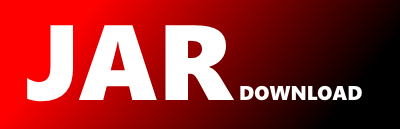
io.dekorate.helm.config.ValueReferenceFluentImpl Maven / Gradle / Ivy
package io.dekorate.helm.config;
import java.lang.Integer;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.ArrayList;
import java.util.Collection;
import java.lang.Object;
import java.util.List;
import java.lang.String;
import java.lang.Boolean;
/**
* Generated
*/
@SuppressWarnings(value = "unchecked")
public class ValueReferenceFluentImpl> extends BaseFluent implements ValueReferenceFluent{
public ValueReferenceFluentImpl() {
}
public ValueReferenceFluentImpl(ValueReference instance) {
this.withProperty(instance.getProperty());
this.withPaths(instance.getPaths());
this.withProfile(instance.getProfile());
this.withValue(instance.getValue());
this.withExpression(instance.getExpression());
}
private String property;
private List paths = new ArrayList();
private String profile;
private String value;
private String expression;
public String getProperty() {
return this.property;
}
public A withProperty(String property) {
this.property=property; return (A) this;
}
public Boolean hasProperty() {
return this.property != null;
}
public A withPaths(java.lang.String... paths) {
if (this.paths != null) {this.paths.clear();}
if (paths != null) {for (String item :paths){ this.addToPaths(item);}} return (A) this;
}
public String[] getPaths() {
//This needs to work with primitives, so we use arrays.
int size = paths != null ? paths.size() : 0;
String[] result = new String[size];
if (size == 0) {
return result;
}
int index = 0;
for (String item : paths) {
result[index++]=item;
}
return result;
}
public A addToPaths(Integer index,String item) {
if (this.paths == null) {this.paths = new ArrayList();}
this.paths.add(index, item);
return (A)this;
}
public A setToPaths(Integer index,String item) {
if (this.paths == null) {this.paths = new ArrayList();}
this.paths.set(index, item); return (A)this;
}
public A addToPaths(java.lang.String... items) {
if (this.paths == null) {this.paths = new ArrayList();}
for (String item : items) {this.paths.add(item);} return (A)this;
}
public A addAllToPaths(Collection items) {
if (this.paths == null) {this.paths = new ArrayList();}
for (String item : items) {this.paths.add(item);} return (A)this;
}
public A removeFromPaths(java.lang.String... items) {
for (String item : items) {if (this.paths!= null){ this.paths.remove(item);}} return (A)this;
}
public A removeAllFromPaths(Collection items) {
for (String item : items) {if (this.paths!= null){ this.paths.remove(item);}} return (A)this;
}
public Boolean hasPaths() {
return paths != null && !paths.isEmpty();
}
public String getProfile() {
return this.profile;
}
public A withProfile(String profile) {
this.profile=profile; return (A) this;
}
public Boolean hasProfile() {
return this.profile != null;
}
public String getValue() {
return this.value;
}
public A withValue(String value) {
this.value=value; return (A) this;
}
public Boolean hasValue() {
return this.value != null;
}
public String getExpression() {
return this.expression;
}
public A withExpression(String expression) {
this.expression=expression; return (A) this;
}
public Boolean hasExpression() {
return this.expression != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ValueReferenceFluentImpl that = (ValueReferenceFluentImpl) o;
if (property != null ? !property.equals(that.property) :that.property != null) return false;
if (paths != null ? !paths.equals(that.paths) :that.paths != null) return false;
if (profile != null ? !profile.equals(that.profile) :that.profile != null) return false;
if (value != null ? !value.equals(that.value) :that.value != null) return false;
if (expression != null ? !expression.equals(that.expression) :that.expression != null) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(property, paths, profile, value, expression, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (property != null) { sb.append("property:"); sb.append(property + ","); }
if (paths != null && !paths.isEmpty()) { sb.append("paths:"); sb.append(paths + ","); }
if (profile != null) { sb.append("profile:"); sb.append(profile + ","); }
if (value != null) { sb.append("value:"); sb.append(value + ","); }
if (expression != null) { sb.append("expression:"); sb.append(expression); }
sb.append("}");
return sb.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy