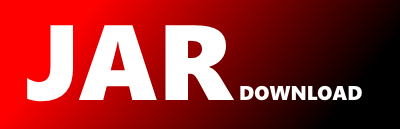
io.descoped.dc.api.services.ObjectCreator Maven / Gradle / Ivy
The newest version!
package io.descoped.dc.api.services;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.Deque;
import java.util.LinkedList;
import java.util.List;
public class ObjectCreator {
public static T newInstance(Class clazz, Services services) {
List> constructors = new ArrayList<>(List.of(clazz.getConstructors()));
// add default constructor unless declared
if (constructors.isEmpty()) {
constructors.add(getDefaultConstructor(clazz));
}
for (Constructor> constructor : constructors) {
Deque
© 2015 - 2025 Weber Informatics LLC | Privacy Policy