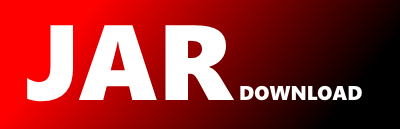
io.thestencil.client.api.ImmutableSiteState Maven / Gradle / Ivy
package io.thestencil.client.api;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableMap;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link StencilComposer.SiteState}.
*
* Use the builder to create immutable instances:
* {@code ImmutableSiteState.builder()}.
*/
@Generated(from = "StencilComposer.SiteState", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableSiteState implements StencilComposer.SiteState {
private final String name;
private final @Nullable String commit;
private final StencilComposer.SiteContentType contentType;
private final ImmutableMap> releases;
private final ImmutableMap> locales;
private final ImmutableMap> pages;
private final ImmutableMap> links;
private final ImmutableMap> articles;
private final ImmutableMap> workflows;
private final ImmutableMap> templates;
private ImmutableSiteState(
String name,
@Nullable String commit,
StencilComposer.SiteContentType contentType,
ImmutableMap> releases,
ImmutableMap> locales,
ImmutableMap> pages,
ImmutableMap> links,
ImmutableMap> articles,
ImmutableMap> workflows,
ImmutableMap> templates) {
this.name = name;
this.commit = commit;
this.contentType = contentType;
this.releases = releases;
this.locales = locales;
this.pages = pages;
this.links = links;
this.articles = articles;
this.workflows = workflows;
this.templates = templates;
}
/**
* @return The value of the {@code name} attribute
*/
@JsonProperty("name")
@Override
public String getName() {
return name;
}
/**
* @return The value of the {@code commit} attribute
*/
@JsonProperty("commit")
@Override
public @Nullable String getCommit() {
return commit;
}
/**
* @return The value of the {@code contentType} attribute
*/
@JsonProperty("contentType")
@Override
public StencilComposer.SiteContentType getContentType() {
return contentType;
}
/**
* @return The value of the {@code releases} attribute
*/
@JsonProperty("releases")
@Override
public ImmutableMap> getReleases() {
return releases;
}
/**
* @return The value of the {@code locales} attribute
*/
@JsonProperty("locales")
@Override
public ImmutableMap> getLocales() {
return locales;
}
/**
* @return The value of the {@code pages} attribute
*/
@JsonProperty("pages")
@Override
public ImmutableMap> getPages() {
return pages;
}
/**
* @return The value of the {@code links} attribute
*/
@JsonProperty("links")
@Override
public ImmutableMap> getLinks() {
return links;
}
/**
* @return The value of the {@code articles} attribute
*/
@JsonProperty("articles")
@Override
public ImmutableMap> getArticles() {
return articles;
}
/**
* @return The value of the {@code workflows} attribute
*/
@JsonProperty("workflows")
@Override
public ImmutableMap> getWorkflows() {
return workflows;
}
/**
* @return The value of the {@code templates} attribute
*/
@JsonProperty("templates")
@Override
public ImmutableMap> getTemplates() {
return templates;
}
/**
* Copy the current immutable object by setting a value for the {@link StencilComposer.SiteState#getName() name} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for name
* @return A modified copy of the {@code this} object
*/
public final ImmutableSiteState withName(String value) {
String newValue = Objects.requireNonNull(value, "name");
if (this.name.equals(newValue)) return this;
return new ImmutableSiteState(
newValue,
this.commit,
this.contentType,
this.releases,
this.locales,
this.pages,
this.links,
this.articles,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by setting a value for the {@link StencilComposer.SiteState#getCommit() commit} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for commit (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableSiteState withCommit(@Nullable String value) {
if (Objects.equals(this.commit, value)) return this;
return new ImmutableSiteState(
this.name,
value,
this.contentType,
this.releases,
this.locales,
this.pages,
this.links,
this.articles,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by setting a value for the {@link StencilComposer.SiteState#getContentType() contentType} attribute.
* A value equality check is used to prevent copying of the same value by returning {@code this}.
* @param value A new value for contentType
* @return A modified copy of the {@code this} object
*/
public final ImmutableSiteState withContentType(StencilComposer.SiteContentType value) {
StencilComposer.SiteContentType newValue = Objects.requireNonNull(value, "contentType");
if (this.contentType == newValue) return this;
return new ImmutableSiteState(
this.name,
this.commit,
newValue,
this.releases,
this.locales,
this.pages,
this.links,
this.articles,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by replacing the {@link StencilComposer.SiteState#getReleases() releases} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the releases map
* @return A modified copy of {@code this} object
*/
public final ImmutableSiteState withReleases(Map> entries) {
if (this.releases == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableSiteState(
this.name,
this.commit,
this.contentType,
newValue,
this.locales,
this.pages,
this.links,
this.articles,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by replacing the {@link StencilComposer.SiteState#getLocales() locales} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the locales map
* @return A modified copy of {@code this} object
*/
public final ImmutableSiteState withLocales(Map> entries) {
if (this.locales == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableSiteState(
this.name,
this.commit,
this.contentType,
this.releases,
newValue,
this.pages,
this.links,
this.articles,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by replacing the {@link StencilComposer.SiteState#getPages() pages} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the pages map
* @return A modified copy of {@code this} object
*/
public final ImmutableSiteState withPages(Map> entries) {
if (this.pages == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableSiteState(
this.name,
this.commit,
this.contentType,
this.releases,
this.locales,
newValue,
this.links,
this.articles,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by replacing the {@link StencilComposer.SiteState#getLinks() links} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the links map
* @return A modified copy of {@code this} object
*/
public final ImmutableSiteState withLinks(Map> entries) {
if (this.links == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableSiteState(
this.name,
this.commit,
this.contentType,
this.releases,
this.locales,
this.pages,
newValue,
this.articles,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by replacing the {@link StencilComposer.SiteState#getArticles() articles} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the articles map
* @return A modified copy of {@code this} object
*/
public final ImmutableSiteState withArticles(Map> entries) {
if (this.articles == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableSiteState(
this.name,
this.commit,
this.contentType,
this.releases,
this.locales,
this.pages,
this.links,
newValue,
this.workflows,
this.templates);
}
/**
* Copy the current immutable object by replacing the {@link StencilComposer.SiteState#getWorkflows() workflows} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the workflows map
* @return A modified copy of {@code this} object
*/
public final ImmutableSiteState withWorkflows(Map> entries) {
if (this.workflows == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableSiteState(
this.name,
this.commit,
this.contentType,
this.releases,
this.locales,
this.pages,
this.links,
this.articles,
newValue,
this.templates);
}
/**
* Copy the current immutable object by replacing the {@link StencilComposer.SiteState#getTemplates() templates} map with the specified map.
* Nulls are not permitted as keys or values.
* A shallow reference equality check is used to prevent copying of the same value by returning {@code this}.
* @param entries The entries to be added to the templates map
* @return A modified copy of {@code this} object
*/
public final ImmutableSiteState withTemplates(Map> entries) {
if (this.templates == entries) return this;
ImmutableMap> newValue = ImmutableMap.copyOf(entries);
return new ImmutableSiteState(
this.name,
this.commit,
this.contentType,
this.releases,
this.locales,
this.pages,
this.links,
this.articles,
this.workflows,
newValue);
}
/**
* This instance is equal to all instances of {@code ImmutableSiteState} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableSiteState
&& equalTo(0, (ImmutableSiteState) another);
}
private boolean equalTo(int synthetic, ImmutableSiteState another) {
return name.equals(another.name)
&& Objects.equals(commit, another.commit)
&& contentType.equals(another.contentType)
&& releases.equals(another.releases)
&& locales.equals(another.locales)
&& pages.equals(another.pages)
&& links.equals(another.links)
&& articles.equals(another.articles)
&& workflows.equals(another.workflows)
&& templates.equals(another.templates);
}
/**
* Computes a hash code from attributes: {@code name}, {@code commit}, {@code contentType}, {@code releases}, {@code locales}, {@code pages}, {@code links}, {@code articles}, {@code workflows}, {@code templates}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + name.hashCode();
h += (h << 5) + Objects.hashCode(commit);
h += (h << 5) + contentType.hashCode();
h += (h << 5) + releases.hashCode();
h += (h << 5) + locales.hashCode();
h += (h << 5) + pages.hashCode();
h += (h << 5) + links.hashCode();
h += (h << 5) + articles.hashCode();
h += (h << 5) + workflows.hashCode();
h += (h << 5) + templates.hashCode();
return h;
}
/**
* Prints the immutable value {@code SiteState} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("SiteState")
.omitNullValues()
.add("name", name)
.add("commit", commit)
.add("contentType", contentType)
.add("releases", releases)
.add("locales", locales)
.add("pages", pages)
.add("links", links)
.add("articles", articles)
.add("workflows", workflows)
.add("templates", templates)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "StencilComposer.SiteState", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements StencilComposer.SiteState {
@Nullable String name;
@Nullable String commit;
@Nullable StencilComposer.SiteContentType contentType;
@Nullable Map> releases = ImmutableMap.of();
@Nullable Map> locales = ImmutableMap.of();
@Nullable Map> pages = ImmutableMap.of();
@Nullable Map> links = ImmutableMap.of();
@Nullable Map> articles = ImmutableMap.of();
@Nullable Map> workflows = ImmutableMap.of();
@Nullable Map> templates = ImmutableMap.of();
@JsonProperty("name")
public void setName(String name) {
this.name = name;
}
@JsonProperty("commit")
public void setCommit(@Nullable String commit) {
this.commit = commit;
}
@JsonProperty("contentType")
public void setContentType(StencilComposer.SiteContentType contentType) {
this.contentType = contentType;
}
@JsonProperty("releases")
public void setReleases(Map> releases) {
this.releases = releases;
}
@JsonProperty("locales")
public void setLocales(Map> locales) {
this.locales = locales;
}
@JsonProperty("pages")
public void setPages(Map> pages) {
this.pages = pages;
}
@JsonProperty("links")
public void setLinks(Map> links) {
this.links = links;
}
@JsonProperty("articles")
public void setArticles(Map> articles) {
this.articles = articles;
}
@JsonProperty("workflows")
public void setWorkflows(Map> workflows) {
this.workflows = workflows;
}
@JsonProperty("templates")
public void setTemplates(Map> templates) {
this.templates = templates;
}
@Override
public String getName() { throw new UnsupportedOperationException(); }
@Override
public String getCommit() { throw new UnsupportedOperationException(); }
@Override
public StencilComposer.SiteContentType getContentType() { throw new UnsupportedOperationException(); }
@Override
public Map> getReleases() { throw new UnsupportedOperationException(); }
@Override
public Map> getLocales() { throw new UnsupportedOperationException(); }
@Override
public Map> getPages() { throw new UnsupportedOperationException(); }
@Override
public Map> getLinks() { throw new UnsupportedOperationException(); }
@Override
public Map> getArticles() { throw new UnsupportedOperationException(); }
@Override
public Map> getWorkflows() { throw new UnsupportedOperationException(); }
@Override
public Map> getTemplates() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableSiteState fromJson(Json json) {
ImmutableSiteState.Builder builder = ImmutableSiteState.builder();
if (json.name != null) {
builder.name(json.name);
}
if (json.commit != null) {
builder.commit(json.commit);
}
if (json.contentType != null) {
builder.contentType(json.contentType);
}
if (json.releases != null) {
builder.putAllReleases(json.releases);
}
if (json.locales != null) {
builder.putAllLocales(json.locales);
}
if (json.pages != null) {
builder.putAllPages(json.pages);
}
if (json.links != null) {
builder.putAllLinks(json.links);
}
if (json.articles != null) {
builder.putAllArticles(json.articles);
}
if (json.workflows != null) {
builder.putAllWorkflows(json.workflows);
}
if (json.templates != null) {
builder.putAllTemplates(json.templates);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link StencilComposer.SiteState} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable SiteState instance
*/
public static ImmutableSiteState copyOf(StencilComposer.SiteState instance) {
if (instance instanceof ImmutableSiteState) {
return (ImmutableSiteState) instance;
}
return ImmutableSiteState.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableSiteState ImmutableSiteState}.
*
* ImmutableSiteState.builder()
* .name(String) // required {@link StencilComposer.SiteState#getName() name}
* .commit(String | null) // nullable {@link StencilComposer.SiteState#getCommit() commit}
* .contentType(io.thestencil.client.api.StencilComposer.SiteContentType) // required {@link StencilComposer.SiteState#getContentType() contentType}
* .putReleases|putAllReleases(String => io.thestencil.client.api.StencilClient.Entity<io.thestencil.client.api.StencilClient.Release>) // {@link StencilComposer.SiteState#getReleases() releases} mappings
* .putLocales|putAllLocales(String => io.thestencil.client.api.StencilClient.Entity<io.thestencil.client.api.StencilClient.Locale>) // {@link StencilComposer.SiteState#getLocales() locales} mappings
* .putPages|putAllPages(String => io.thestencil.client.api.StencilClient.Entity<io.thestencil.client.api.StencilClient.Page>) // {@link StencilComposer.SiteState#getPages() pages} mappings
* .putLinks|putAllLinks(String => io.thestencil.client.api.StencilClient.Entity<io.thestencil.client.api.StencilClient.Link>) // {@link StencilComposer.SiteState#getLinks() links} mappings
* .putArticles|putAllArticles(String => io.thestencil.client.api.StencilClient.Entity<io.thestencil.client.api.StencilClient.Article>) // {@link StencilComposer.SiteState#getArticles() articles} mappings
* .putWorkflows|putAllWorkflows(String => io.thestencil.client.api.StencilClient.Entity<io.thestencil.client.api.StencilClient.Workflow>) // {@link StencilComposer.SiteState#getWorkflows() workflows} mappings
* .putTemplates|putAllTemplates(String => io.thestencil.client.api.StencilClient.Entity<io.thestencil.client.api.StencilClient.Template>) // {@link StencilComposer.SiteState#getTemplates() templates} mappings
* .build();
*
* @return A new ImmutableSiteState builder
*/
public static ImmutableSiteState.Builder builder() {
return new ImmutableSiteState.Builder();
}
/**
* Builds instances of type {@link ImmutableSiteState ImmutableSiteState}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "StencilComposer.SiteState", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_NAME = 0x1L;
private static final long INIT_BIT_CONTENT_TYPE = 0x2L;
private long initBits = 0x3L;
private @Nullable String name;
private @Nullable String commit;
private @Nullable StencilComposer.SiteContentType contentType;
private ImmutableMap.Builder> releases = ImmutableMap.builder();
private ImmutableMap.Builder> locales = ImmutableMap.builder();
private ImmutableMap.Builder> pages = ImmutableMap.builder();
private ImmutableMap.Builder> links = ImmutableMap.builder();
private ImmutableMap.Builder> articles = ImmutableMap.builder();
private ImmutableMap.Builder> workflows = ImmutableMap.builder();
private ImmutableMap.Builder> templates = ImmutableMap.builder();
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code SiteState} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(StencilComposer.SiteState instance) {
Objects.requireNonNull(instance, "instance");
this.name(instance.getName());
@Nullable String commitValue = instance.getCommit();
if (commitValue != null) {
commit(commitValue);
}
this.contentType(instance.getContentType());
putAllReleases(instance.getReleases());
putAllLocales(instance.getLocales());
putAllPages(instance.getPages());
putAllLinks(instance.getLinks());
putAllArticles(instance.getArticles());
putAllWorkflows(instance.getWorkflows());
putAllTemplates(instance.getTemplates());
return this;
}
/**
* Initializes the value for the {@link StencilComposer.SiteState#getName() name} attribute.
* @param name The value for name
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("name")
public final Builder name(String name) {
this.name = Objects.requireNonNull(name, "name");
initBits &= ~INIT_BIT_NAME;
return this;
}
/**
* Initializes the value for the {@link StencilComposer.SiteState#getCommit() commit} attribute.
* @param commit The value for commit (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("commit")
public final Builder commit(@Nullable String commit) {
this.commit = commit;
return this;
}
/**
* Initializes the value for the {@link StencilComposer.SiteState#getContentType() contentType} attribute.
* @param contentType The value for contentType
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("contentType")
public final Builder contentType(StencilComposer.SiteContentType contentType) {
this.contentType = Objects.requireNonNull(contentType, "contentType");
initBits &= ~INIT_BIT_CONTENT_TYPE;
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getReleases() releases} map.
* @param key The key in the releases map
* @param value The associated value in the releases map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putReleases(String key, StencilClient.Entity value) {
this.releases.put(key, value);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getReleases() releases} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putReleases(Map.Entry> entry) {
this.releases.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StencilComposer.SiteState#getReleases() releases} map. Nulls are not permitted
* @param entries The entries that will be added to the releases map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("releases")
public final Builder releases(Map> entries) {
this.releases = ImmutableMap.builder();
return putAllReleases(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StencilComposer.SiteState#getReleases() releases} map. Nulls are not permitted
* @param entries The entries that will be added to the releases map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllReleases(Map> entries) {
this.releases.putAll(entries);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getLocales() locales} map.
* @param key The key in the locales map
* @param value The associated value in the locales map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putLocales(String key, StencilClient.Entity value) {
this.locales.put(key, value);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getLocales() locales} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putLocales(Map.Entry> entry) {
this.locales.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StencilComposer.SiteState#getLocales() locales} map. Nulls are not permitted
* @param entries The entries that will be added to the locales map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("locales")
public final Builder locales(Map> entries) {
this.locales = ImmutableMap.builder();
return putAllLocales(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StencilComposer.SiteState#getLocales() locales} map. Nulls are not permitted
* @param entries The entries that will be added to the locales map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllLocales(Map> entries) {
this.locales.putAll(entries);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getPages() pages} map.
* @param key The key in the pages map
* @param value The associated value in the pages map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putPages(String key, StencilClient.Entity value) {
this.pages.put(key, value);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getPages() pages} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putPages(Map.Entry> entry) {
this.pages.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StencilComposer.SiteState#getPages() pages} map. Nulls are not permitted
* @param entries The entries that will be added to the pages map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("pages")
public final Builder pages(Map> entries) {
this.pages = ImmutableMap.builder();
return putAllPages(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StencilComposer.SiteState#getPages() pages} map. Nulls are not permitted
* @param entries The entries that will be added to the pages map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllPages(Map> entries) {
this.pages.putAll(entries);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getLinks() links} map.
* @param key The key in the links map
* @param value The associated value in the links map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putLinks(String key, StencilClient.Entity value) {
this.links.put(key, value);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getLinks() links} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putLinks(Map.Entry> entry) {
this.links.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StencilComposer.SiteState#getLinks() links} map. Nulls are not permitted
* @param entries The entries that will be added to the links map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("links")
public final Builder links(Map> entries) {
this.links = ImmutableMap.builder();
return putAllLinks(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StencilComposer.SiteState#getLinks() links} map. Nulls are not permitted
* @param entries The entries that will be added to the links map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllLinks(Map> entries) {
this.links.putAll(entries);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getArticles() articles} map.
* @param key The key in the articles map
* @param value The associated value in the articles map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putArticles(String key, StencilClient.Entity value) {
this.articles.put(key, value);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getArticles() articles} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putArticles(Map.Entry> entry) {
this.articles.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StencilComposer.SiteState#getArticles() articles} map. Nulls are not permitted
* @param entries The entries that will be added to the articles map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("articles")
public final Builder articles(Map> entries) {
this.articles = ImmutableMap.builder();
return putAllArticles(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StencilComposer.SiteState#getArticles() articles} map. Nulls are not permitted
* @param entries The entries that will be added to the articles map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllArticles(Map> entries) {
this.articles.putAll(entries);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getWorkflows() workflows} map.
* @param key The key in the workflows map
* @param value The associated value in the workflows map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putWorkflows(String key, StencilClient.Entity value) {
this.workflows.put(key, value);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getWorkflows() workflows} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putWorkflows(Map.Entry> entry) {
this.workflows.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StencilComposer.SiteState#getWorkflows() workflows} map. Nulls are not permitted
* @param entries The entries that will be added to the workflows map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("workflows")
public final Builder workflows(Map> entries) {
this.workflows = ImmutableMap.builder();
return putAllWorkflows(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StencilComposer.SiteState#getWorkflows() workflows} map. Nulls are not permitted
* @param entries The entries that will be added to the workflows map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllWorkflows(Map> entries) {
this.workflows.putAll(entries);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getTemplates() templates} map.
* @param key The key in the templates map
* @param value The associated value in the templates map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putTemplates(String key, StencilClient.Entity value) {
this.templates.put(key, value);
return this;
}
/**
* Put one entry to the {@link StencilComposer.SiteState#getTemplates() templates} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putTemplates(Map.Entry> entry) {
this.templates.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link StencilComposer.SiteState#getTemplates() templates} map. Nulls are not permitted
* @param entries The entries that will be added to the templates map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("templates")
public final Builder templates(Map> entries) {
this.templates = ImmutableMap.builder();
return putAllTemplates(entries);
}
/**
* Put all mappings from the specified map as entries to {@link StencilComposer.SiteState#getTemplates() templates} map. Nulls are not permitted
* @param entries The entries that will be added to the templates map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder putAllTemplates(Map> entries) {
this.templates.putAll(entries);
return this;
}
/**
* Builds a new {@link ImmutableSiteState ImmutableSiteState}.
* @return An immutable instance of SiteState
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableSiteState build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableSiteState(
name,
commit,
contentType,
releases.build(),
locales.build(),
pages.build(),
links.build(),
articles.build(),
workflows.build(),
templates.build());
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_NAME) != 0) attributes.add("name");
if ((initBits & INIT_BIT_CONTENT_TYPE) != 0) attributes.add("contentType");
return "Cannot build SiteState, some of required attributes are not set " + attributes;
}
}
}