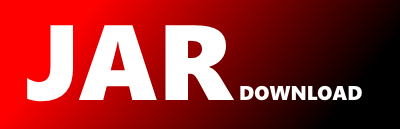
io.dekorate.prometheus.model.ServiceMonitorSpecFluentImpl Maven / Gradle / Ivy
package io.dekorate.prometheus.model;
import io.dekorate.deps.kubernetes.api.builder.VisitableBuilder;
import io.dekorate.deps.jackson.annotation.JsonProperty;
import io.dekorate.deps.kubernetes.api.model.LabelSelectorFluentImpl;
import io.dekorate.deps.kubernetes.api.builder.Nested;
import java.util.ArrayList;
import java.lang.String;
import io.dekorate.deps.kubernetes.api.builder.Predicate;
import java.lang.Deprecated;
import io.dekorate.deps.kubernetes.api.builder.BaseFluent;
import java.util.Iterator;
import java.util.List;
import java.lang.Boolean;
import io.dekorate.deps.kubernetes.api.model.LabelSelectorBuilder;
import io.dekorate.deps.kubernetes.api.model.LabelSelector;
import io.dekorate.deps.javax.validation.Valid;
import java.util.Collection;
import java.lang.Object;
public class ServiceMonitorSpecFluentImpl> extends io.dekorate.deps.kubernetes.api.builder.BaseFluent implements ServiceMonitorSpecFluent{
private LabelSelectorBuilder selector;
private java.util.List endpoints;
public ServiceMonitorSpecFluentImpl(){
}
public ServiceMonitorSpecFluentImpl(ServiceMonitorSpec instance){
this.withSelector(instance.getSelector());
this.withEndpoints(instance.getEndpoints());
}
/**
* This method has been deprecated, please use method buildSelector instead.
* @return The buildable object.
*/
@Deprecated public LabelSelector getSelector(){
return this.selector!=null?this.selector.build():null;
}
public LabelSelector buildSelector(){
return this.selector!=null?this.selector.build():null;
}
public A withSelector(LabelSelector selector){
_visitables.get("selector").remove(this.selector);
if (selector!=null){ this.selector= new LabelSelectorBuilder(selector); _visitables.get("selector").add(this.selector);} return (A) this;
}
public Boolean hasSelector(){
return this.selector != null;
}
public ServiceMonitorSpecFluent.SelectorNested withNewSelector(){
return new SelectorNestedImpl();
}
public ServiceMonitorSpecFluent.SelectorNested withNewSelectorLike(LabelSelector item){
return new SelectorNestedImpl(item);
}
public ServiceMonitorSpecFluent.SelectorNested editSelector(){
return withNewSelectorLike(getSelector());
}
public ServiceMonitorSpecFluent.SelectorNested editOrNewSelector(){
return withNewSelectorLike(getSelector() != null ? getSelector(): new LabelSelectorBuilder().build());
}
public ServiceMonitorSpecFluent.SelectorNested editOrNewSelectorLike(LabelSelector item){
return withNewSelectorLike(getSelector() != null ? getSelector(): item);
}
public A addToEndpoints(int index,Endpoint item){
if (this.endpoints == null) {this.endpoints = new ArrayList();}
EndpointBuilder builder = new EndpointBuilder(item);_visitables.get("endpoints").add(index >= 0 ? index : _visitables.get("endpoints").size(), builder);this.endpoints.add(index >= 0 ? index : endpoints.size(), builder); return (A)this;
}
public A setToEndpoints(int index,Endpoint item){
if (this.endpoints == null) {this.endpoints = new ArrayList();}
EndpointBuilder builder = new EndpointBuilder(item);
if (index < 0 || index >= _visitables.get("endpoints").size()) { _visitables.get("endpoints").add(builder); } else { _visitables.get("endpoints").set(index, builder);}
if (index < 0 || index >= endpoints.size()) { endpoints.add(builder); } else { endpoints.set(index, builder);}
return (A)this;
}
public A addToEndpoints(Endpoint... items){
if (this.endpoints == null) {this.endpoints = new ArrayList();}
for (Endpoint item : items) {EndpointBuilder builder = new EndpointBuilder(item);_visitables.get("endpoints").add(builder);this.endpoints.add(builder);} return (A)this;
}
public A addAllToEndpoints(Collection items){
if (this.endpoints == null) {this.endpoints = new ArrayList();}
for (Endpoint item : items) {EndpointBuilder builder = new EndpointBuilder(item);_visitables.get("endpoints").add(builder);this.endpoints.add(builder);} return (A)this;
}
public A removeFromEndpoints(Endpoint... items){
for (Endpoint item : items) {EndpointBuilder builder = new EndpointBuilder(item);_visitables.get("endpoints").remove(builder);if (this.endpoints != null) {this.endpoints.remove(builder);}} return (A)this;
}
public A removeAllFromEndpoints(Collection items){
for (Endpoint item : items) {EndpointBuilder builder = new EndpointBuilder(item);_visitables.get("endpoints").remove(builder);if (this.endpoints != null) {this.endpoints.remove(builder);}} return (A)this;
}
public A removeMatchingFromEndpoints(io.dekorate.deps.kubernetes.api.builder.Predicate predicate){
if (endpoints == null) return (A) this;
final Iterator each = endpoints.iterator();
final List visitables = _visitables.get("endpoints");
while (each.hasNext()) {
EndpointBuilder builder = each.next();
if (predicate.apply(builder)) {
visitables.remove(builder);
each.remove();
}
}
return (A)this;
}
/**
* This method has been deprecated, please use method buildEndpoints instead.
* @return The buildable object.
*/
@Deprecated public java.util.List getEndpoints(){
return build(endpoints);
}
public java.util.List buildEndpoints(){
return build(endpoints);
}
public Endpoint buildEndpoint(int index){
return this.endpoints.get(index).build();
}
public Endpoint buildFirstEndpoint(){
return this.endpoints.get(0).build();
}
public Endpoint buildLastEndpoint(){
return this.endpoints.get(endpoints.size() - 1).build();
}
public Endpoint buildMatchingEndpoint(io.dekorate.deps.kubernetes.api.builder.Predicate predicate){
for (EndpointBuilder item: endpoints) { if(predicate.apply(item)){ return item.build();} } return null;
}
public Boolean hasMatchingEndpoint(io.dekorate.deps.kubernetes.api.builder.Predicate predicate){
for (EndpointBuilder item: endpoints) { if(predicate.apply(item)){ return true;} } return false;
}
public A withEndpoints(java.util.List endpoints){
if (this.endpoints != null) { _visitables.get("endpoints").removeAll(this.endpoints);}
if (endpoints != null) {this.endpoints = new ArrayList(); for (Endpoint item : endpoints){this.addToEndpoints(item);}} else { this.endpoints = null;} return (A) this;
}
public A withEndpoints(Endpoint... endpoints){
if (this.endpoints != null) {this.endpoints.clear();}
if (endpoints != null) {for (Endpoint item :endpoints){ this.addToEndpoints(item);}} return (A) this;
}
public Boolean hasEndpoints(){
return endpoints != null && !endpoints.isEmpty();
}
public A addNewEndpoint(String port,String path,String interval,boolean honorLabels){
return (A)addToEndpoints(new Endpoint(port, path, interval, honorLabels));
}
public ServiceMonitorSpecFluent.EndpointsNested addNewEndpoint(){
return new EndpointsNestedImpl();
}
public ServiceMonitorSpecFluent.EndpointsNested addNewEndpointLike(Endpoint item){
return new EndpointsNestedImpl(-1, item);
}
public ServiceMonitorSpecFluent.EndpointsNested setNewEndpointLike(int index,Endpoint item){
return new EndpointsNestedImpl(index, item);
}
public ServiceMonitorSpecFluent.EndpointsNested editEndpoint(int index){
if (endpoints.size() <= index) throw new RuntimeException("Can't edit endpoints. Index exceeds size.");
return setNewEndpointLike(index, buildEndpoint(index));
}
public ServiceMonitorSpecFluent.EndpointsNested editFirstEndpoint(){
if (endpoints.size() == 0) throw new RuntimeException("Can't edit first endpoints. The list is empty.");
return setNewEndpointLike(0, buildEndpoint(0));
}
public ServiceMonitorSpecFluent.EndpointsNested editLastEndpoint(){
int index = endpoints.size() - 1;
if (index < 0) throw new RuntimeException("Can't edit last endpoints. The list is empty.");
return setNewEndpointLike(index, buildEndpoint(index));
}
public ServiceMonitorSpecFluent.EndpointsNested editMatchingEndpoint(io.dekorate.deps.kubernetes.api.builder.Predicate predicate){
int index = -1;
for (int i=0;i extends LabelSelectorFluentImpl> implements ServiceMonitorSpecFluent.SelectorNested,io.dekorate.deps.kubernetes.api.builder.Nested{
private final LabelSelectorBuilder builder;
SelectorNestedImpl(LabelSelector item){
this.builder = new LabelSelectorBuilder(this, item);
}
SelectorNestedImpl(){
this.builder = new LabelSelectorBuilder(this);
}
public N and(){
return (N) ServiceMonitorSpecFluentImpl.this.withSelector(builder.build());
}
public N endSelector(){
return and();
}
}
public class EndpointsNestedImpl extends EndpointFluentImpl> implements ServiceMonitorSpecFluent.EndpointsNested,io.dekorate.deps.kubernetes.api.builder.Nested{
private final EndpointBuilder builder;
private final int index;
EndpointsNestedImpl(int index,Endpoint item){
this.index = index;
this.builder = new EndpointBuilder(this, item);
}
EndpointsNestedImpl(){
this.index = -1;
this.builder = new EndpointBuilder(this);
}
public N and(){
return (N) ServiceMonitorSpecFluentImpl.this.setToEndpoints(index, builder.build());
}
public N endEndpoint(){
return and();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy