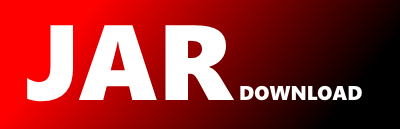
io.deepsense.neptune.apiclient.model.Job Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-api-client Show documentation
Show all versions of neptune-api-client Show documentation
Enables integration with Neptune in your Java code
/**
* Neptune API
* Neptune API
*
* OpenAPI spec version: 1.4_c9e4693-SNAPSHOT
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.deepsense.neptune.apiclient.model;
import java.util.Objects;
import com.google.gson.annotations.SerializedName;
import io.deepsense.neptune.apiclient.model.Action;
import io.deepsense.neptune.apiclient.model.Channel;
import io.deepsense.neptune.apiclient.model.ChannelWithValue;
import io.deepsense.neptune.apiclient.model.Chart;
import io.deepsense.neptune.apiclient.model.JobState;
import io.deepsense.neptune.apiclient.model.KeyValueProperty;
import io.deepsense.neptune.apiclient.model.Parameter;
import io.deepsense.neptune.apiclient.model.ParameterValue;
import io.deepsense.neptune.apiclient.model.ThirdPartyData;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
/**
* Job
*/
@javax.annotation.Generated(value = "class io.swagger.codegen.languages.JavaClientCodegen", date = "2016-12-09T18:25:08.092+01:00")
public class Job {
@SerializedName("id")
private String id = null;
@SerializedName("name")
private String name = null;
@SerializedName("description")
private String description = "";
@SerializedName("project")
private String project = "";
@SerializedName("owner")
private String owner = null;
@SerializedName("timeOfCreation")
private OffsetDateTime timeOfCreation = null;
@SerializedName("timeOfCompletion")
private OffsetDateTime timeOfCompletion = null;
@SerializedName("timeOfEnteredRunningState")
private OffsetDateTime timeOfEnteredRunningState = null;
@SerializedName("state")
private JobState state = null;
@SerializedName("responding")
private Boolean responding = null;
@SerializedName("tags")
private List tags = new ArrayList();
@SerializedName("properties")
private List properties = new ArrayList();
@SerializedName("channels")
private List channels = new ArrayList();
@SerializedName("charts")
private List charts = new ArrayList();
@SerializedName("channelsLastValues")
private List channelsLastValues = new ArrayList();
@SerializedName("parameters")
private List parameters = new ArrayList();
@SerializedName("parameterValues")
private List parameterValues = new ArrayList();
@SerializedName("actions")
private List actions = new ArrayList();
@SerializedName("traceback")
private String traceback = null;
@SerializedName("dumpDirLocation")
private String dumpDirLocation = null;
@SerializedName("dumpDirRoot")
private String dumpDirRoot = null;
@SerializedName("sourceCodeLocation")
private String sourceCodeLocation = null;
@SerializedName("stdoutLogLocation")
private String stdoutLogLocation = null;
@SerializedName("stderrLogLocation")
private String stderrLogLocation = null;
@SerializedName("execCommand")
private String execCommand = null;
@SerializedName("archived")
private Boolean archived = null;
@SerializedName("trashed")
private Boolean trashed = null;
@SerializedName("size")
private Long size = null;
@SerializedName("thirdPartyData")
private ThirdPartyData thirdPartyData = null;
@SerializedName("dockerImage")
private String dockerImage = null;
public Job id(String id) {
this.id = id;
return this;
}
/**
* Get id
* @return id
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public Job name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Job description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public Job project(String project) {
this.project = project;
return this;
}
/**
* Get project
* @return project
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getProject() {
return project;
}
public void setProject(String project) {
this.project = project;
}
public Job owner(String owner) {
this.owner = owner;
return this;
}
/**
* Get owner
* @return owner
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getOwner() {
return owner;
}
public void setOwner(String owner) {
this.owner = owner;
}
public Job timeOfCreation(OffsetDateTime timeOfCreation) {
this.timeOfCreation = timeOfCreation;
return this;
}
/**
* Get timeOfCreation
* @return timeOfCreation
**/
@ApiModelProperty(example = "null", required = true, value = "")
public OffsetDateTime getTimeOfCreation() {
return timeOfCreation;
}
public void setTimeOfCreation(OffsetDateTime timeOfCreation) {
this.timeOfCreation = timeOfCreation;
}
public Job timeOfCompletion(OffsetDateTime timeOfCompletion) {
this.timeOfCompletion = timeOfCompletion;
return this;
}
/**
* Present only when the job is completed
* @return timeOfCompletion
**/
@ApiModelProperty(example = "null", value = "Present only when the job is completed")
public OffsetDateTime getTimeOfCompletion() {
return timeOfCompletion;
}
public void setTimeOfCompletion(OffsetDateTime timeOfCompletion) {
this.timeOfCompletion = timeOfCompletion;
}
public Job timeOfEnteredRunningState(OffsetDateTime timeOfEnteredRunningState) {
this.timeOfEnteredRunningState = timeOfEnteredRunningState;
return this;
}
/**
* Present only when job has been running
* @return timeOfEnteredRunningState
**/
@ApiModelProperty(example = "null", value = "Present only when job has been running")
public OffsetDateTime getTimeOfEnteredRunningState() {
return timeOfEnteredRunningState;
}
public void setTimeOfEnteredRunningState(OffsetDateTime timeOfEnteredRunningState) {
this.timeOfEnteredRunningState = timeOfEnteredRunningState;
}
public Job state(JobState state) {
this.state = state;
return this;
}
/**
* Get state
* @return state
**/
@ApiModelProperty(example = "null", required = true, value = "")
public JobState getState() {
return state;
}
public void setState(JobState state) {
this.state = state;
}
public Job responding(Boolean responding) {
this.responding = responding;
return this;
}
/**
* Whether the connection between the job and the server is working.
* @return responding
**/
@ApiModelProperty(example = "null", required = true, value = "Whether the connection between the job and the server is working.")
public Boolean getResponding() {
return responding;
}
public void setResponding(Boolean responding) {
this.responding = responding;
}
public Job tags(List tags) {
this.tags = tags;
return this;
}
/**
* Get tags
* @return tags
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public Job properties(List properties) {
this.properties = properties;
return this;
}
/**
* Get properties
* @return properties
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getProperties() {
return properties;
}
public void setProperties(List properties) {
this.properties = properties;
}
public Job channels(List channels) {
this.channels = channels;
return this;
}
/**
* Get channels
* @return channels
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getChannels() {
return channels;
}
public void setChannels(List channels) {
this.channels = channels;
}
public Job charts(List charts) {
this.charts = charts;
return this;
}
/**
* Get charts
* @return charts
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getCharts() {
return charts;
}
public void setCharts(List charts) {
this.charts = charts;
}
public Job channelsLastValues(List channelsLastValues) {
this.channelsLastValues = channelsLastValues;
return this;
}
/**
* Get channelsLastValues
* @return channelsLastValues
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getChannelsLastValues() {
return channelsLastValues;
}
public void setChannelsLastValues(List channelsLastValues) {
this.channelsLastValues = channelsLastValues;
}
public Job parameters(List parameters) {
this.parameters = parameters;
return this;
}
/**
* Get parameters
* @return parameters
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getParameters() {
return parameters;
}
public void setParameters(List parameters) {
this.parameters = parameters;
}
public Job parameterValues(List parameterValues) {
this.parameterValues = parameterValues;
return this;
}
/**
* Get parameterValues
* @return parameterValues
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getParameterValues() {
return parameterValues;
}
public void setParameterValues(List parameterValues) {
this.parameterValues = parameterValues;
}
public Job actions(List actions) {
this.actions = actions;
return this;
}
/**
* Get actions
* @return actions
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getActions() {
return actions;
}
public void setActions(List actions) {
this.actions = actions;
}
public Job traceback(String traceback) {
this.traceback = traceback;
return this;
}
/**
* Traceback from the job; present only when the job's state is 'failed'
* @return traceback
**/
@ApiModelProperty(example = "null", required = true, value = "Traceback from the job; present only when the job's state is 'failed'")
public String getTraceback() {
return traceback;
}
public void setTraceback(String traceback) {
this.traceback = traceback;
}
public Job dumpDirLocation(String dumpDirLocation) {
this.dumpDirLocation = dumpDirLocation;
return this;
}
/**
* Link to job's dump directory.
* @return dumpDirLocation
**/
@ApiModelProperty(example = "null", required = true, value = "Link to job's dump directory.")
public String getDumpDirLocation() {
return dumpDirLocation;
}
public void setDumpDirLocation(String dumpDirLocation) {
this.dumpDirLocation = dumpDirLocation;
}
public Job dumpDirRoot(String dumpDirRoot) {
this.dumpDirRoot = dumpDirRoot;
return this;
}
/**
* Link to job's dump root directory.
* @return dumpDirRoot
**/
@ApiModelProperty(example = "null", required = true, value = "Link to job's dump root directory.")
public String getDumpDirRoot() {
return dumpDirRoot;
}
public void setDumpDirRoot(String dumpDirRoot) {
this.dumpDirRoot = dumpDirRoot;
}
public Job sourceCodeLocation(String sourceCodeLocation) {
this.sourceCodeLocation = sourceCodeLocation;
return this;
}
/**
* Link to job's source code.
* @return sourceCodeLocation
**/
@ApiModelProperty(example = "null", required = true, value = "Link to job's source code.")
public String getSourceCodeLocation() {
return sourceCodeLocation;
}
public void setSourceCodeLocation(String sourceCodeLocation) {
this.sourceCodeLocation = sourceCodeLocation;
}
public Job stdoutLogLocation(String stdoutLogLocation) {
this.stdoutLogLocation = stdoutLogLocation;
return this;
}
/**
* Link to job's stdout log.
* @return stdoutLogLocation
**/
@ApiModelProperty(example = "null", required = true, value = "Link to job's stdout log.")
public String getStdoutLogLocation() {
return stdoutLogLocation;
}
public void setStdoutLogLocation(String stdoutLogLocation) {
this.stdoutLogLocation = stdoutLogLocation;
}
public Job stderrLogLocation(String stderrLogLocation) {
this.stderrLogLocation = stderrLogLocation;
return this;
}
/**
* Link to job's stderr log.
* @return stderrLogLocation
**/
@ApiModelProperty(example = "null", required = true, value = "Link to job's stderr log.")
public String getStderrLogLocation() {
return stderrLogLocation;
}
public void setStderrLogLocation(String stderrLogLocation) {
this.stderrLogLocation = stderrLogLocation;
}
public Job execCommand(String execCommand) {
this.execCommand = execCommand;
return this;
}
/**
* Command to execute a job.
* @return execCommand
**/
@ApiModelProperty(example = "null", required = true, value = "Command to execute a job.")
public String getExecCommand() {
return execCommand;
}
public void setExecCommand(String execCommand) {
this.execCommand = execCommand;
}
public Job archived(Boolean archived) {
this.archived = archived;
return this;
}
/**
* Whether the job is archived.
* @return archived
**/
@ApiModelProperty(example = "null", required = true, value = "Whether the job is archived.")
public Boolean getArchived() {
return archived;
}
public void setArchived(Boolean archived) {
this.archived = archived;
}
public Job trashed(Boolean trashed) {
this.trashed = trashed;
return this;
}
/**
* Whether the job is trashed.
* @return trashed
**/
@ApiModelProperty(example = "null", required = true, value = "Whether the job is trashed.")
public Boolean getTrashed() {
return trashed;
}
public void setTrashed(Boolean trashed) {
this.trashed = trashed;
}
public Job size(Long size) {
this.size = size;
return this;
}
/**
* The size of all images from image channels in bytes.
* @return size
**/
@ApiModelProperty(example = "null", required = true, value = "The size of all images from image channels in bytes.")
public Long getSize() {
return size;
}
public void setSize(Long size) {
this.size = size;
}
public Job thirdPartyData(ThirdPartyData thirdPartyData) {
this.thirdPartyData = thirdPartyData;
return this;
}
/**
* Get thirdPartyData
* @return thirdPartyData
**/
@ApiModelProperty(example = "null", required = true, value = "")
public ThirdPartyData getThirdPartyData() {
return thirdPartyData;
}
public void setThirdPartyData(ThirdPartyData thirdPartyData) {
this.thirdPartyData = thirdPartyData;
}
public Job dockerImage(String dockerImage) {
this.dockerImage = dockerImage;
return this;
}
/**
* Docker image to execute the job in.
* @return dockerImage
**/
@ApiModelProperty(example = "null", value = "Docker image to execute the job in.")
public String getDockerImage() {
return dockerImage;
}
public void setDockerImage(String dockerImage) {
this.dockerImage = dockerImage;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Job job = (Job) o;
return Objects.equals(this.id, job.id) &&
Objects.equals(this.name, job.name) &&
Objects.equals(this.description, job.description) &&
Objects.equals(this.project, job.project) &&
Objects.equals(this.owner, job.owner) &&
Objects.equals(this.timeOfCreation, job.timeOfCreation) &&
Objects.equals(this.timeOfCompletion, job.timeOfCompletion) &&
Objects.equals(this.timeOfEnteredRunningState, job.timeOfEnteredRunningState) &&
Objects.equals(this.state, job.state) &&
Objects.equals(this.responding, job.responding) &&
Objects.equals(this.tags, job.tags) &&
Objects.equals(this.properties, job.properties) &&
Objects.equals(this.channels, job.channels) &&
Objects.equals(this.charts, job.charts) &&
Objects.equals(this.channelsLastValues, job.channelsLastValues) &&
Objects.equals(this.parameters, job.parameters) &&
Objects.equals(this.parameterValues, job.parameterValues) &&
Objects.equals(this.actions, job.actions) &&
Objects.equals(this.traceback, job.traceback) &&
Objects.equals(this.dumpDirLocation, job.dumpDirLocation) &&
Objects.equals(this.dumpDirRoot, job.dumpDirRoot) &&
Objects.equals(this.sourceCodeLocation, job.sourceCodeLocation) &&
Objects.equals(this.stdoutLogLocation, job.stdoutLogLocation) &&
Objects.equals(this.stderrLogLocation, job.stderrLogLocation) &&
Objects.equals(this.execCommand, job.execCommand) &&
Objects.equals(this.archived, job.archived) &&
Objects.equals(this.trashed, job.trashed) &&
Objects.equals(this.size, job.size) &&
Objects.equals(this.thirdPartyData, job.thirdPartyData) &&
Objects.equals(this.dockerImage, job.dockerImage);
}
@Override
public int hashCode() {
return Objects.hash(id, name, description, project, owner, timeOfCreation, timeOfCompletion, timeOfEnteredRunningState, state, responding, tags, properties, channels, charts, channelsLastValues, parameters, parameterValues, actions, traceback, dumpDirLocation, dumpDirRoot, sourceCodeLocation, stdoutLogLocation, stderrLogLocation, execCommand, archived, trashed, size, thirdPartyData, dockerImage);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class Job {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" project: ").append(toIndentedString(project)).append("\n");
sb.append(" owner: ").append(toIndentedString(owner)).append("\n");
sb.append(" timeOfCreation: ").append(toIndentedString(timeOfCreation)).append("\n");
sb.append(" timeOfCompletion: ").append(toIndentedString(timeOfCompletion)).append("\n");
sb.append(" timeOfEnteredRunningState: ").append(toIndentedString(timeOfEnteredRunningState)).append("\n");
sb.append(" state: ").append(toIndentedString(state)).append("\n");
sb.append(" responding: ").append(toIndentedString(responding)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" properties: ").append(toIndentedString(properties)).append("\n");
sb.append(" channels: ").append(toIndentedString(channels)).append("\n");
sb.append(" charts: ").append(toIndentedString(charts)).append("\n");
sb.append(" channelsLastValues: ").append(toIndentedString(channelsLastValues)).append("\n");
sb.append(" parameters: ").append(toIndentedString(parameters)).append("\n");
sb.append(" parameterValues: ").append(toIndentedString(parameterValues)).append("\n");
sb.append(" actions: ").append(toIndentedString(actions)).append("\n");
sb.append(" traceback: ").append(toIndentedString(traceback)).append("\n");
sb.append(" dumpDirLocation: ").append(toIndentedString(dumpDirLocation)).append("\n");
sb.append(" dumpDirRoot: ").append(toIndentedString(dumpDirRoot)).append("\n");
sb.append(" sourceCodeLocation: ").append(toIndentedString(sourceCodeLocation)).append("\n");
sb.append(" stdoutLogLocation: ").append(toIndentedString(stdoutLogLocation)).append("\n");
sb.append(" stderrLogLocation: ").append(toIndentedString(stderrLogLocation)).append("\n");
sb.append(" execCommand: ").append(toIndentedString(execCommand)).append("\n");
sb.append(" archived: ").append(toIndentedString(archived)).append("\n");
sb.append(" trashed: ").append(toIndentedString(trashed)).append("\n");
sb.append(" size: ").append(toIndentedString(size)).append("\n");
sb.append(" thirdPartyData: ").append(toIndentedString(thirdPartyData)).append("\n");
sb.append(" dockerImage: ").append(toIndentedString(dockerImage)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy