart with selected id
* @param jobId (required)
* @param chartId (required)
* @return ApiResponse<Chart>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse chartsChartIdGetWithHttpInfo(String jobId, String chartId) throws ApiException {
com.squareup.okhttp.Call call = chartsChartIdGetCall(jobId, chartId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns the chart with selected id
* @param jobId (required)
* @param chartId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call chartsChartIdGetAsync(String jobId, String chartId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = chartsChartIdGetCall(jobId, chartId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for chartsChartIdPut */
private com.squareup.okhttp.Call chartsChartIdPutCall(String jobId, String chartId, Chart updatedChart, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = updatedChart;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling chartsChartIdPut(Async)");
}
// verify the required parameter 'chartId' is set
if (chartId == null) {
throw new ApiException("Missing the required parameter 'chartId' when calling chartsChartIdPut(Async)");
}
// verify the required parameter 'updatedChart' is set
if (updatedChart == null) {
throw new ApiException("Missing the required parameter 'updatedChart' when calling chartsChartIdPut(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/charts/{chartId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()))
.replaceAll("\\{" + "chartId" + "\\}", apiClient.escapeString(chartId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Update the chart
* @param jobId (required)
* @param chartId (required)
* @param updatedChart (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void chartsChartIdPut(String jobId, String chartId, Chart updatedChart) throws ApiException {
chartsChartIdPutWithHttpInfo(jobId, chartId, updatedChart);
}
/**
*
* Update the chart
* @param jobId (required)
* @param chartId (required)
* @param updatedChart (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse chartsChartIdPutWithHttpInfo(String jobId, String chartId, Chart updatedChart) throws ApiException {
com.squareup.okhttp.Call call = chartsChartIdPutCall(jobId, chartId, updatedChart, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
* Update the chart
* @param jobId (required)
* @param chartId (required)
* @param updatedChart (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call chartsChartIdPutAsync(String jobId, String chartId, Chart updatedChart, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = chartsChartIdPutCall(jobId, chartId, updatedChart, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for chartsGet */
private com.squareup.okhttp.Call chartsGetCall(String jobId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling chartsGet(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/charts".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Returns all charts related to the job
* @param jobId (required)
* @return List<Chart>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List chartsGet(String jobId) throws ApiException {
ApiResponse> resp = chartsGetWithHttpInfo(jobId);
return resp.getData();
}
/**
*
* Returns all charts related to the job
* @param jobId (required)
* @return ApiResponse<List<Chart>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> chartsGetWithHttpInfo(String jobId) throws ApiException {
com.squareup.okhttp.Call call = chartsGetCall(jobId, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns all charts related to the job
* @param jobId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call chartsGetAsync(String jobId, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = chartsGetCall(jobId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for chartsPost */
private com.squareup.okhttp.Call chartsPostCall(String jobId, Chart chartToCreate, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = chartToCreate;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling chartsPost(Async)");
}
// verify the required parameter 'chartToCreate' is set
if (chartToCreate == null) {
throw new ApiException("Missing the required parameter 'chartToCreate' when calling chartsPost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/charts".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Create a new chart
* @param jobId (required)
* @param chartToCreate (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void chartsPost(String jobId, Chart chartToCreate) throws ApiException {
chartsPostWithHttpInfo(jobId, chartToCreate);
}
/**
*
* Create a new chart
* @param jobId (required)
* @param chartToCreate (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse chartsPostWithHttpInfo(String jobId, Chart chartToCreate) throws ApiException {
com.squareup.okhttp.Call call = chartsPostCall(jobId, chartToCreate, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
* Create a new chart
* @param jobId (required)
* @param chartToCreate (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call chartsPostAsync(String jobId, Chart chartToCreate, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = chartsPostCall(jobId, chartToCreate, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for configInfoGet */
private com.squareup.okhttp.Call configInfoGetCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/1.4/default/configInfo".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @return ConfigInfo
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ConfigInfo configInfoGet() throws ApiException {
ApiResponse resp = configInfoGetWithHttpInfo();
return resp.getData();
}
/**
*
*
* @return ApiResponse<ConfigInfo>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse configInfoGetWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = configInfoGetCall(null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call configInfoGetAsync(final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = configInfoGetCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for filterGroupsGet */
private com.squareup.okhttp.Call filterGroupsGetCall(final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/1.4/default/filterGroups".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @return List<FilterGroup>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List filterGroupsGet() throws ApiException {
ApiResponse> resp = filterGroupsGetWithHttpInfo();
return resp.getData();
}
/**
*
*
* @return ApiResponse<List<FilterGroup>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> filterGroupsGetWithHttpInfo() throws ApiException {
com.squareup.okhttp.Call call = filterGroupsGetCall(null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call filterGroupsGetAsync(final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = filterGroupsGetCall(progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for filterGroupsGroupIdDelete */
private com.squareup.okhttp.Call filterGroupsGroupIdDeleteCall(String groupId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling filterGroupsGroupIdDelete(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/filterGroups/{groupId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "DELETE", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param groupId (required)
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public void filterGroupsGroupIdDelete(String groupId) throws ApiException {
filterGroupsGroupIdDeleteWithHttpInfo(groupId);
}
/**
*
*
* @param groupId (required)
* @return ApiResponse<Void>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse filterGroupsGroupIdDeleteWithHttpInfo(String groupId) throws ApiException {
com.squareup.okhttp.Call call = filterGroupsGroupIdDeleteCall(groupId, null, null);
return apiClient.execute(call);
}
/**
* (asynchronously)
*
* @param groupId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call filterGroupsGroupIdDeleteAsync(String groupId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = filterGroupsGroupIdDeleteCall(groupId, progressListener, progressRequestListener);
apiClient.executeAsync(call, callback);
return call;
}
/* Build call for filterGroupsGroupIdPut */
private com.squareup.okhttp.Call filterGroupsGroupIdPutCall(String groupId, FilterGroupParams filterGroupParams, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = filterGroupParams;
// verify the required parameter 'groupId' is set
if (groupId == null) {
throw new ApiException("Missing the required parameter 'groupId' when calling filterGroupsGroupIdPut(Async)");
}
// verify the required parameter 'filterGroupParams' is set
if (filterGroupParams == null) {
throw new ApiException("Missing the required parameter 'filterGroupParams' when calling filterGroupsGroupIdPut(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/filterGroups/{groupId}".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "groupId" + "\\}", apiClient.escapeString(groupId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "PUT", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Modifies selected fields of a filter group.
* @param groupId (required)
* @param filterGroupParams (required)
* @return FilterGroup
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public FilterGroup filterGroupsGroupIdPut(String groupId, FilterGroupParams filterGroupParams) throws ApiException {
ApiResponse resp = filterGroupsGroupIdPutWithHttpInfo(groupId, filterGroupParams);
return resp.getData();
}
/**
*
* Modifies selected fields of a filter group.
* @param groupId (required)
* @param filterGroupParams (required)
* @return ApiResponse<FilterGroup>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse filterGroupsGroupIdPutWithHttpInfo(String groupId, FilterGroupParams filterGroupParams) throws ApiException {
com.squareup.okhttp.Call call = filterGroupsGroupIdPutCall(groupId, filterGroupParams, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Modifies selected fields of a filter group.
* @param groupId (required)
* @param filterGroupParams (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call filterGroupsGroupIdPutAsync(String groupId, FilterGroupParams filterGroupParams, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = filterGroupsGroupIdPutCall(groupId, filterGroupParams, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for filterGroupsPost */
private com.squareup.okhttp.Call filterGroupsPostCall(FilterGroupParams filterGroupParams, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = filterGroupParams;
// verify the required parameter 'filterGroupParams' is set
if (filterGroupParams == null) {
throw new ApiException("Missing the required parameter 'filterGroupParams' when calling filterGroupsPost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/filterGroups".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param filterGroupParams (required)
* @return FilterGroup
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public FilterGroup filterGroupsPost(FilterGroupParams filterGroupParams) throws ApiException {
ApiResponse resp = filterGroupsPostWithHttpInfo(filterGroupParams);
return resp.getData();
}
/**
*
*
* @param filterGroupParams (required)
* @return ApiResponse<FilterGroup>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse filterGroupsPostWithHttpInfo(FilterGroupParams filterGroupParams) throws ApiException {
com.squareup.okhttp.Call call = filterGroupsPostCall(filterGroupParams, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param filterGroupParams (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call filterGroupsPostAsync(FilterGroupParams filterGroupParams, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = filterGroupsPostCall(filterGroupParams, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for getTensorflowGraphs */
private com.squareup.okhttp.Call getTensorflowGraphsCall(String jobId, List ids, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling getTensorflowGraphs(Async)");
}
// verify the required parameter 'ids' is set
if (ids == null) {
throw new ApiException("Missing the required parameter 'ids' when calling getTensorflowGraphs(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/thirdPartyData/tensorflowGraph".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
if (ids != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("multi", "ids", ids));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Returns requested tensorflow graphs.
* @param jobId (required)
* @param ids (required)
* @return List<TensorflowGraph>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List getTensorflowGraphs(String jobId, List ids) throws ApiException {
ApiResponse> resp = getTensorflowGraphsWithHttpInfo(jobId, ids);
return resp.getData();
}
/**
*
* Returns requested tensorflow graphs.
* @param jobId (required)
* @param ids (required)
* @return ApiResponse<List<TensorflowGraph>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> getTensorflowGraphsWithHttpInfo(String jobId, List ids) throws ApiException {
com.squareup.okhttp.Call call = getTensorflowGraphsCall(jobId, ids, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns requested tensorflow graphs.
* @param jobId (required)
* @param ids (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call getTensorflowGraphsAsync(String jobId, List ids, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = getTensorflowGraphsCall(jobId, ids, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsAbortPost */
private com.squareup.okhttp.Call jobsAbortPostCall(List jobIds, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = jobIds;
// verify the required parameter 'jobIds' is set
if (jobIds == null) {
throw new ApiException("Missing the required parameter 'jobIds' when calling jobsAbortPost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/abort".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param jobIds (required)
* @return List<BatchJobUpdateResult>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List jobsAbortPost(List jobIds) throws ApiException {
ApiResponse> resp = jobsAbortPostWithHttpInfo(jobIds);
return resp.getData();
}
/**
*
*
* @param jobIds (required)
* @return ApiResponse<List<BatchJobUpdateResult>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> jobsAbortPostWithHttpInfo(List jobIds) throws ApiException {
com.squareup.okhttp.Call call = jobsAbortPostCall(jobIds, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param jobIds (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call jobsAbortPostAsync(List jobIds, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = jobsAbortPostCall(jobIds, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsArchivePost */
private com.squareup.okhttp.Call jobsArchivePostCall(List jobIds, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = jobIds;
// verify the required parameter 'jobIds' is set
if (jobIds == null) {
throw new ApiException("Missing the required parameter 'jobIds' when calling jobsArchivePost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/archive".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param jobIds (required)
* @return List<BatchJobUpdateResult>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List jobsArchivePost(List jobIds) throws ApiException {
ApiResponse> resp = jobsArchivePostWithHttpInfo(jobIds);
return resp.getData();
}
/**
*
*
* @param jobIds (required)
* @return ApiResponse<List<BatchJobUpdateResult>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> jobsArchivePostWithHttpInfo(List jobIds) throws ApiException {
com.squareup.okhttp.Call call = jobsArchivePostCall(jobIds, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param jobIds (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call jobsArchivePostAsync(List jobIds, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = jobsArchivePostCall(jobIds, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsGet */
private com.squareup.okhttp.Call jobsGetCall(Boolean trashed, Boolean archived, Boolean responding, List tags, List states, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// create path and map variables
String localVarPath = "/1.4/default/jobs".replaceAll("\\{format\\}","json");
List localVarQueryParams = new ArrayList();
if (trashed != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "trashed", trashed));
if (archived != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "archived", archived));
if (responding != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("", "responding", responding));
if (tags != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("multi", "tags", tags));
if (states != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("multi", "states", states));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param trashed Filters jobs by their 'trashed' property. The value corresponds to trashed property's value. (optional)
* @param archived Filters jobs by their 'archived' property. The value corresponds to archived property's value. (optional)
* @param responding Filters jobs by their 'responding' property. The value corresponds to responding property's value. (optional)
* @param tags (optional)
* @param states (optional)
* @return List<JobSimpleView>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List jobsGet(Boolean trashed, Boolean archived, Boolean responding, List tags, List states) throws ApiException {
ApiResponse> resp = jobsGetWithHttpInfo(trashed, archived, responding, tags, states);
return resp.getData();
}
/**
*
*
* @param trashed Filters jobs by their 'trashed' property. The value corresponds to trashed property's value. (optional)
* @param archived Filters jobs by their 'archived' property. The value corresponds to archived property's value. (optional)
* @param responding Filters jobs by their 'responding' property. The value corresponds to responding property's value. (optional)
* @param tags (optional)
* @param states (optional)
* @return ApiResponse<List<JobSimpleView>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> jobsGetWithHttpInfo(Boolean trashed, Boolean archived, Boolean responding, List tags, List states) throws ApiException {
com.squareup.okhttp.Call call = jobsGetCall(trashed, archived, responding, tags, states, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
*
* @param trashed Filters jobs by their 'trashed' property. The value corresponds to trashed property's value. (optional)
* @param archived Filters jobs by their 'archived' property. The value corresponds to archived property's value. (optional)
* @param responding Filters jobs by their 'responding' property. The value corresponds to responding property's value. (optional)
* @param tags (optional)
* @param states (optional)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call jobsGetAsync(Boolean trashed, Boolean archived, Boolean responding, List tags, List states, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = jobsGetCall(trashed, archived, responding, tags, states, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsJobIdAbortPost */
private com.squareup.okhttp.Call jobsJobIdAbortPostCall(String jobId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling jobsJobIdAbortPost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/abort".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Sends request to finish a job execution. If selected job is not running, an error is returned. Note that successful response does not guarantees that job was aborted.
* @param jobId (required)
* @return Job
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public Job jobsJobIdAbortPost(String jobId) throws ApiException {
ApiResponse resp = jobsJobIdAbortPostWithHttpInfo(jobId);
return resp.getData();
}
/**
*
* Sends request to finish a job execution. If selected job is not running, an error is returned. Note that successful response does not guarantees that job was aborted.
* @param jobId (required)
* @return ApiResponse<Job>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse jobsJobIdAbortPostWithHttpInfo(String jobId) throws ApiException {
com.squareup.okhttp.Call call = jobsJobIdAbortPostCall(jobId, null, null);
Type localVarReturnType = new TypeToken(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Sends request to finish a job execution. If selected job is not running, an error is returned. Note that successful response does not guarantees that job was aborted.
* @param jobId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call jobsJobIdAbortPostAsync(String jobId, final ApiCallback callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = jobsJobIdAbortPostCall(jobId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsJobIdActionEventsGet */
private com.squareup.okhttp.Call jobsJobIdActionEventsGetCall(String jobId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling jobsJobIdActionEventsGet(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/actionEvents".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Returns a history of action related events.
* @param jobId (required)
* @return List<ActionEvent>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List jobsJobIdActionEventsGet(String jobId) throws ApiException {
ApiResponse> resp = jobsJobIdActionEventsGetWithHttpInfo(jobId);
return resp.getData();
}
/**
*
* Returns a history of action related events.
* @param jobId (required)
* @return ApiResponse<List<ActionEvent>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> jobsJobIdActionEventsGetWithHttpInfo(String jobId) throws ApiException {
com.squareup.okhttp.Call call = jobsJobIdActionEventsGetCall(jobId, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns a history of action related events.
* @param jobId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call jobsJobIdActionEventsGetAsync(String jobId, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = jobsJobIdActionEventsGetCall(jobId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsJobIdActionInvocationsGet */
private com.squareup.okhttp.Call jobsJobIdActionInvocationsGetCall(String jobId, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling jobsJobIdActionInvocationsGet(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/actionInvocations".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Returns action invocation details.
* @param jobId (required)
* @return List<ActionInvocation>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List jobsJobIdActionInvocationsGet(String jobId) throws ApiException {
ApiResponse> resp = jobsJobIdActionInvocationsGetWithHttpInfo(jobId);
return resp.getData();
}
/**
*
* Returns action invocation details.
* @param jobId (required)
* @return ApiResponse<List<ActionInvocation>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> jobsJobIdActionInvocationsGetWithHttpInfo(String jobId) throws ApiException {
com.squareup.okhttp.Call call = jobsJobIdActionInvocationsGetCall(jobId, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns action invocation details.
* @param jobId (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call jobsJobIdActionInvocationsGetAsync(String jobId, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = jobsJobIdActionInvocationsGetCall(jobId, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsJobIdChannelsValuesGet */
private com.squareup.okhttp.Call jobsJobIdChannelsValuesGetCall(String jobId, List channelIds, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = null;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling jobsJobIdChannelsValuesGet(Async)");
}
// verify the required parameter 'channelIds' is set
if (channelIds == null) {
throw new ApiException("Missing the required parameter 'channelIds' when calling jobsJobIdChannelsValuesGet(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/channels/values".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
if (channelIds != null)
localVarQueryParams.addAll(apiClient.parameterToPairs("multi", "channelIds", channelIds));
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "GET", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
* Returns numeric and text channel values.
* @param jobId (required)
* @param channelIds (required)
* @return List<ChannelValues>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List jobsJobIdChannelsValuesGet(String jobId, List channelIds) throws ApiException {
ApiResponse> resp = jobsJobIdChannelsValuesGetWithHttpInfo(jobId, channelIds);
return resp.getData();
}
/**
*
* Returns numeric and text channel values.
* @param jobId (required)
* @param channelIds (required)
* @return ApiResponse<List<ChannelValues>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> jobsJobIdChannelsValuesGetWithHttpInfo(String jobId, List channelIds) throws ApiException {
com.squareup.okhttp.Call call = jobsJobIdChannelsValuesGetCall(jobId, channelIds, null, null);
Type localVarReturnType = new TypeToken>(){}.getType();
return apiClient.execute(call, localVarReturnType);
}
/**
* (asynchronously)
* Returns numeric and text channel values.
* @param jobId (required)
* @param channelIds (required)
* @param callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
*/
public com.squareup.okhttp.Call jobsJobIdChannelsValuesGetAsync(String jobId, List channelIds, final ApiCallback> callback) throws ApiException {
ProgressResponseBody.ProgressListener progressListener = null;
ProgressRequestBody.ProgressRequestListener progressRequestListener = null;
if (callback != null) {
progressListener = new ProgressResponseBody.ProgressListener() {
@Override
public void update(long bytesRead, long contentLength, boolean done) {
callback.onDownloadProgress(bytesRead, contentLength, done);
}
};
progressRequestListener = new ProgressRequestBody.ProgressRequestListener() {
@Override
public void onRequestProgress(long bytesWritten, long contentLength, boolean done) {
callback.onUploadProgress(bytesWritten, contentLength, done);
}
};
}
com.squareup.okhttp.Call call = jobsJobIdChannelsValuesGetCall(jobId, channelIds, progressListener, progressRequestListener);
Type localVarReturnType = new TypeToken>(){}.getType();
apiClient.executeAsync(call, localVarReturnType, callback);
return call;
}
/* Build call for jobsJobIdChannelsValuesPost */
private com.squareup.okhttp.Call jobsJobIdChannelsValuesPostCall(String jobId, List channelsValues, final ProgressResponseBody.ProgressListener progressListener, final ProgressRequestBody.ProgressRequestListener progressRequestListener) throws ApiException {
Object localVarPostBody = channelsValues;
// verify the required parameter 'jobId' is set
if (jobId == null) {
throw new ApiException("Missing the required parameter 'jobId' when calling jobsJobIdChannelsValuesPost(Async)");
}
// verify the required parameter 'channelsValues' is set
if (channelsValues == null) {
throw new ApiException("Missing the required parameter 'channelsValues' when calling jobsJobIdChannelsValuesPost(Async)");
}
// create path and map variables
String localVarPath = "/1.4/default/jobs/{jobId}/channels/values".replaceAll("\\{format\\}","json")
.replaceAll("\\{" + "jobId" + "\\}", apiClient.escapeString(jobId.toString()));
List localVarQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarFormParams = new HashMap();
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) localVarHeaderParams.put("Accept", localVarAccept);
final String[] localVarContentTypes = {
};
final String localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
localVarHeaderParams.put("Content-Type", localVarContentType);
if(progressListener != null) {
apiClient.getHttpClient().networkInterceptors().add(new com.squareup.okhttp.Interceptor() {
@Override
public com.squareup.okhttp.Response intercept(com.squareup.okhttp.Interceptor.Chain chain) throws IOException {
com.squareup.okhttp.Response originalResponse = chain.proceed(chain.request());
return originalResponse.newBuilder()
.body(new ProgressResponseBody(originalResponse.body(), progressListener))
.build();
}
});
}
String[] localVarAuthNames = new String[] { };
return apiClient.buildCall(localVarPath, "POST", localVarQueryParams, localVarPostBody, localVarHeaderParams, localVarFormParams, localVarAuthNames, progressRequestListener);
}
/**
*
*
* @param jobId (required)
* @param channelsValues (required)
* @return List<BatchChannelValueError>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public List jobsJobIdChannelsValuesPost(String jobId, List channelsValues) throws ApiException {
ApiResponse> resp = jobsJobIdChannelsValuesPostWithHttpInfo(jobId, channelsValues);
return resp.getData();
}
/**
*
*
* @param jobId (required)
* @param channelsValues (required)
* @return ApiResponse<List<BatchChannelValueError>>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
*/
public ApiResponse> jobsJobIdChannelsValuesPostWithHttpInfo(String jobId, List channelsValues) throws ApiException {
com.squareup.okhttp.Call call = jobsJobIdChannelsValuesPostCall(jobId, channelsValues, null, null);
Type localVarReturnType = new TypeToken