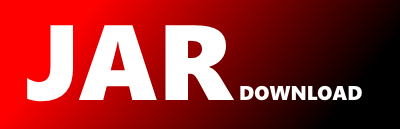
io.deepsense.neptune.clientlibrary.services.channelvaluesender.ChannelValueUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of neptune-client-library Show documentation
Show all versions of neptune-client-library Show documentation
Enables integration with Neptune in your Java code
/**
* Copyright (c) 2016, CodiLime Inc.
*/
package io.deepsense.neptune.clientlibrary.services.channelvaluesender;
import io.deepsense.neptune.apiclient.model.InputChannelValues;
import io.deepsense.neptune.apiclient.model.InputImage;
import io.deepsense.neptune.apiclient.model.Point;
import io.deepsense.neptune.clientlibrary.exceptions.common.NeptuneRuntimeException;
import java.nio.charset.Charset;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
public final class ChannelValueUtils {
private ChannelValueUtils() {
}
public static final String CHANNEL_VALUE_ENCODING = "utf-8";
public static long getEstimatedChannelValuesSize(InputChannelValues values) {
final int uuidSize = 36;
final long pointsTotalSize = values.getValues().stream()
.mapToLong(ChannelValueUtils::getEstimatedPointSize)
.sum();
final int isMetricSize = 5;
return uuidSize + pointsTotalSize + isMetricSize;
}
public static long getEstimatedPointSize(Point point) {
if (Optional.ofNullable(point.getY().getInputImageValue()).isPresent()) {
return getEstimatedImageSize(point.getY().getInputImageValue());
} else if (Optional.ofNullable(point.getY().getTextValue()).isPresent()) {
return getEstimatedTextSize(point.getY().getTextValue());
} else if (Optional.ofNullable(point.getY().getNumericValue()).isPresent()) {
return getEstimatedNumericSize();
} else {
throw new NeptuneRuntimeException("Unsupported type of Point!");
}
}
private static long getEstimatedImageSize(InputImage image) {
List imageFields = Arrays.asList(image.getData(), image.getDescription(), image.getName());
return imageFields.stream()
.mapToLong(ChannelValueUtils::getEstimatedTextSize)
.sum();
}
private static long getEstimatedTextSize(String value) {
return value.getBytes(Charset.forName(CHANNEL_VALUE_ENCODING)).length;
}
private static long getEstimatedNumericSize() {
return String.valueOf(Long.MAX_VALUE).length();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy