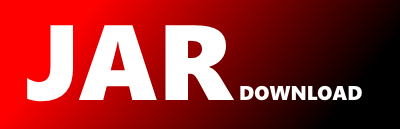
io.divide.client.cache.LocalStorageIBoxDb Maven / Gradle / Ivy
The newest version!
/*
* Copyright (C) 2014 Divide.io
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package io.divide.client.cache;
import com.google.inject.Inject;
import iBoxDB.LocalServer.AutoBox;
import iBoxDB.LocalServer.DB;
import iBoxDB.LocalServer.E.CommitExpection;
import iBoxDB.LocalServer.IFunction;
import io.divide.client.Config;
import io.divide.shared.server.DAO;
import io.divide.shared.transitory.TransientObject;
import io.divide.shared.transitory.query.Clause;
import io.divide.shared.transitory.query.OPERAND;
import io.divide.shared.transitory.query.Query;
import io.divide.shared.util.ObjectUtils;
import java.io.File;
import java.util.*;
import static io.divide.shared.util.ObjectUtils.isArray;
public class LocalStorageIBoxDb implements DAO {
//#from where order by desc, limit <0,-1> #Condition == != < <= > >= & | #IFunction=[F1,F2,F3]
DB db;
AutoBox box;
@Inject
public LocalStorageIBoxDb(Config config){
this(config.fileSavePath + "dbs");
}
public LocalStorageIBoxDb(String path){
System.out.println("iBox: " + path);
File f = new File(path);
f.mkdirs();
db = new DB(path);
// db.getConfig().ensureTable("Wrapper", Wrapper.class, "Key", "Table");
db.ensureTable(Wrapper.class, "Wrapper", "Key", "Table");
box = db.open();
}
@Override
public void save(T1... objects) throws DAOException {
for(TransientObject b : objects){
try {
boolean exists = exists(b);
System.out.println("Exists: " + exists);
if(exists) {
System.out.println("update: "+ b.getObjectKey());
box.update("Wrapper", new Wrapper(b));
} else {
System.out.println("insert: " + b.getObjectKey());
box.insert("Wrapper", new Wrapper(b));
}
} catch (CommitExpection e){
e.printStackTrace();
throw new DAOException(e);
}
}
}
@Override
public void delete(T1... objects) throws DAOException {
String[] keys = new String[objects.length];
for(int x=0;x x = internalGet(objects[0].getObjectType(), keys);
for(Wrapper wr : x){
box.delete("Wrapper", wr);
}
// String[] keys = new String[objects.length];
// for(int x=0;x toDelete = internalGet(table, keys);
//
// box.delete("Wrapper", ObjectUtils.toArray(Wrapper.class, toDelete));
}
@Override
public boolean exists(TransientObject... objects) {
if(objects.length == 0) return false;
boolean found = true;
for(TransientObject o : objects){
Wrapper o1 = iBoxUtils.GetFrist(box.select(Wrapper.class,"from Wrapper where Key==? && Table==?", o.getObjectKey(), o.getObjectType() ));
if(o1 == null) found = false;
}
return found;
}
@Override
public int count(String type) {
return (int) box.selectCount("from Wrapper where Table==?", type);
}
@Override
public List query(Query query) {
Class type = null;
try {
type = (Class) Class.forName(Query.reverseTable(query.getFrom()));
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
String table = query.getFrom();
Map where = query.getWhere();
List