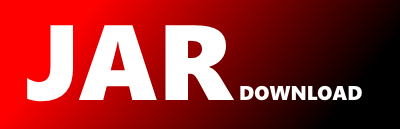
io.deepsense.neptune.apiclient.model.QueuedJobParams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-api-client Show documentation
Show all versions of neptune-api-client Show documentation
Enables integration with Neptune in your Java code
/**
* Neptune API
* Neptune API
*
* OpenAPI spec version: 1.4_c9e4693-SNAPSHOT
*
*
* NOTE: This class is auto generated by the swagger code generator program.
* https://github.com/swagger-api/swagger-codegen.git
* Do not edit the class manually.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.deepsense.neptune.apiclient.model;
import java.util.Objects;
import com.google.gson.annotations.SerializedName;
import io.deepsense.neptune.apiclient.model.KeyValueProperty;
import io.deepsense.neptune.apiclient.model.Parameter;
import io.deepsense.neptune.apiclient.model.ParameterValue;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import java.util.ArrayList;
import java.util.List;
/**
* QueuedJobParams
*/
@javax.annotation.Generated(value = "class io.swagger.codegen.languages.JavaClientCodegen", date = "2016-12-13T15:34:20.025+01:00")
public class QueuedJobParams {
@SerializedName("name")
private String name = null;
@SerializedName("description")
private String description = "";
@SerializedName("project")
private String project = "";
@SerializedName("tags")
private List tags = new ArrayList();
@SerializedName("parameters")
private List parameters = new ArrayList();
@SerializedName("parameterValues")
private List parameterValues = new ArrayList();
@SerializedName("properties")
private List properties = new ArrayList();
@SerializedName("dumpDirLocation")
private String dumpDirLocation = null;
@SerializedName("dumpDirRoot")
private String dumpDirRoot = null;
@SerializedName("sourceCodeLocation")
private String sourceCodeLocation = null;
@SerializedName("stdoutLogLocation")
private String stdoutLogLocation = null;
@SerializedName("stderrLogLocation")
private String stderrLogLocation = null;
@SerializedName("execCommand")
private String execCommand = null;
@SerializedName("dockerImage")
private String dockerImage = null;
public QueuedJobParams name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public QueuedJobParams description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public QueuedJobParams project(String project) {
this.project = project;
return this;
}
/**
* Get project
* @return project
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getProject() {
return project;
}
public void setProject(String project) {
this.project = project;
}
public QueuedJobParams tags(List tags) {
this.tags = tags;
return this;
}
/**
* Get tags
* @return tags
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getTags() {
return tags;
}
public void setTags(List tags) {
this.tags = tags;
}
public QueuedJobParams parameters(List parameters) {
this.parameters = parameters;
return this;
}
/**
* Get parameters
* @return parameters
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getParameters() {
return parameters;
}
public void setParameters(List parameters) {
this.parameters = parameters;
}
public QueuedJobParams parameterValues(List parameterValues) {
this.parameterValues = parameterValues;
return this;
}
/**
* Get parameterValues
* @return parameterValues
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getParameterValues() {
return parameterValues;
}
public void setParameterValues(List parameterValues) {
this.parameterValues = parameterValues;
}
public QueuedJobParams properties(List properties) {
this.properties = properties;
return this;
}
/**
* Get properties
* @return properties
**/
@ApiModelProperty(example = "null", required = true, value = "")
public List getProperties() {
return properties;
}
public void setProperties(List properties) {
this.properties = properties;
}
public QueuedJobParams dumpDirLocation(String dumpDirLocation) {
this.dumpDirLocation = dumpDirLocation;
return this;
}
/**
* Get dumpDirLocation
* @return dumpDirLocation
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getDumpDirLocation() {
return dumpDirLocation;
}
public void setDumpDirLocation(String dumpDirLocation) {
this.dumpDirLocation = dumpDirLocation;
}
public QueuedJobParams dumpDirRoot(String dumpDirRoot) {
this.dumpDirRoot = dumpDirRoot;
return this;
}
/**
* Get dumpDirRoot
* @return dumpDirRoot
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getDumpDirRoot() {
return dumpDirRoot;
}
public void setDumpDirRoot(String dumpDirRoot) {
this.dumpDirRoot = dumpDirRoot;
}
public QueuedJobParams sourceCodeLocation(String sourceCodeLocation) {
this.sourceCodeLocation = sourceCodeLocation;
return this;
}
/**
* Get sourceCodeLocation
* @return sourceCodeLocation
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getSourceCodeLocation() {
return sourceCodeLocation;
}
public void setSourceCodeLocation(String sourceCodeLocation) {
this.sourceCodeLocation = sourceCodeLocation;
}
public QueuedJobParams stdoutLogLocation(String stdoutLogLocation) {
this.stdoutLogLocation = stdoutLogLocation;
return this;
}
/**
* Get stdoutLogLocation
* @return stdoutLogLocation
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getStdoutLogLocation() {
return stdoutLogLocation;
}
public void setStdoutLogLocation(String stdoutLogLocation) {
this.stdoutLogLocation = stdoutLogLocation;
}
public QueuedJobParams stderrLogLocation(String stderrLogLocation) {
this.stderrLogLocation = stderrLogLocation;
return this;
}
/**
* Get stderrLogLocation
* @return stderrLogLocation
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getStderrLogLocation() {
return stderrLogLocation;
}
public void setStderrLogLocation(String stderrLogLocation) {
this.stderrLogLocation = stderrLogLocation;
}
public QueuedJobParams execCommand(String execCommand) {
this.execCommand = execCommand;
return this;
}
/**
* Get execCommand
* @return execCommand
**/
@ApiModelProperty(example = "null", required = true, value = "")
public String getExecCommand() {
return execCommand;
}
public void setExecCommand(String execCommand) {
this.execCommand = execCommand;
}
public QueuedJobParams dockerImage(String dockerImage) {
this.dockerImage = dockerImage;
return this;
}
/**
* Get dockerImage
* @return dockerImage
**/
@ApiModelProperty(example = "null", value = "")
public String getDockerImage() {
return dockerImage;
}
public void setDockerImage(String dockerImage) {
this.dockerImage = dockerImage;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
QueuedJobParams queuedJobParams = (QueuedJobParams) o;
return Objects.equals(this.name, queuedJobParams.name) &&
Objects.equals(this.description, queuedJobParams.description) &&
Objects.equals(this.project, queuedJobParams.project) &&
Objects.equals(this.tags, queuedJobParams.tags) &&
Objects.equals(this.parameters, queuedJobParams.parameters) &&
Objects.equals(this.parameterValues, queuedJobParams.parameterValues) &&
Objects.equals(this.properties, queuedJobParams.properties) &&
Objects.equals(this.dumpDirLocation, queuedJobParams.dumpDirLocation) &&
Objects.equals(this.dumpDirRoot, queuedJobParams.dumpDirRoot) &&
Objects.equals(this.sourceCodeLocation, queuedJobParams.sourceCodeLocation) &&
Objects.equals(this.stdoutLogLocation, queuedJobParams.stdoutLogLocation) &&
Objects.equals(this.stderrLogLocation, queuedJobParams.stderrLogLocation) &&
Objects.equals(this.execCommand, queuedJobParams.execCommand) &&
Objects.equals(this.dockerImage, queuedJobParams.dockerImage);
}
@Override
public int hashCode() {
return Objects.hash(name, description, project, tags, parameters, parameterValues, properties, dumpDirLocation, dumpDirRoot, sourceCodeLocation, stdoutLogLocation, stderrLogLocation, execCommand, dockerImage);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class QueuedJobParams {\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" project: ").append(toIndentedString(project)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" parameters: ").append(toIndentedString(parameters)).append("\n");
sb.append(" parameterValues: ").append(toIndentedString(parameterValues)).append("\n");
sb.append(" properties: ").append(toIndentedString(properties)).append("\n");
sb.append(" dumpDirLocation: ").append(toIndentedString(dumpDirLocation)).append("\n");
sb.append(" dumpDirRoot: ").append(toIndentedString(dumpDirRoot)).append("\n");
sb.append(" sourceCodeLocation: ").append(toIndentedString(sourceCodeLocation)).append("\n");
sb.append(" stdoutLogLocation: ").append(toIndentedString(stdoutLogLocation)).append("\n");
sb.append(" stderrLogLocation: ").append(toIndentedString(stderrLogLocation)).append("\n");
sb.append(" execCommand: ").append(toIndentedString(execCommand)).append("\n");
sb.append(" dockerImage: ").append(toIndentedString(dockerImage)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy