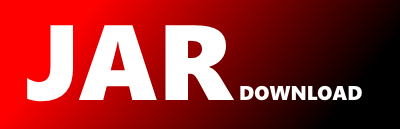
io.deepsense.neptune.clientlibrary.parsers.jobargumentsparser.JobArgumentsParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-client-library Show documentation
Show all versions of neptune-client-library Show documentation
Enables integration with Neptune in your Java code
/**
* Copyright (c) 2016, CodiLime Inc.
*/
package io.deepsense.neptune.clientlibrary.parsers.jobargumentsparser;
import com.google.common.base.Preconditions;
import net.sourceforge.argparse4j.ArgumentParsers;
import net.sourceforge.argparse4j.impl.Arguments;
import net.sourceforge.argparse4j.inf.ArgumentParser;
import net.sourceforge.argparse4j.inf.ArgumentParserException;
import net.sourceforge.argparse4j.inf.Namespace;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.net.URI;
import java.util.Arrays;
import java.util.UUID;
public class JobArgumentsParser {
private static final Logger logger = LoggerFactory.getLogger(JobArgumentsParser.class);
private static final String REST_API_URL_PARAMETER = "rest_api_url";
private static final String WEBSOCKET_API_URL_PARAMETER = "ws_api_url";
private static final String JOB_ID_PARAMETER = "job_id";
private static final String DEBUG_PARAMETER = "debug";
private final ArgumentParser argparseParser;
public JobArgumentsParser() {
argparseParser = ArgumentParsers.newArgumentParser("neptune-job");
Arrays.asList(REST_API_URL_PARAMETER, WEBSOCKET_API_URL_PARAMETER, JOB_ID_PARAMETER).forEach(
this::configureRequiredStringParameter);
argparseParser.addArgument("--" + DEBUG_PARAMETER)
.action(Arguments.storeTrue());
}
public JobArguments parse(String[] programArguments) {
try {
Namespace argumentsNamespace = argparseParser.parseArgs(Preconditions.checkNotNull(programArguments));
String restApiUrl = Preconditions.checkNotNull(
argumentsNamespace.getString(REST_API_URL_PARAMETER));
String websocketApiUrl = Preconditions.checkNotNull(
argumentsNamespace.getString(WEBSOCKET_API_URL_PARAMETER));
UUID jobId = UUID.fromString(Preconditions.checkNotNull(
argumentsNamespace.getString(JOB_ID_PARAMETER)));
boolean debug = argumentsNamespace.getBoolean(DEBUG_PARAMETER);
return new JobArguments(URI.create(restApiUrl), URI.create(websocketApiUrl), jobId, debug);
} catch (ArgumentParserException exc) {
logger.error("Invalid arguments passed from CLI to the job!", exc);
throw new RuntimeException(exc);
}
}
private void configureRequiredStringParameter(String parameterName) {
argparseParser.addArgument("--" + parameterName.replace('_', '-'))
.type(String.class)
.required(true);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy