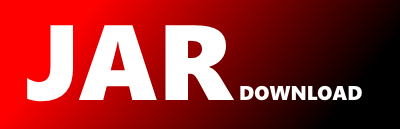
io.dekorate.helm.config.HelmChartConfig Maven / Gradle / Ivy
package io.dekorate.helm.config;
import io.dekorate.kubernetes.config.ConfigKey;
import io.dekorate.kubernetes.config.Configuration;
import java.lang.String;
import io.dekorate.project.Project;
import java.lang.Object;
import java.lang.Boolean;
import java.util.Map;
import io.sundr.builder.annotations.Buildable;
/**
* Generated
*/
@Buildable(builderPackage = "io.fabric8.kubernetes.api.builder")
public class HelmChartConfig extends Configuration{
public HelmChartConfig() {
}
public HelmChartConfig(Project project,Map attributes,Boolean enabled,String name,String home,String[] sources,String version,String description,String[] keywords,Maintainer[] maintainers,String icon,String apiVersion,String condition,String tags,String appVersion,Boolean deprecated,Annotation[] annotations,String kubeVersion,HelmDependency[] dependencies,String type,String valuesRootAlias,Boolean createTarFile,String extension,String tarFileClassifier,String notes,String inputFolder,String outputFolder,ValueReference[] values,HelmExpression[] expressions,AddIfStatement[] addIfStatements) {
super(project, attributes);
this.enabled = enabled;
this.name = name;
this.home = home;
this.sources = sources != null ? sources : new String[0];
this.version = version;
this.description = description;
this.keywords = keywords != null ? keywords : new String[0];
this.maintainers = maintainers != null ? maintainers : new Maintainer[0];
this.icon = icon;
this.apiVersion = apiVersion;
this.condition = condition;
this.tags = tags;
this.appVersion = appVersion;
this.deprecated = deprecated;
this.annotations = annotations != null ? annotations : new Annotation[0];
this.kubeVersion = kubeVersion;
this.dependencies = dependencies != null ? dependencies : new HelmDependency[0];
this.type = type;
this.valuesRootAlias = valuesRootAlias;
this.createTarFile = createTarFile;
this.extension = extension;
this.tarFileClassifier = tarFileClassifier;
this.notes = notes;
this.inputFolder = inputFolder;
this.outputFolder = outputFolder;
this.values = values != null ? values : new ValueReference[0];
this.expressions = expressions != null ? expressions : new HelmExpression[0];
this.addIfStatements = addIfStatements != null ? addIfStatements : new AddIfStatement[0];
}
private Boolean enabled;
private String name;
private String home;
private String[] sources = new String[0];
private String version;
private String description;
private String[] keywords = new String[0];
private Maintainer[] maintainers = new Maintainer[0];
private String icon;
private String apiVersion;
private String condition;
private String tags;
private String appVersion;
private Boolean deprecated;
private Annotation[] annotations = new Annotation[0];
private String kubeVersion;
private HelmDependency[] dependencies = new HelmDependency[0];
private String type;
private String valuesRootAlias;
private Boolean createTarFile;
private String extension;
private String tarFileClassifier;
private String notes;
private String inputFolder;
private String outputFolder;
private ValueReference[] values = new ValueReference[0];
private HelmExpression[] expressions = new HelmExpression[0];
private AddIfStatement[] addIfStatements = new AddIfStatement[0];
public Boolean getEnabled() {
return this.enabled;
}
public boolean isEnabled() {
return this.enabled != null && this.enabled;
}
public String getName() {
return this.name;
}
public String getHome() {
return this.home;
}
public String[] getSources() {
return this.sources;
}
public String getVersion() {
return this.version;
}
public String getDescription() {
return this.description;
}
public String[] getKeywords() {
return this.keywords;
}
public Maintainer[] getMaintainers() {
return this.maintainers;
}
public String getIcon() {
return this.icon;
}
public String getApiVersion() {
return this.apiVersion;
}
public String getCondition() {
return this.condition;
}
public String getTags() {
return this.tags;
}
public String getAppVersion() {
return this.appVersion;
}
public Boolean getDeprecated() {
return this.deprecated;
}
public boolean isDeprecated() {
return this.deprecated != null && this.deprecated;
}
public Annotation[] getAnnotations() {
return this.annotations;
}
public String getKubeVersion() {
return this.kubeVersion;
}
public HelmDependency[] getDependencies() {
return this.dependencies;
}
public String getType() {
return this.type;
}
public String getValuesRootAlias() {
return this.valuesRootAlias;
}
public Boolean getCreateTarFile() {
return this.createTarFile;
}
public boolean isCreateTarFile() {
return this.createTarFile != null && this.createTarFile;
}
public String getExtension() {
return this.extension;
}
public String getTarFileClassifier() {
return this.tarFileClassifier;
}
public String getNotes() {
return this.notes;
}
public String getInputFolder() {
return this.inputFolder;
}
public String getOutputFolder() {
return this.outputFolder;
}
public ValueReference[] getValues() {
return this.values;
}
public HelmExpression[] getExpressions() {
return this.expressions;
}
public AddIfStatement[] getAddIfStatements() {
return this.addIfStatements;
}
public static HelmChartConfigBuilder newHelmChartConfigBuilder() {
return new HelmChartConfigBuilder();
}
public static HelmChartConfigBuilder newHelmChartConfigBuilderFromDefaults() {
return new HelmChartConfigBuilder().withEnabled(true).withApiVersion("v2").withDeprecated(false).withValuesRootAlias("app").withCreateTarFile(false).withExtension("tar.gz").withNotes("/NOTES.template.txt").withInputFolder("helm").withOutputFolder("helm");
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
HelmChartConfig that = (HelmChartConfig) o;
if (enabled != null ? !enabled.equals(that.enabled) :that.enabled != null) return false;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
if (home != null ? !home.equals(that.home) :that.home != null) return false;
if (sources != null ? !sources.equals(that.sources) :that.sources != null) return false;
if (version != null ? !version.equals(that.version) :that.version != null) return false;
if (description != null ? !description.equals(that.description) :that.description != null) return false;
if (keywords != null ? !keywords.equals(that.keywords) :that.keywords != null) return false;
if (maintainers != null ? !maintainers.equals(that.maintainers) :that.maintainers != null) return false;
if (icon != null ? !icon.equals(that.icon) :that.icon != null) return false;
if (apiVersion != null ? !apiVersion.equals(that.apiVersion) :that.apiVersion != null) return false;
if (condition != null ? !condition.equals(that.condition) :that.condition != null) return false;
if (tags != null ? !tags.equals(that.tags) :that.tags != null) return false;
if (appVersion != null ? !appVersion.equals(that.appVersion) :that.appVersion != null) return false;
if (deprecated != null ? !deprecated.equals(that.deprecated) :that.deprecated != null) return false;
if (annotations != null ? !annotations.equals(that.annotations) :that.annotations != null) return false;
if (kubeVersion != null ? !kubeVersion.equals(that.kubeVersion) :that.kubeVersion != null) return false;
if (dependencies != null ? !dependencies.equals(that.dependencies) :that.dependencies != null) return false;
if (type != null ? !type.equals(that.type) :that.type != null) return false;
if (valuesRootAlias != null ? !valuesRootAlias.equals(that.valuesRootAlias) :that.valuesRootAlias != null) return false;
if (createTarFile != null ? !createTarFile.equals(that.createTarFile) :that.createTarFile != null) return false;
if (extension != null ? !extension.equals(that.extension) :that.extension != null) return false;
if (tarFileClassifier != null ? !tarFileClassifier.equals(that.tarFileClassifier) :that.tarFileClassifier != null) return false;
if (notes != null ? !notes.equals(that.notes) :that.notes != null) return false;
if (inputFolder != null ? !inputFolder.equals(that.inputFolder) :that.inputFolder != null) return false;
if (outputFolder != null ? !outputFolder.equals(that.outputFolder) :that.outputFolder != null) return false;
if (values != null ? !values.equals(that.values) :that.values != null) return false;
if (expressions != null ? !expressions.equals(that.expressions) :that.expressions != null) return false;
if (addIfStatements != null ? !addIfStatements.equals(that.addIfStatements) :that.addIfStatements != null) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(enabled, name, home, sources, version, description, keywords, maintainers, icon, apiVersion, condition, tags, appVersion, deprecated, annotations, kubeVersion, dependencies, type, valuesRootAlias, createTarFile, extension, tarFileClassifier, notes, inputFolder, outputFolder, values, expressions, addIfStatements, super.hashCode());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy