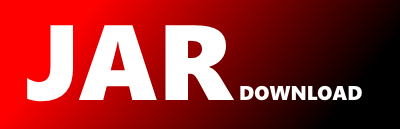
io.dinject.generator.Util Maven / Gradle / Ivy
package io.dinject.generator;
class Util {
static String classOfMethod(String method) {
return packageOf(method);
}
static String shortMethod(String method) {
int p = method.lastIndexOf('.');
if (p > -1) {
p = method.lastIndexOf('.', p - 1);
if (p > -1) {
return method.substring(p + 1);
}
}
return method;
}
static String packageOf(String cls) {
int pos = cls.lastIndexOf('.');
return (pos == -1) ? "" : cls.substring(0, pos);
}
static String unwrapProvider(String maybeProvider) {
if (isProvider(maybeProvider)) {
return extractProviderType(maybeProvider);
} else {
return maybeProvider;
}
}
static String shortName(String fullType) {
int p = fullType.lastIndexOf('.');
if (p == -1) {
return fullType;
} else {
return fullType.substring(p + 1);
}
}
static String extractOptionalType(String rawType) {
return rawType.substring(19, rawType.length() - 1);
}
static boolean isOptional(String rawType) {
return rawType.startsWith("java.util.Optional<");
}
static String extractList(String rawType) {
return rawType.substring(15, rawType.length() - 1);
}
static boolean isList(String rawType) {
return rawType.startsWith("java.util.List<");
}
static boolean isProvider(String rawType) {
return rawType.startsWith("javax.inject.Provider<");
}
static String extractProviderType(String rawType) {
return rawType.substring(22, rawType.length() - 1);
}
/**
* Return the common parent package.
*/
static String commonParent(String currentTop, String aPackage) {
if (aPackage == null) return currentTop;
if (currentTop == null) return aPackage;
if (aPackage.startsWith(currentTop)) {
return currentTop;
}
int next;
do {
next = currentTop.lastIndexOf('.');
if (next > -1) {
currentTop = currentTop.substring(0, next);
if (aPackage.startsWith(currentTop)) {
return currentTop;
}
}
} while (next > -1);
return currentTop;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy