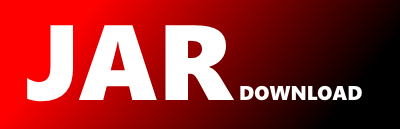
io.devbench.uibuilder.components.combobox.UIBuilderComboBox Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of uibuilder-combobox Show documentation
Show all versions of uibuilder-combobox Show documentation
A ComboBox component for the UIBuilder Framework
/*
*
* Copyright © 2018 Webvalto Ltd.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.devbench.uibuilder.components.combobox;
import com.vaadin.flow.component.*;
import com.vaadin.flow.component.dependency.JsModule;
import com.vaadin.flow.shared.Registration;
import io.devbench.uibuilder.components.combobox.event.ComboBoxComponentValueChangeEvent;
import io.devbench.uibuilder.components.combobox.event.CustomValueChangeEvent;
import io.devbench.uibuilder.components.combobox.event.SelectionChangedEvent;
import io.devbench.uibuilder.core.controllerbean.uiproperty.PropertyConverter;
import io.devbench.uibuilder.core.controllerbean.uiproperty.PropertyConverters;
import io.devbench.uibuilder.data.collectionds.datasource.component.AbstractDataSourceComponent;
import io.devbench.uibuilder.data.common.dataprovidersupport.KeyMapper;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import java.util.Objects;
import java.util.Optional;
import java.util.function.Function;
@Slf4j
@Tag(UIBuilderComboBox.TAG_NAME)
@JsModule("./uibuilder-combobox/src/uibuilder-combobox.js")
public class UIBuilderComboBox
extends AbstractDataSourceComponent
implements HasValueAndElement, T>, HasValidation {
public static final String TAG_NAME = "uibuilder-combobox";
@SuppressWarnings({"rawtypes", "unchecked"})
private static final PropertyConverter FUNCTION_CONVERTER =
(PropertyConverter) PropertyConverters.getConverterByType(Function.class);
private T selectedValue;
private String customValueFunctionId;
@Override
public void onAttached() {
super.onAttached();
addListener(ComboBoxComponentValueChangeEvent.class, (ComponentEventListener) event -> {
T oldValue = selectedValue;
T newValue = findValueBasedOnEvent(event);
selectedValue = newValue;
if (!Objects.equals(newValue, oldValue)) {
ComponentUtil.fireEvent(this, new SelectionChangedEvent<>(this, event.isFromClient(), oldValue, newValue));
}
});
}
private T findValueBasedOnEvent(ComboBoxComponentValueChangeEvent valueChangeEvent) {
String value = valueChangeEvent.getValue();
if (StringUtils.isBlank(value)) {
return null;
} else {
if (isAllowCustomValue()) {
return KeyMapper.decodeKey(value)
.map(jsonObject -> Objects.requireNonNull(getDataSource()).findItemByIdValue(jsonObject))
.orElseGet(() -> findCustomValueFunction()
.map(customValueFunction -> customValueFunction.apply(value))
.orElse(null));
} else {
return Objects.requireNonNull(getDataSource()).findItemByIdValue(value);
}
}
}
@Override
public void setValue(T value) {
if (isNewValueValid(value)) {
T oldValue = selectedValue;
selectedValue = value;
ComponentUtil.fireEvent(this, new SelectionChangedEvent<>(this, false, oldValue, value));
if (getDataSource() != null) {
getElement().callJsFunction("_onItemSelected", value != null ? getDataSource().convertToKey(value) : null);
}
}
}
@Override
public T getValue() {
return selectedValue;
}
@Override
public void refresh() {
getElement().callJsFunction("_refresh");
}
@Override
public void onItemsSet() {
setValue(null);
refresh();
}
@Override
@SuppressWarnings({"unchecked", "RedundantCast", "rawtypes"})
public Registration addValueChangeListener(ValueChangeListener super SelectionChangedEvent> listener) {
return addListener(SelectionChangedEvent.class, (ComponentEventListener) event -> {
((ValueChangeListener) listener).valueChanged((SelectionChangedEvent) event);
});
}
@SuppressWarnings({"unchecked", "rawtypes"})
public Registration addCustomValueChangeListener(CustomValueChangeEvent.CustomValueChangeListener listener) {
return addListener(CustomValueChangeEvent.class, (ComponentEventListener) event -> {
listener.customValueChanged((CustomValueChangeEvent) event);
});
}
@Synchronize("null-values-disabled-changed")
public boolean isNullValuesDisabled() {
return getElement().getProperty("nullValuesDisabled", false);
}
public void setNullValuesDisabled(boolean nullValuesDisabled) {
getElement().setProperty("nullValuesDisabled", nullValuesDisabled);
}
@Synchronize("allow-custom-value-changed")
public boolean isAllowCustomValue() {
return getElement().getProperty("allowCustomValue", false);
}
public void setAllowCustomValue(boolean allowCustomValue) {
getElement().setProperty("allowCustomValue", allowCustomValue);
}
public Optional> findCustomValueFunction() {
if (StringUtils.isNotBlank(customValueFunctionId)) {
@SuppressWarnings("unchecked")
Function function = (Function) FUNCTION_CONVERTER.convertFrom(customValueFunctionId);
return Optional.ofNullable(function);
}
return Optional.empty();
}
public void setCustomValueFunctionId(String customValueFunctionId) {
this.customValueFunctionId = customValueFunctionId;
}
private boolean isNewValueValid(T value) {
if (value == null) {
return !isNullValuesDisabled();
} else {
return true;
}
}
@Override
protected void setSelectedItemsIfItemWasSetPreviously() {
if (selectedValue != null) {
getElement().callJsFunction("_onItemSelected", Objects.requireNonNull(getDataSource()).convertToKey(selectedValue));
}
}
@Synchronize(property = "invalid", value = {"invalid-changed"})
public boolean isInvalid() {
return getElement().getProperty("invalid", false);
}
public void setInvalid(boolean invalid) {
getElement().setProperty("invalid", invalid);
}
public String getErrorMessage() {
return getElement().getProperty("errorMessage");
}
public void setErrorMessage(String errorMessage) {
getElement().setProperty("errorMessage", errorMessage == null ? "" : errorMessage);
}
@Synchronize(property = "opened", value = "opened-changed")
public boolean isOpened() {
return getElement().getProperty("opened", false);
}
public void setOpened(boolean opened) {
getElement().setProperty("opened", opened);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy