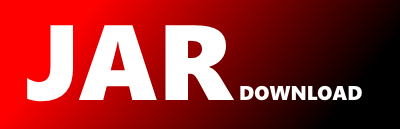
io.dekorate.openshift.config.OpenshiftConfigBuilder Maven / Gradle / Ivy
package io.dekorate.openshift.config;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.Object;
import java.lang.Boolean;
public class OpenshiftConfigBuilder extends OpenshiftConfigFluentImpl implements VisitableBuilder {
OpenshiftConfigFluent> fluent;
Boolean validationEnabled;
public OpenshiftConfigBuilder() {
this(true);
}
public OpenshiftConfigBuilder(Boolean validationEnabled) {
this(new OpenshiftConfig(), validationEnabled);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent) {
this(fluent, true);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent,Boolean validationEnabled) {
this(fluent, new OpenshiftConfig(), validationEnabled);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent,OpenshiftConfig instance) {
this(fluent, instance, true);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent,OpenshiftConfig instance,Boolean validationEnabled) {
this.fluent = fluent;
fluent.withProject(instance.getProject());
fluent.withAttributes(instance.getAttributes());
fluent.withPartOf(instance.getPartOf());
fluent.withName(instance.getName());
fluent.withVersion(instance.getVersion());
fluent.withLabels(instance.getLabels());
fluent.withAnnotations(instance.getAnnotations());
fluent.withEnvVars(instance.getEnvVars());
fluent.withWorkingDir(instance.getWorkingDir());
fluent.withCommand(instance.getCommand());
fluent.withArguments(instance.getArguments());
fluent.withServiceAccount(instance.getServiceAccount());
fluent.withHost(instance.getHost());
fluent.withPorts(instance.getPorts());
fluent.withServiceType(instance.getServiceType());
fluent.withPvcVolumes(instance.getPvcVolumes());
fluent.withSecretVolumes(instance.getSecretVolumes());
fluent.withConfigMapVolumes(instance.getConfigMapVolumes());
fluent.withGitRepoVolumes(instance.getGitRepoVolumes());
fluent.withAwsElasticBlockStoreVolumes(instance.getAwsElasticBlockStoreVolumes());
fluent.withAzureDiskVolumes(instance.getAzureDiskVolumes());
fluent.withAzureFileVolumes(instance.getAzureFileVolumes());
fluent.withMounts(instance.getMounts());
fluent.withImagePullPolicy(instance.getImagePullPolicy());
fluent.withImagePullSecrets(instance.getImagePullSecrets());
fluent.withHostAliases(instance.getHostAliases());
fluent.withLivenessProbe(instance.getLivenessProbe());
fluent.withReadinessProbe(instance.getReadinessProbe());
fluent.withRequestResources(instance.getRequestResources());
fluent.withLimitResources(instance.getLimitResources());
fluent.withSidecars(instance.getSidecars());
fluent.withExpose(instance.getExpose());
fluent.withAutoDeployEnabled(instance.getAutoDeployEnabled());
fluent.withInitContainers(instance.getInitContainers());
fluent.withReplicas(instance.getReplicas());
fluent.withHeadless(instance.getHeadless());
this.validationEnabled = validationEnabled;
}
public OpenshiftConfigBuilder(OpenshiftConfig instance) {
this(instance,true);
}
public OpenshiftConfigBuilder(OpenshiftConfig instance,Boolean validationEnabled) {
this.fluent = this;
this.withProject(instance.getProject());
this.withAttributes(instance.getAttributes());
this.withPartOf(instance.getPartOf());
this.withName(instance.getName());
this.withVersion(instance.getVersion());
this.withLabels(instance.getLabels());
this.withAnnotations(instance.getAnnotations());
this.withEnvVars(instance.getEnvVars());
this.withWorkingDir(instance.getWorkingDir());
this.withCommand(instance.getCommand());
this.withArguments(instance.getArguments());
this.withServiceAccount(instance.getServiceAccount());
this.withHost(instance.getHost());
this.withPorts(instance.getPorts());
this.withServiceType(instance.getServiceType());
this.withPvcVolumes(instance.getPvcVolumes());
this.withSecretVolumes(instance.getSecretVolumes());
this.withConfigMapVolumes(instance.getConfigMapVolumes());
this.withGitRepoVolumes(instance.getGitRepoVolumes());
this.withAwsElasticBlockStoreVolumes(instance.getAwsElasticBlockStoreVolumes());
this.withAzureDiskVolumes(instance.getAzureDiskVolumes());
this.withAzureFileVolumes(instance.getAzureFileVolumes());
this.withMounts(instance.getMounts());
this.withImagePullPolicy(instance.getImagePullPolicy());
this.withImagePullSecrets(instance.getImagePullSecrets());
this.withHostAliases(instance.getHostAliases());
this.withLivenessProbe(instance.getLivenessProbe());
this.withReadinessProbe(instance.getReadinessProbe());
this.withRequestResources(instance.getRequestResources());
this.withLimitResources(instance.getLimitResources());
this.withSidecars(instance.getSidecars());
this.withExpose(instance.getExpose());
this.withAutoDeployEnabled(instance.getAutoDeployEnabled());
this.withInitContainers(instance.getInitContainers());
this.withReplicas(instance.getReplicas());
this.withHeadless(instance.getHeadless());
this.validationEnabled = validationEnabled;
}
public EditableOpenshiftConfig build() {
EditableOpenshiftConfig buildable = new EditableOpenshiftConfig(fluent.getProject(),fluent.getAttributes(),fluent.getPartOf(),fluent.getName(),fluent.getVersion(),fluent.getLabels(),fluent.getAnnotations(),fluent.getEnvVars(),fluent.getWorkingDir(),fluent.getCommand(),fluent.getArguments(),fluent.getServiceAccount(),fluent.getHost(),fluent.getPorts(),fluent.getServiceType(),fluent.getPvcVolumes(),fluent.getSecretVolumes(),fluent.getConfigMapVolumes(),fluent.getGitRepoVolumes(),fluent.getAwsElasticBlockStoreVolumes(),fluent.getAzureDiskVolumes(),fluent.getAzureFileVolumes(),fluent.getMounts(),fluent.getImagePullPolicy(),fluent.getImagePullSecrets(),fluent.getHostAliases(),fluent.getLivenessProbe(),fluent.getReadinessProbe(),fluent.getRequestResources(),fluent.getLimitResources(),fluent.getSidecars(),fluent.getExpose(),fluent.getAutoDeployEnabled(),fluent.getInitContainers(),fluent.getReplicas(),fluent.getHeadless());
return buildable;
}
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
if (!super.equals(o)) return false;
OpenshiftConfigBuilder that = (OpenshiftConfigBuilder) o;
if (fluent != null &&fluent != this ? !fluent.equals(that.fluent) :that.fluent != null &&fluent != this ) return false;
if (validationEnabled != null ? !validationEnabled.equals(that.validationEnabled) :that.validationEnabled != null) return false;
return true;
}
public int hashCode() {
return java.util.Objects.hash(fluent, validationEnabled, super.hashCode());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy