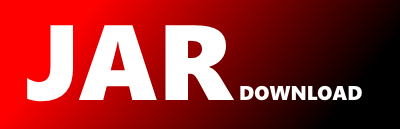
io.dekorate.helm.config.HelmDependencyFluentImpl Maven / Gradle / Ivy
package io.dekorate.helm.config;
import java.lang.Integer;
import java.lang.SuppressWarnings;
import io.fabric8.kubernetes.api.builder.BaseFluent;
import java.util.ArrayList;
import java.util.Collection;
import java.lang.Object;
import java.util.List;
import java.lang.String;
import java.lang.Boolean;
/**
* Generated
*/
@SuppressWarnings(value = "unchecked")
public class HelmDependencyFluentImpl> extends BaseFluent implements HelmDependencyFluent{
public HelmDependencyFluentImpl() {
}
public HelmDependencyFluentImpl(HelmDependency instance) {
this.withName(instance.getName());
this.withVersion(instance.getVersion());
this.withRepository(instance.getRepository());
this.withCondition(instance.getCondition());
this.withTags(instance.getTags());
this.withEnabled(instance.getEnabled());
this.withAlias(instance.getAlias());
}
private String name;
private String version;
private String repository;
private String condition;
private List tags = new ArrayList();
private Boolean enabled;
private String alias;
public String getName() {
return this.name;
}
public A withName(String name) {
this.name=name; return (A) this;
}
public Boolean hasName() {
return this.name != null;
}
public String getVersion() {
return this.version;
}
public A withVersion(String version) {
this.version=version; return (A) this;
}
public Boolean hasVersion() {
return this.version != null;
}
public String getRepository() {
return this.repository;
}
public A withRepository(String repository) {
this.repository=repository; return (A) this;
}
public Boolean hasRepository() {
return this.repository != null;
}
public String getCondition() {
return this.condition;
}
public A withCondition(String condition) {
this.condition=condition; return (A) this;
}
public Boolean hasCondition() {
return this.condition != null;
}
public A withTags(java.lang.String... tags) {
if (this.tags != null) {this.tags.clear();}
if (tags != null) {for (String item :tags){ this.addToTags(item);}} return (A) this;
}
public String[] getTags() {
//This needs to work with primitives, so we use arrays.
int size = tags != null ? tags.size() : 0;
String[] result = new String[size];
if (size == 0) {
return result;
}
int index = 0;
for (String item : tags) {
result[index++]=item;
}
return result;
}
public A addToTags(Integer index,String item) {
if (this.tags == null) {this.tags = new ArrayList();}
this.tags.add(index, item);
return (A)this;
}
public A setToTags(Integer index,String item) {
if (this.tags == null) {this.tags = new ArrayList();}
this.tags.set(index, item); return (A)this;
}
public A addToTags(java.lang.String... items) {
if (this.tags == null) {this.tags = new ArrayList();}
for (String item : items) {this.tags.add(item);} return (A)this;
}
public A addAllToTags(Collection items) {
if (this.tags == null) {this.tags = new ArrayList();}
for (String item : items) {this.tags.add(item);} return (A)this;
}
public A removeFromTags(java.lang.String... items) {
for (String item : items) {if (this.tags!= null){ this.tags.remove(item);}} return (A)this;
}
public A removeAllFromTags(Collection items) {
for (String item : items) {if (this.tags!= null){ this.tags.remove(item);}} return (A)this;
}
public Boolean hasTags() {
return tags != null && !tags.isEmpty();
}
public Boolean getEnabled() {
return this.enabled;
}
public A withEnabled(Boolean enabled) {
this.enabled=enabled; return (A) this;
}
public Boolean hasEnabled() {
return this.enabled != null;
}
public String getAlias() {
return this.alias;
}
public A withAlias(String alias) {
this.alias=alias; return (A) this;
}
public Boolean hasAlias() {
return this.alias != null;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HelmDependencyFluentImpl that = (HelmDependencyFluentImpl) o;
if (name != null ? !name.equals(that.name) :that.name != null) return false;
if (version != null ? !version.equals(that.version) :that.version != null) return false;
if (repository != null ? !repository.equals(that.repository) :that.repository != null) return false;
if (condition != null ? !condition.equals(that.condition) :that.condition != null) return false;
if (tags != null ? !tags.equals(that.tags) :that.tags != null) return false;
if (enabled != null ? !enabled.equals(that.enabled) :that.enabled != null) return false;
if (alias != null ? !alias.equals(that.alias) :that.alias != null) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(name, version, repository, condition, tags, enabled, alias, super.hashCode());
}
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (name != null) { sb.append("name:"); sb.append(name + ","); }
if (version != null) { sb.append("version:"); sb.append(version + ","); }
if (repository != null) { sb.append("repository:"); sb.append(repository + ","); }
if (condition != null) { sb.append("condition:"); sb.append(condition + ","); }
if (tags != null && !tags.isEmpty()) { sb.append("tags:"); sb.append(tags + ","); }
if (enabled != null) { sb.append("enabled:"); sb.append(enabled + ","); }
if (alias != null) { sb.append("alias:"); sb.append(alias); }
sb.append("}");
return sb.toString();
}
public A withEnabled() {
return withEnabled(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy