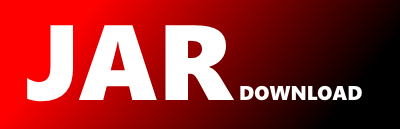
io.deepsense.neptune.clientlibrary.models.Job Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-client-library Show documentation
Show all versions of neptune-client-library Show documentation
Enables integration with Neptune in your Java code
/**
* Copyright (c) 2016, CodiLime Inc.
*/
package io.deepsense.neptune.clientlibrary.models;
import java.util.UUID;
import java.util.function.Function;
/**
* An object created to access and manipulate Neptune Job.
*
* Neptune job is a program registered for execution within Neptune.
* Every neptune job consists of source code and configuration files defining metadata of the job.
*/
public interface Job {
/**
*
* @return Id of this Job.
*/
UUID getId();
/**
*
* @return State of this job.
*/
JobState getJobState();
/**
* Creates a new numeric channel with a given name and extra parameters.
*
*
* Channel numericChannel = job.createChannel("numeric_channel", true, true);
*
* numericChannel.send(1.0, 2.5);
* numericChannel.send(1.5, 5);
*
*
* @param name Name of the channel
* @param isHistoryPersisted If True, all values sent to the channel are memorized.
* Otherwise only the last value is available.
* @param isLastValueExposed If True, the channel values can be displayed on the job list.
* @return Channel @see io.deepsense.neptune.clientlibrary.models.Channel
*/
Channel createNumericChannel(String name, boolean isHistoryPersisted, boolean isLastValueExposed);
/**
* Creates a new numeric channel with a given name. All values sent to the channel are
* memorized. The channel values can be displayed on the job list.
*
* @param name Name of the channel
* @return Channel @see io.deepsense.neptune.clientlibrary.models.Channel
*/
Channel createNumericChannel(String name);
/**
* Creates a new text channel with a given name and extra parameters.
*
*
* Channel textChannel = job.createTextChannel("text_channel", true, true);
*
* textChannel.send(2.5, "text 1");
* textChannel.send(3.0, "text 2");
*
*
*
* @param name Name of the channel
* @param isHistoryPersisted If True, all values sent to the channel are memorized.
* Otherwise only the last value is available.
* @param isLastValueExposed If True, the channel values can be displayed on the job list.
* @return Channel @see io.deepsense.neptune.clientlibrary.models.Channel
*/
Channel createTextChannel(String name, boolean isHistoryPersisted, boolean isLastValueExposed);
/**
* Creates a new text channel with a given name. All values sent to the channel are
* memorized. The channel values can be displayed on the job list.
*
* @param name Name of the channel
* @return Channel @see io.deepsense.neptune.clientlibrary.models.Channel
*/
Channel createTextChannel(String name);
/**
* Creates a new image channel with a given name.
*
*
* channel = job.createChannel("image_channel");
*
* channel.send(
* 1.0,
* new NeptuneImage(
* "#1 image name",
* "#1 image description",
* "/home/ubuntu/image1.jpg"
* )
* );
*
*
* @param name Name of the channel
* @return Channel @see io.deepsense.neptune.clientlibrary.models.Channel
*/
Channel createImageChannel(String name);
/**
* Creates a new chart that groups values of one or more numeric channels.
*
* Creating a chart:
*
*
* {@code
* Channel ch1 = job.createChannel(
* "ch1",
* ChannelType.NUMERIC);
*
* Channel ch2 = job.createChannel(
* "ch2",
* ChannelType.NUMERIC);
*
* ChartSeriesCollection series = job.createChartSeriesCollection();
* series.add("channel 1", ch1, ChartSeriesType.LINE);
* series.add("channel 2", ch2, ChartSeriesType.DOT);
*
* job.createChart(
* "comparison of ch1 & ch2",
* series);
* }
*
*
* @param name Unique chart name.
* @param series @see io.deepsense.neptune.clientlibrary.models.ChartSeriesCollection
* @return Chart @see io.deepsense.neptune.clientlibrary.models.Chart
*/
Chart createChart(String name, ChartSeriesCollection series);
/**
* Factory method to create ChartSeriesCollection.
*
* @return ChartSeriesCollection @see io.deepsense.neptune.clientlibrary.models.ChartSeriesCollection
*/
ChartSeriesCollection createChartSeriesCollection();
/**
* Registers a new action that calls handler with provided argument on invocation.
*
* Registering an action:
*
*
* Session session = ...
* String saveModel(String path) {
* session.saveModel(path);
* return "model saved";
* }
*
* job.registerAction("save model", this::saveModel);
*
*
* @param name Unique action name.
* @param handler An one argument function that will be called on an action invocation.
* @return Action @see io.deepsense.neptune.clientlibrary.models.Action
*/
Action registerAction(String name, Function handler);
/**
* Gets the set of user-defined tags for the job.
* @see io.deepsense.neptune.clientlibrary.models.Tags
*
* @return Tags of this job.
*/
Tags getTags();
/**
* Gets the set of user-defined properties of the Job.
* @see io.deepsense.neptune.clientlibrary.models.JobProperties
*
* @return Properties of this job.
*/
JobProperties getProperties();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy