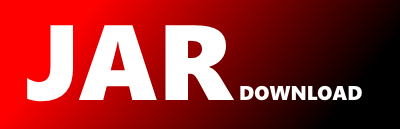
io.delta.flink.internal.options.DeltaConfigOption Maven / Gradle / Ivy
package io.delta.flink.internal.options;
import org.apache.flink.configuration.ConfigOption;
/**
* A wrapper class on Flink's {@link ConfigOption} exposing expected type for given option.
* The type is used for validation and value conversion for used options.
*
* @implNote
* The wrapped {@link ConfigOption} class hides value type in a way that even if we would extend
* it, we would nto have access to field type.
*/
public class DeltaConfigOption {
/**
* Wrapped {@link ConfigOption}
*/
private final ConfigOption decoratedOption;
/**
* Java class type for decorated option value.
*/
private final Class decoratedType;
/**
* Value type converter for this configuration option.
*/
private final OptionTypeConverter typeConverter;
private DeltaConfigOption(
ConfigOption decoratedOption,
Class type,
OptionTypeConverter typeConverter) {
this.decoratedOption = decoratedOption;
this.decoratedType = type;
this.typeConverter = typeConverter;
}
@SuppressWarnings("unchecked")
public static DeltaConfigOption of(ConfigOption configOption, Class type) {
return new DeltaConfigOption<>(
configOption,
type,
(OptionTypeConverter) new DefaultOptionTypeConverter()
);
}
public static DeltaConfigOption of(
ConfigOption configOption,
Class type,
OptionTypeConverter typeConverter) {
return new DeltaConfigOption<>(configOption, type, typeConverter);
}
/**
* @return {@link Class} type for option.
*/
public Class getValueType() {
return decoratedType;
}
/**
* @return the configuration key.
*/
public String key() {
return decoratedOption.key();
}
/**
* @return the default value, or null, if there is no default value.
*/
public T defaultValue() {
return decoratedOption.defaultValue();
}
//-------Keeping type safety with implementation of a Visitor pattern -------//
public void setOnConfig(DeltaConnectorConfiguration sourceConfiguration, boolean value) {
T convertedValue = typeConverter.convertType(this, value);
sourceConfiguration.addOption(this, convertedValue);
}
public void setOnConfig(DeltaConnectorConfiguration sourceConfiguration, int value) {
T convertedValue = typeConverter.convertType(this, value);
sourceConfiguration.addOption(this, convertedValue);
}
public void setOnConfig(DeltaConnectorConfiguration sourceConfiguration, long value) {
T convertedValue = typeConverter.convertType(this, value);
sourceConfiguration.addOption(this, convertedValue);
}
public void setOnConfig(DeltaConnectorConfiguration sourceConfiguration, String value) {
T convertedValue = typeConverter.convertType(this, value);
sourceConfiguration.addOption(this, convertedValue);
}
//---------------------------------------------------------------------------//
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy