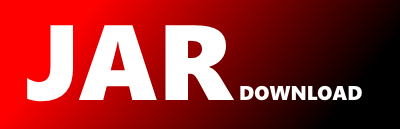
io.devbench.uibuilder.components.combobox.UIBuilderComboBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of uibuilder-combobox Show documentation
Show all versions of uibuilder-combobox Show documentation
A ComboBox component for the UIBuilder Framework
/*
*
* Copyright © 2018 Webvalto Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.devbench.uibuilder.components.combobox;
import com.vaadin.flow.component.*;
import com.vaadin.flow.component.dependency.JsModule;
import com.vaadin.flow.shared.Registration;
import io.devbench.uibuilder.components.combobox.event.ComboBoxComponentValueChangeEvent;
import io.devbench.uibuilder.components.combobox.event.SelectionChangedEvent;
import io.devbench.uibuilder.data.collectionds.datasource.component.AbstractDataSourceComponent;
import lombok.extern.slf4j.Slf4j;
import java.util.Objects;
@Slf4j
@Tag(UIBuilderComboBox.TAG_NAME)
@JsModule("./uibuilder-combobox/src/uibuilder-combobox.js")
public class UIBuilderComboBox
extends AbstractDataSourceComponent
implements HasValueAndElement, T> {
public static final String TAG_NAME = "uibuilder-combobox";
private T selectedValue;
@Override
@SuppressWarnings("unchecked")
public void onAttached() {
super.onAttached();
addListener(ComboBoxComponentValueChangeEvent.class, (ComponentEventListener) event -> {
ComboBoxComponentValueChangeEvent valueChangeEvent = (ComboBoxComponentValueChangeEvent) event;
T oldValue = selectedValue;
T newValue = findNewValueBasedOnEvent(valueChangeEvent);
selectedValue = newValue;
if (!Objects.equals(newValue, oldValue)) {
ComponentUtil.fireEvent(this, new SelectionChangedEvent<>(this, event.isFromClient(), oldValue, newValue));
}
});
}
private T findNewValueBasedOnEvent(ComboBoxComponentValueChangeEvent valueChangeEvent) {
if (valueChangeEvent.getValue() == null || valueChangeEvent.getValue().trim().isEmpty()) {
return null;
} else {
return getDataSource().findItemByIdValue(valueChangeEvent.getValue());
}
}
@Override
public void setValue(T value) {
if (isNewValueValid(value)) {
T oldValue = selectedValue;
selectedValue = value;
ComponentUtil.fireEvent(this, new SelectionChangedEvent<>(this, false, oldValue, value));
if (getDataSource() != null) {
getElement().callJsFunction("_onItemSelected", value != null ? getDataSource().convertToKey(value) : null);
}
}
}
@Override
public T getValue() {
return selectedValue;
}
@Override
public void refresh() {
getElement().callJsFunction("_refresh");
}
@Override
public void onItemsSet() {
setValue(null);
refresh();
}
@Override
@SuppressWarnings({"unchecked", "RedundantCast"})
public Registration addValueChangeListener(ValueChangeListener super SelectionChangedEvent> listener) {
return ComponentUtil.addListener(this, SelectionChangedEvent.class, (ComponentEventListener) event -> {
((ValueChangeListener) listener).valueChanged((SelectionChangedEvent) event);
});
}
@Synchronize("null-values-disabled-changed")
public String getNullValuesDisabled() {
return getElement().getProperty("nullValuesDisabled");
}
private boolean isNewValueValid(T value) {
if (value == null) {
return isNullValuesEnabled();
} else {
return true;
}
}
private boolean isNullValuesEnabled() {
return !Boolean.parseBoolean(getNullValuesDisabled());
}
@Override
protected void setSelectedItemsIfItemWasSetPreviously() {
if (selectedValue != null) {
getElement().callJsFunction("_onItemSelected", getDataSource().convertToKey(selectedValue));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy