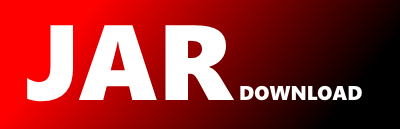
io.resys.thena.docdb.file.tables.ImmutableCommitTableRow Maven / Gradle / Ivy
package io.resys.thena.docdb.file.tables;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.google.common.base.MoreObjects;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import javax.annotation.CheckReturnValue;
import javax.annotation.Nullable;
import javax.annotation.ParametersAreNonnullByDefault;
import javax.annotation.concurrent.Immutable;
import javax.annotation.concurrent.NotThreadSafe;
import org.immutables.value.Generated;
/**
* Immutable implementation of {@link CommitTable.CommitTableRow}.
*
* Use the builder to create immutable instances:
* {@code ImmutableCommitTableRow.builder()}.
*/
@Generated(from = "CommitTable.CommitTableRow", generator = "Immutables")
@SuppressWarnings({"all"})
@ParametersAreNonnullByDefault
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
@Immutable
@CheckReturnValue
public final class ImmutableCommitTableRow
implements CommitTable.CommitTableRow {
private final String id;
private final String datetime;
private final String author;
private final String message;
private final String tree;
private final @Nullable String parent;
private final @Nullable String merge;
private ImmutableCommitTableRow(
String id,
String datetime,
String author,
String message,
String tree,
@Nullable String parent,
@Nullable String merge) {
this.id = id;
this.datetime = datetime;
this.author = author;
this.message = message;
this.tree = tree;
this.parent = parent;
this.merge = merge;
}
/**
* @return The value of the {@code id} attribute
*/
@JsonProperty("id")
@Override
public String getId() {
return id;
}
/**
* @return The value of the {@code datetime} attribute
*/
@JsonProperty("datetime")
@Override
public String getDatetime() {
return datetime;
}
/**
* @return The value of the {@code author} attribute
*/
@JsonProperty("author")
@Override
public String getAuthor() {
return author;
}
/**
* @return The value of the {@code message} attribute
*/
@JsonProperty("message")
@Override
public String getMessage() {
return message;
}
/**
* @return The value of the {@code tree} attribute
*/
@JsonProperty("tree")
@Override
public String getTree() {
return tree;
}
/**
* @return The value of the {@code parent} attribute
*/
@JsonProperty("parent")
@Override
public @Nullable String getParent() {
return parent;
}
/**
* @return The value of the {@code merge} attribute
*/
@JsonProperty("merge")
@Override
public @Nullable String getMerge() {
return merge;
}
/**
* Copy the current immutable object by setting a value for the {@link CommitTable.CommitTableRow#getId() id} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for id
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitTableRow withId(String value) {
String newValue = Objects.requireNonNull(value, "id");
if (this.id.equals(newValue)) return this;
return new ImmutableCommitTableRow(newValue, this.datetime, this.author, this.message, this.tree, this.parent, this.merge);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitTable.CommitTableRow#getDatetime() datetime} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for datetime
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitTableRow withDatetime(String value) {
String newValue = Objects.requireNonNull(value, "datetime");
if (this.datetime.equals(newValue)) return this;
return new ImmutableCommitTableRow(this.id, newValue, this.author, this.message, this.tree, this.parent, this.merge);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitTable.CommitTableRow#getAuthor() author} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for author
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitTableRow withAuthor(String value) {
String newValue = Objects.requireNonNull(value, "author");
if (this.author.equals(newValue)) return this;
return new ImmutableCommitTableRow(this.id, this.datetime, newValue, this.message, this.tree, this.parent, this.merge);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitTable.CommitTableRow#getMessage() message} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for message
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitTableRow withMessage(String value) {
String newValue = Objects.requireNonNull(value, "message");
if (this.message.equals(newValue)) return this;
return new ImmutableCommitTableRow(this.id, this.datetime, this.author, newValue, this.tree, this.parent, this.merge);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitTable.CommitTableRow#getTree() tree} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for tree
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitTableRow withTree(String value) {
String newValue = Objects.requireNonNull(value, "tree");
if (this.tree.equals(newValue)) return this;
return new ImmutableCommitTableRow(this.id, this.datetime, this.author, this.message, newValue, this.parent, this.merge);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitTable.CommitTableRow#getParent() parent} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for parent (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitTableRow withParent(@Nullable String value) {
if (Objects.equals(this.parent, value)) return this;
return new ImmutableCommitTableRow(this.id, this.datetime, this.author, this.message, this.tree, value, this.merge);
}
/**
* Copy the current immutable object by setting a value for the {@link CommitTable.CommitTableRow#getMerge() merge} attribute.
* An equals check used to prevent copying of the same value by returning {@code this}.
* @param value A new value for merge (can be {@code null})
* @return A modified copy of the {@code this} object
*/
public final ImmutableCommitTableRow withMerge(@Nullable String value) {
if (Objects.equals(this.merge, value)) return this;
return new ImmutableCommitTableRow(this.id, this.datetime, this.author, this.message, this.tree, this.parent, value);
}
/**
* This instance is equal to all instances of {@code ImmutableCommitTableRow} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(@Nullable Object another) {
if (this == another) return true;
return another instanceof ImmutableCommitTableRow
&& equalTo(0, (ImmutableCommitTableRow) another);
}
private boolean equalTo(int synthetic, ImmutableCommitTableRow another) {
return id.equals(another.id)
&& datetime.equals(another.datetime)
&& author.equals(another.author)
&& message.equals(another.message)
&& tree.equals(another.tree)
&& Objects.equals(parent, another.parent)
&& Objects.equals(merge, another.merge);
}
/**
* Computes a hash code from attributes: {@code id}, {@code datetime}, {@code author}, {@code message}, {@code tree}, {@code parent}, {@code merge}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + id.hashCode();
h += (h << 5) + datetime.hashCode();
h += (h << 5) + author.hashCode();
h += (h << 5) + message.hashCode();
h += (h << 5) + tree.hashCode();
h += (h << 5) + Objects.hashCode(parent);
h += (h << 5) + Objects.hashCode(merge);
return h;
}
/**
* Prints the immutable value {@code CommitTableRow} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("CommitTableRow")
.omitNullValues()
.add("id", id)
.add("datetime", datetime)
.add("author", author)
.add("message", message)
.add("tree", tree)
.add("parent", parent)
.add("merge", merge)
.toString();
}
/**
* Utility type used to correctly read immutable object from JSON representation.
* @deprecated Do not use this type directly, it exists only for the Jackson-binding infrastructure
*/
@Generated(from = "CommitTable.CommitTableRow", generator = "Immutables")
@Deprecated
@SuppressWarnings("Immutable")
@JsonDeserialize
@JsonAutoDetect(fieldVisibility = JsonAutoDetect.Visibility.NONE)
static final class Json implements CommitTable.CommitTableRow {
@Nullable String id;
@Nullable String datetime;
@Nullable String author;
@Nullable String message;
@Nullable String tree;
@Nullable String parent;
@Nullable String merge;
@JsonProperty("id")
public void setId(String id) {
this.id = id;
}
@JsonProperty("datetime")
public void setDatetime(String datetime) {
this.datetime = datetime;
}
@JsonProperty("author")
public void setAuthor(String author) {
this.author = author;
}
@JsonProperty("message")
public void setMessage(String message) {
this.message = message;
}
@JsonProperty("tree")
public void setTree(String tree) {
this.tree = tree;
}
@JsonProperty("parent")
public void setParent(@Nullable String parent) {
this.parent = parent;
}
@JsonProperty("merge")
public void setMerge(@Nullable String merge) {
this.merge = merge;
}
@Override
public String getId() { throw new UnsupportedOperationException(); }
@Override
public String getDatetime() { throw new UnsupportedOperationException(); }
@Override
public String getAuthor() { throw new UnsupportedOperationException(); }
@Override
public String getMessage() { throw new UnsupportedOperationException(); }
@Override
public String getTree() { throw new UnsupportedOperationException(); }
@Override
public String getParent() { throw new UnsupportedOperationException(); }
@Override
public String getMerge() { throw new UnsupportedOperationException(); }
}
/**
* @param json A JSON-bindable data structure
* @return An immutable value type
* @deprecated Do not use this method directly, it exists only for the Jackson-binding infrastructure
*/
@Deprecated
@JsonCreator(mode = JsonCreator.Mode.DELEGATING)
static ImmutableCommitTableRow fromJson(Json json) {
ImmutableCommitTableRow.Builder builder = ImmutableCommitTableRow.builder();
if (json.id != null) {
builder.id(json.id);
}
if (json.datetime != null) {
builder.datetime(json.datetime);
}
if (json.author != null) {
builder.author(json.author);
}
if (json.message != null) {
builder.message(json.message);
}
if (json.tree != null) {
builder.tree(json.tree);
}
if (json.parent != null) {
builder.parent(json.parent);
}
if (json.merge != null) {
builder.merge(json.merge);
}
return builder.build();
}
/**
* Creates an immutable copy of a {@link CommitTable.CommitTableRow} value.
* Uses accessors to get values to initialize the new immutable instance.
* If an instance is already immutable, it is returned as is.
* @param instance The instance to copy
* @return A copied immutable CommitTableRow instance
*/
public static ImmutableCommitTableRow copyOf(CommitTable.CommitTableRow instance) {
if (instance instanceof ImmutableCommitTableRow) {
return (ImmutableCommitTableRow) instance;
}
return ImmutableCommitTableRow.builder()
.from(instance)
.build();
}
/**
* Creates a builder for {@link ImmutableCommitTableRow ImmutableCommitTableRow}.
*
* ImmutableCommitTableRow.builder()
* .id(String) // required {@link CommitTable.CommitTableRow#getId() id}
* .datetime(String) // required {@link CommitTable.CommitTableRow#getDatetime() datetime}
* .author(String) // required {@link CommitTable.CommitTableRow#getAuthor() author}
* .message(String) // required {@link CommitTable.CommitTableRow#getMessage() message}
* .tree(String) // required {@link CommitTable.CommitTableRow#getTree() tree}
* .parent(String | null) // nullable {@link CommitTable.CommitTableRow#getParent() parent}
* .merge(String | null) // nullable {@link CommitTable.CommitTableRow#getMerge() merge}
* .build();
*
* @return A new ImmutableCommitTableRow builder
*/
public static ImmutableCommitTableRow.Builder builder() {
return new ImmutableCommitTableRow.Builder();
}
/**
* Builds instances of type {@link ImmutableCommitTableRow ImmutableCommitTableRow}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code Builder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CommitTable.CommitTableRow", generator = "Immutables")
@NotThreadSafe
public static final class Builder {
private static final long INIT_BIT_ID = 0x1L;
private static final long INIT_BIT_DATETIME = 0x2L;
private static final long INIT_BIT_AUTHOR = 0x4L;
private static final long INIT_BIT_MESSAGE = 0x8L;
private static final long INIT_BIT_TREE = 0x10L;
private long initBits = 0x1fL;
private @Nullable String id;
private @Nullable String datetime;
private @Nullable String author;
private @Nullable String message;
private @Nullable String tree;
private @Nullable String parent;
private @Nullable String merge;
private Builder() {
}
/**
* Fill a builder with attribute values from the provided {@code CommitTableRow} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final Builder from(CommitTable.CommitTableRow instance) {
Objects.requireNonNull(instance, "instance");
this.id(instance.getId());
this.datetime(instance.getDatetime());
this.author(instance.getAuthor());
this.message(instance.getMessage());
this.tree(instance.getTree());
@Nullable String parentValue = instance.getParent();
if (parentValue != null) {
parent(parentValue);
}
@Nullable String mergeValue = instance.getMerge();
if (mergeValue != null) {
merge(mergeValue);
}
return this;
}
/**
* Initializes the value for the {@link CommitTable.CommitTableRow#getId() id} attribute.
* @param id The value for id
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("id")
public final Builder id(String id) {
this.id = Objects.requireNonNull(id, "id");
initBits &= ~INIT_BIT_ID;
return this;
}
/**
* Initializes the value for the {@link CommitTable.CommitTableRow#getDatetime() datetime} attribute.
* @param datetime The value for datetime
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("datetime")
public final Builder datetime(String datetime) {
this.datetime = Objects.requireNonNull(datetime, "datetime");
initBits &= ~INIT_BIT_DATETIME;
return this;
}
/**
* Initializes the value for the {@link CommitTable.CommitTableRow#getAuthor() author} attribute.
* @param author The value for author
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("author")
public final Builder author(String author) {
this.author = Objects.requireNonNull(author, "author");
initBits &= ~INIT_BIT_AUTHOR;
return this;
}
/**
* Initializes the value for the {@link CommitTable.CommitTableRow#getMessage() message} attribute.
* @param message The value for message
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("message")
public final Builder message(String message) {
this.message = Objects.requireNonNull(message, "message");
initBits &= ~INIT_BIT_MESSAGE;
return this;
}
/**
* Initializes the value for the {@link CommitTable.CommitTableRow#getTree() tree} attribute.
* @param tree The value for tree
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("tree")
public final Builder tree(String tree) {
this.tree = Objects.requireNonNull(tree, "tree");
initBits &= ~INIT_BIT_TREE;
return this;
}
/**
* Initializes the value for the {@link CommitTable.CommitTableRow#getParent() parent} attribute.
* @param parent The value for parent (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("parent")
public final Builder parent(@Nullable String parent) {
this.parent = parent;
return this;
}
/**
* Initializes the value for the {@link CommitTable.CommitTableRow#getMerge() merge} attribute.
* @param merge The value for merge (can be {@code null})
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
@JsonProperty("merge")
public final Builder merge(@Nullable String merge) {
this.merge = merge;
return this;
}
/**
* Builds a new {@link ImmutableCommitTableRow ImmutableCommitTableRow}.
* @return An immutable instance of CommitTableRow
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public ImmutableCommitTableRow build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return new ImmutableCommitTableRow(id, datetime, author, message, tree, parent, merge);
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_ID) != 0) attributes.add("id");
if ((initBits & INIT_BIT_DATETIME) != 0) attributes.add("datetime");
if ((initBits & INIT_BIT_AUTHOR) != 0) attributes.add("author");
if ((initBits & INIT_BIT_MESSAGE) != 0) attributes.add("message");
if ((initBits & INIT_BIT_TREE) != 0) attributes.add("tree");
return "Cannot build CommitTableRow, some of required attributes are not set " + attributes;
}
}
}