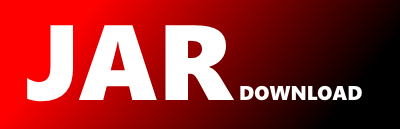
io.dekorate.openshift.config.OpenshiftConfigBuilder Maven / Gradle / Ivy
package io.dekorate.openshift.config;
import io.fabric8.kubernetes.api.builder.VisitableBuilder;
import java.lang.Boolean;
/**
* Generated
*/
public class OpenshiftConfigBuilder extends OpenshiftConfigFluentImpl implements VisitableBuilder{
public OpenshiftConfigBuilder() {
this(false);
}
public OpenshiftConfigBuilder(Boolean validationEnabled) {
this(new OpenshiftConfig(), validationEnabled);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent) {
this(fluent, false);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent,Boolean validationEnabled) {
this(fluent, new OpenshiftConfig(), validationEnabled);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent,OpenshiftConfig instance) {
this(fluent, instance, false);
}
public OpenshiftConfigBuilder(OpenshiftConfigFluent> fluent,OpenshiftConfig instance,Boolean validationEnabled) {
this.fluent = fluent;
fluent.withProject(instance.getProject());
fluent.withAttributes(instance.getAttributes());
fluent.withPartOf(instance.getPartOf());
fluent.withName(instance.getName());
fluent.withVersion(instance.getVersion());
fluent.withDeploymentKind(instance.getDeploymentKind());
fluent.withLabels(instance.getLabels());
fluent.withAnnotations(instance.getAnnotations());
fluent.withEnvVars(instance.getEnvVars());
fluent.withWorkingDir(instance.getWorkingDir());
fluent.withCommand(instance.getCommand());
fluent.withArguments(instance.getArguments());
fluent.withServiceAccount(instance.getServiceAccount());
fluent.withPorts(instance.getPorts());
fluent.withServiceType(instance.getServiceType());
fluent.withPvcVolumes(instance.getPvcVolumes());
fluent.withSecretVolumes(instance.getSecretVolumes());
fluent.withConfigMapVolumes(instance.getConfigMapVolumes());
fluent.withEmptyDirVolumes(instance.getEmptyDirVolumes());
fluent.withGitRepoVolumes(instance.getGitRepoVolumes());
fluent.withAwsElasticBlockStoreVolumes(instance.getAwsElasticBlockStoreVolumes());
fluent.withAzureDiskVolumes(instance.getAzureDiskVolumes());
fluent.withAzureFileVolumes(instance.getAzureFileVolumes());
fluent.withMounts(instance.getMounts());
fluent.withImagePullPolicy(instance.getImagePullPolicy());
fluent.withImagePullSecrets(instance.getImagePullSecrets());
fluent.withHostAliases(instance.getHostAliases());
fluent.withLivenessProbe(instance.getLivenessProbe());
fluent.withReadinessProbe(instance.getReadinessProbe());
fluent.withStartupProbe(instance.getStartupProbe());
fluent.withRequestResources(instance.getRequestResources());
fluent.withLimitResources(instance.getLimitResources());
fluent.withSidecars(instance.getSidecars());
fluent.withAutoDeployEnabled(instance.getAutoDeployEnabled());
fluent.withJobs(instance.getJobs());
fluent.withCronJobs(instance.getCronJobs());
fluent.withInitContainers(instance.getInitContainers());
fluent.withReplicas(instance.getReplicas());
fluent.withRoute(instance.getRoute());
fluent.withHeadless(instance.getHeadless());
this.validationEnabled = validationEnabled;
}
public OpenshiftConfigBuilder(OpenshiftConfig instance) {
this(instance,false);
}
public OpenshiftConfigBuilder(OpenshiftConfig instance,Boolean validationEnabled) {
this.fluent = this;
this.withProject(instance.getProject());
this.withAttributes(instance.getAttributes());
this.withPartOf(instance.getPartOf());
this.withName(instance.getName());
this.withVersion(instance.getVersion());
this.withDeploymentKind(instance.getDeploymentKind());
this.withLabels(instance.getLabels());
this.withAnnotations(instance.getAnnotations());
this.withEnvVars(instance.getEnvVars());
this.withWorkingDir(instance.getWorkingDir());
this.withCommand(instance.getCommand());
this.withArguments(instance.getArguments());
this.withServiceAccount(instance.getServiceAccount());
this.withPorts(instance.getPorts());
this.withServiceType(instance.getServiceType());
this.withPvcVolumes(instance.getPvcVolumes());
this.withSecretVolumes(instance.getSecretVolumes());
this.withConfigMapVolumes(instance.getConfigMapVolumes());
this.withEmptyDirVolumes(instance.getEmptyDirVolumes());
this.withGitRepoVolumes(instance.getGitRepoVolumes());
this.withAwsElasticBlockStoreVolumes(instance.getAwsElasticBlockStoreVolumes());
this.withAzureDiskVolumes(instance.getAzureDiskVolumes());
this.withAzureFileVolumes(instance.getAzureFileVolumes());
this.withMounts(instance.getMounts());
this.withImagePullPolicy(instance.getImagePullPolicy());
this.withImagePullSecrets(instance.getImagePullSecrets());
this.withHostAliases(instance.getHostAliases());
this.withLivenessProbe(instance.getLivenessProbe());
this.withReadinessProbe(instance.getReadinessProbe());
this.withStartupProbe(instance.getStartupProbe());
this.withRequestResources(instance.getRequestResources());
this.withLimitResources(instance.getLimitResources());
this.withSidecars(instance.getSidecars());
this.withAutoDeployEnabled(instance.getAutoDeployEnabled());
this.withJobs(instance.getJobs());
this.withCronJobs(instance.getCronJobs());
this.withInitContainers(instance.getInitContainers());
this.withReplicas(instance.getReplicas());
this.withRoute(instance.getRoute());
this.withHeadless(instance.getHeadless());
this.validationEnabled = validationEnabled;
}
OpenshiftConfigFluent> fluent;
Boolean validationEnabled;
public EditableOpenshiftConfig build() {
EditableOpenshiftConfig buildable = new EditableOpenshiftConfig(fluent.getProject(),fluent.getAttributes(),fluent.getPartOf(),fluent.getName(),fluent.getVersion(),fluent.getDeploymentKind(),fluent.getLabels(),fluent.getAnnotations(),fluent.getEnvVars(),fluent.getWorkingDir(),fluent.getCommand(),fluent.getArguments(),fluent.getServiceAccount(),fluent.getPorts(),fluent.getServiceType(),fluent.getPvcVolumes(),fluent.getSecretVolumes(),fluent.getConfigMapVolumes(),fluent.getEmptyDirVolumes(),fluent.getGitRepoVolumes(),fluent.getAwsElasticBlockStoreVolumes(),fluent.getAzureDiskVolumes(),fluent.getAzureFileVolumes(),fluent.getMounts(),fluent.getImagePullPolicy(),fluent.getImagePullSecrets(),fluent.getHostAliases(),fluent.getLivenessProbe(),fluent.getReadinessProbe(),fluent.getStartupProbe(),fluent.getRequestResources(),fluent.getLimitResources(),fluent.getSidecars(),fluent.getAutoDeployEnabled(),fluent.getJobs(),fluent.getCronJobs(),fluent.getInitContainers(),fluent.getReplicas(),fluent.getRoute(),fluent.getHeadless());
return buildable;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy