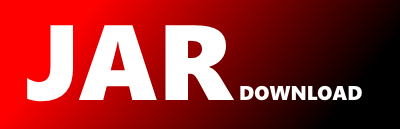
io.dekorate.halkyon.model.BuildConfigFluentImpl Maven / Gradle / Ivy
package io.dekorate.halkyon.model;
import java.lang.StringBuffer;
import java.lang.StringBuilder;
import io.dekorate.deps.kubernetes.api.builder.BaseFluent;
import java.lang.Object;
import java.lang.String;
import java.lang.Boolean;
public class BuildConfigFluentImpl> extends BaseFluent implements BuildConfigFluent {
private String type;
private String url;
private String ref;
private String contextPath;
private String moduleDirName;
public BuildConfigFluentImpl() {
}
public BuildConfigFluentImpl(BuildConfig instance) {
this.withType(instance.getType());
this.withUrl(instance.getUrl());
this.withRef(instance.getRef());
this.withContextPath(instance.getContextPath());
this.withModuleDirName(instance.getModuleDirName());
}
public String getType() {
return this.type;
}
public A withType(String type) {
this.type=type; return (A) this;
}
public Boolean hasType() {
return this.type != null;
}
public A withNewType(String arg1) {
return (A)withType(new String(arg1));
}
public A withNewType(StringBuilder arg1) {
return (A)withType(new String(arg1));
}
public A withNewType(StringBuffer arg1) {
return (A)withType(new String(arg1));
}
public String getUrl() {
return this.url;
}
public A withUrl(String url) {
this.url=url; return (A) this;
}
public Boolean hasUrl() {
return this.url != null;
}
public A withNewUrl(String arg1) {
return (A)withUrl(new String(arg1));
}
public A withNewUrl(StringBuilder arg1) {
return (A)withUrl(new String(arg1));
}
public A withNewUrl(StringBuffer arg1) {
return (A)withUrl(new String(arg1));
}
public String getRef() {
return this.ref;
}
public A withRef(String ref) {
this.ref=ref; return (A) this;
}
public Boolean hasRef() {
return this.ref != null;
}
public A withNewRef(String arg1) {
return (A)withRef(new String(arg1));
}
public A withNewRef(StringBuilder arg1) {
return (A)withRef(new String(arg1));
}
public A withNewRef(StringBuffer arg1) {
return (A)withRef(new String(arg1));
}
public String getContextPath() {
return this.contextPath;
}
public A withContextPath(String contextPath) {
this.contextPath=contextPath; return (A) this;
}
public Boolean hasContextPath() {
return this.contextPath != null;
}
public A withNewContextPath(String arg1) {
return (A)withContextPath(new String(arg1));
}
public A withNewContextPath(StringBuilder arg1) {
return (A)withContextPath(new String(arg1));
}
public A withNewContextPath(StringBuffer arg1) {
return (A)withContextPath(new String(arg1));
}
public String getModuleDirName() {
return this.moduleDirName;
}
public A withModuleDirName(String moduleDirName) {
this.moduleDirName=moduleDirName; return (A) this;
}
public Boolean hasModuleDirName() {
return this.moduleDirName != null;
}
public A withNewModuleDirName(String arg1) {
return (A)withModuleDirName(new String(arg1));
}
public A withNewModuleDirName(StringBuilder arg1) {
return (A)withModuleDirName(new String(arg1));
}
public A withNewModuleDirName(StringBuffer arg1) {
return (A)withModuleDirName(new String(arg1));
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
BuildConfigFluentImpl that = (BuildConfigFluentImpl) o;
if (type != null ? !type.equals(that.type) :that.type != null) return false;
if (url != null ? !url.equals(that.url) :that.url != null) return false;
if (ref != null ? !ref.equals(that.ref) :that.ref != null) return false;
if (contextPath != null ? !contextPath.equals(that.contextPath) :that.contextPath != null) return false;
if (moduleDirName != null ? !moduleDirName.equals(that.moduleDirName) :that.moduleDirName != null) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(type, url, ref, contextPath, moduleDirName, super.hashCode());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy