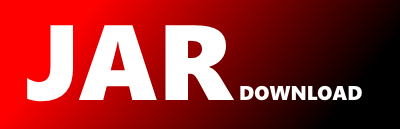
io.deepsense.neptune.clientlibrary.models.impl.job.OfflineJob Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-client-library Show documentation
Show all versions of neptune-client-library Show documentation
Enables integration with Neptune in your Java code
/**
* Copyright (c) 2016, CodiLime Inc.
*/
package io.deepsense.neptune.clientlibrary.models.impl.job;
import com.google.common.base.Objects;
import com.google.common.base.Preconditions;
import io.deepsense.neptune.clientlibrary.models.*;
import io.deepsense.neptune.clientlibrary.models.impl.channels.OfflineImageChannel;
import io.deepsense.neptune.clientlibrary.models.impl.channels.OfflineNumericChannel;
import io.deepsense.neptune.clientlibrary.models.impl.channels.OfflineTextChannel;
import io.deepsense.neptune.clientlibrary.models.impl.charts.OfflineChart;
import java.util.UUID;
import java.util.function.Function;
public class OfflineJob implements Job {
private final UUID id;
private final JobProperties properties;
private final Tags tags;
private JobState state;
public OfflineJob(UUID jobId, JobProperties properties, Tags tags) {
this.id = Preconditions.checkNotNull(jobId);
this.properties = Preconditions.checkNotNull(properties);
this.tags = Preconditions.checkNotNull(tags);
this.state = JobState.RUNNING;
}
@Override
public UUID getId() {
return id;
}
@Override
public JobState getJobState() {
return state;
}
@Override
public Channel createNumericChannel(String name, boolean isHistoryPersisted) {
return new OfflineNumericChannel(UUID.randomUUID(), name, isHistoryPersisted);
}
@Override
public Channel createNumericChannel(String name) {
return new OfflineNumericChannel(UUID.randomUUID(), name, true);
}
@Override
public Channel createTextChannel(String name, boolean isHistoryPersisted) {
return new OfflineTextChannel(UUID.randomUUID(), name, isHistoryPersisted);
}
@Override
public Channel createTextChannel(String name) {
return new OfflineTextChannel(UUID.randomUUID(), name, true);
}
@Override
public Channel createImageChannel(String name) {
return new OfflineImageChannel(UUID.randomUUID(), name, true);
}
@Override
public Chart createChart(String name, Iterable series) {
return new OfflineChart(UUID.randomUUID(), name, series);
}
@Override
public Action registerAction(String name, Function handler) {
throw new UnsupportedOperationException();
}
@Override
public Tags getTags() {
return tags;
}
@Override
public JobProperties getProperties() {
return properties;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
OfflineJob that = (OfflineJob) o;
return Objects.equal(id, that.id)
&& Objects.equal(properties, that.properties)
&& Objects.equal(tags, that.tags)
&& state == that.state;
}
@Override
public int hashCode() {
return Objects.hashCode(id, properties, tags, state);
}
@Override
public String toString() {
return "OfflineJob{"
+ "id=" + id
+ ", properties=" + properties
+ ", tags=" + tags
+ ", state=" + state
+ '}';
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy